How to Declare an Array in Batch Script
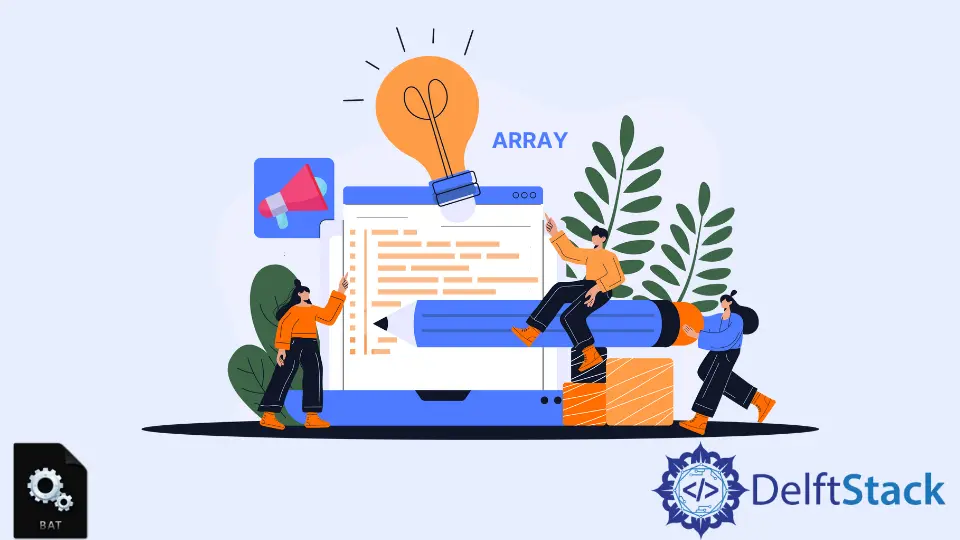
The array is a collection of data that are of the same type. This date can be in various types like integer, float, character, etc.
This article will discuss how to declare and work with an array for various purposes. We will also see necessary examples and explanations to make it easier to understand.
Declare an Array in Batch Script
The general format for declaring an array is shown below:
SET ArrayName=VALUE_1 VALUE_2 ...
Let’s see an example and its part-by-part explanation.
In our below example, we will declare an array with a collection of values. After that, we will show all the elements of the array.
@echo off
SET NumArray=1 2 3 4
(FOR %%x IN (%NumArray%) DO (
ECHO %%x
))
You can notice that we are using a FOR
loop to access all of the components inside the array. Remember, the array index always starts at 0.
After running the example shared above, you will get the below output.
Output:
1
2
3
4
To collect specific indexed elements on the array, you need to put the element’s index inside the third bracket like the following.
%Array_Name[index]%
In our below example, we will collect the second element of an array and show it to the user. Below is the whole code for this example:
@echo off
SET NumArray[0]=1
SET NumArray[1]=2
SET NumArray[2]=3
ECHO The 2nd element of the array is %NumArray[1]%
You can also set or modify an array’s specific indexed element by using the format below:
Array_Name[index]=Value
This is similar to what you can find in our example above. Now when you run the above example, you will get an output like the one below:
The 2nd element of the array is 2
In this last example, we will see how we can modify our array with a specific index. Our example code is shown below.
@echo off
SET NumArray[0]=1
SET NumArray[1]=2
SET NumArray[2]=3
ECHO Currently, the second element of the array is %NumArray[1]%
SET NumArray[1]=12
ECHO The second element of the array after modification is %NumArray[1]%
We have already discussed the necessary parts of the code above. Now, after running this code, we will get the below output:
Currently, the second element of the array is 2
The second element of the array after modification is 12
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn