How to Download File From URL in Batch Script
-
Use the
curl
Command to Download File From URL in Batch Script -
Some Important Notes About the
curl
Command
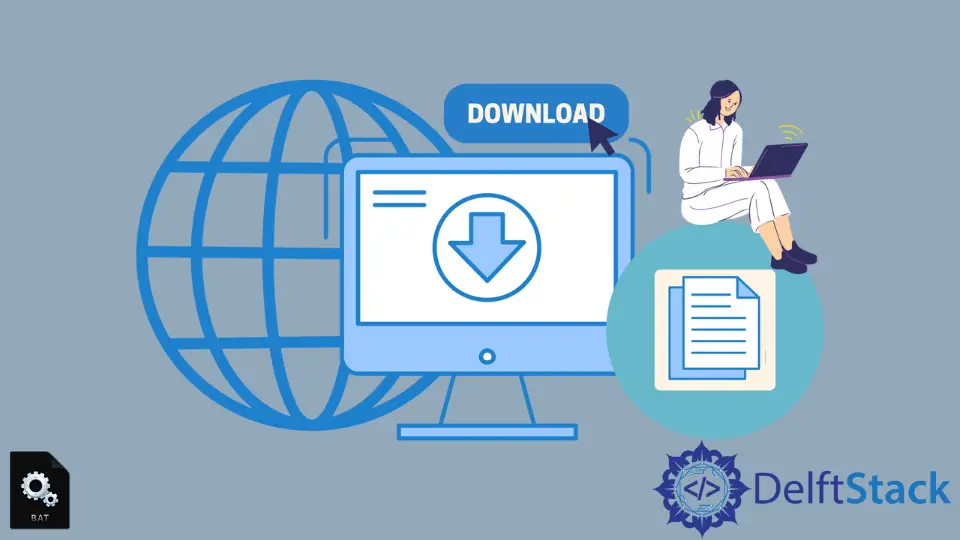
Today we have several download managers to download the necessary files from the internet. Also, there is a download manager integrated into every web browser.
But you can create a batch script that also can do the similar task of downloading files from the internet.
In this article, we will see how we can create a Batch script that can download files from the internet. Also, we will look at some examples and explanations to make the topic easier.
Use the curl
Command to Download File From URL in Batch Script
We can easily download files with a built-in batch command named curl
. In our example below, we will download a file from a website and name it sample.jpg
.
The command for this purpose is shown below:
curl "https://image4.uhdpaper.com/wallpaper/abstract-colorful-digital-art-uhdpaper.com-4K-4.337.jpg" --output sample.jpg
If the code is successfully run, you will get an output like the below. You can see your downloaded file on the directory where the command prompt is open.
So here the download URL is:
https://image4.uhdpaper.com/wallpaper/abstract-colorful-digital-art-uhdpaper.com-4K-4.337.jpg
Output:
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 261 0 261 0 0 261 0 --:--:-- --:--:-- --:--:-- 261
Some Important Notes About the curl
Command
The curl
tool fetches the URL given to the command. When you hit Enter after writing the command with the necessary information, you will see a progress indicator that we already see as an output above.
The general format for this command is:
curl "https://YourLink.com/Yourfile.type" --output Filename.type
If you do not want to see the progress bar, you can include the flag -s
or -silent
. This will make progress invisible.
Now, if you do not include any flag or output file name, then the command will return the source code of the directory.
Check out the below example:
<!doctype html>
<html>
<head>
<title>Example Domain</title>
<meta charset="utf-8" />
<meta http-equiv="Content-type" content="text/html; charset=utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<style type="text/css">
body {
background-color: #f0f0f2;
margin: 0;
padding: 0;
.
.
.
a:link, a:visited {
color: #38488f;
text-decoration: none;
}
@media (max-width: 700px) {
div {
margin: 0 auto;
width: auto;
}
}
</style>
</head>
<body>
<div>
.
.
.
</div>
</body>
</html>
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn