How to Add Comments in Batch Script
-
Add Comments Using Double Colon
::
in Batch Script -
Add Comments Using the
REM
Keyword in Batch Script - Add Multiple-Line Comments in Batch Script
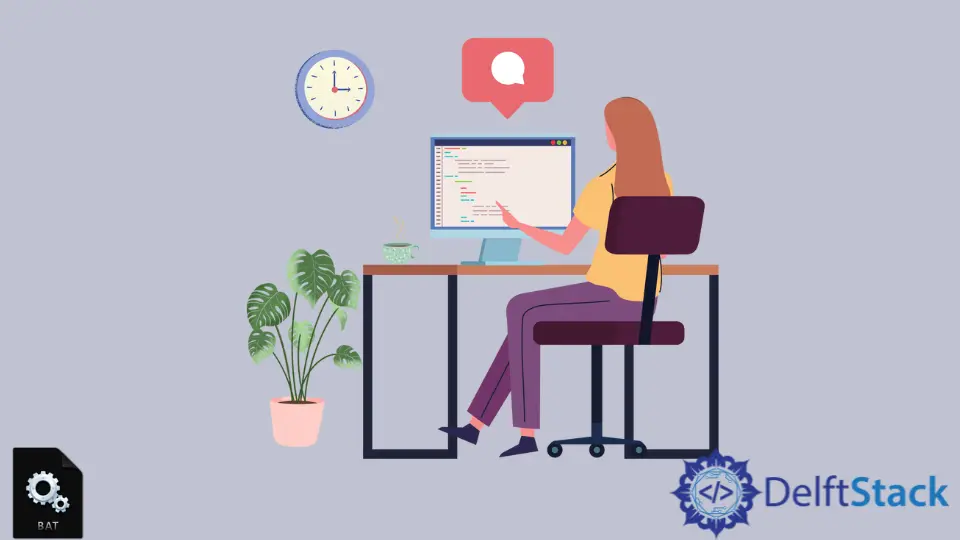
Comments are the most important part as they help include necessary information and explanation to other developers and make it easier to understand the code. It’s an essential element of professional coding.
Comments don’t have any effect on the other part of the. This article will tackle how we can include a single line or multiline comments in our code.
There are two ways to include comments on a Batch Script. We can use the double colon ::
or the keyword REM
.
We will discuss both ways with the necessary examples below.
Add Comments Using Double Colon ::
in Batch Script
To add a comment with the double colon ::
, you can use the following format.
:: THIS IS YOUR COMMENT
We commented on the below code using this method.
@echo off
:: This is your comment
ECHO Commented with ::
Add Comments Using the REM
Keyword in Batch Script
REM
is a keyword used to remark (comment) on Batch Script. To use this keyword, you have to follow the format below.
REM THIS IS YOUR COMMENT
Check out this example for a better understanding,
@echo off
REM This is your comment
ECHO Commented with REM
Some Important Notes on Adding Comments in Batch Script
When comments are not at the beginning of the line, you have to add &
character.
When working with nested parts like IF...ELSE
, loops, etc., you have to use ::
as a normal line; otherwise, it gives an error. You also can use the REM
keyword there.
::
may fail within the SETLOCAL
keyword.
Add Multiple-Line Comments in Batch Script
Sometimes we need to add comments with multiple lines. Here, we will discuss two methods to add multiple-line comments.
Let’s discuss them below. The first method demonstrates adding either ::
or REM
at the beginning of each line of the comment.
@echo off
:: The first line of your comment here.
:: The second line of your comment here.
:: The third line of your comment here.
echo Multiple line comment
Or, like below:
@echo off
REM First line of your comment here.
REM Second line of your comment here.
REM Third line of your comment here.
echo Multiple line comment
The other method is to add the keyword GOTO
. This keyword enables you to skip a specific part of the code.
We can put our comment inside the GOTO
keyword so that the lines become not executable in these lines, like below:
@echo off
goto comment
The first line of your comment is here.
The second line of your comment is here.
The third line of your comment is here.
-
-
-
:comment
echo Multiple line comment...
This example will only execute the last line as it skips the line inside the GOTO
keyword, and the output will be like the following:
Output:
Multiple line comment...
Remember, all methods discussed here are written using Batch Script and will only work in a Windows CMD environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn