How to Get the Date and Time in Batch Script
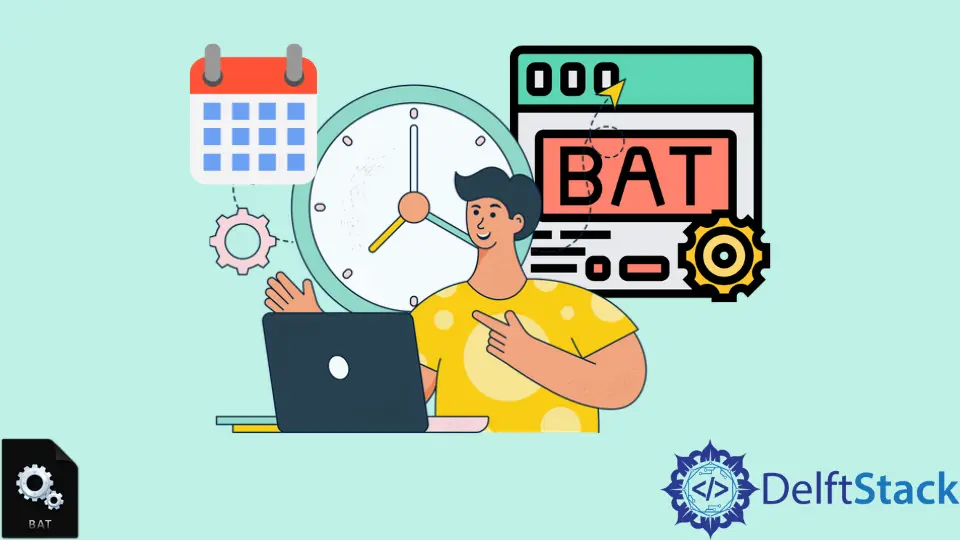
Sometimes we need to work with time and date on our program for various purposes. This article will discuss how we can get or find the time and date in Batch.
We will also see some examples regarding this topic with proper explanations to make it more understandable.
Get the Time in Batch Script
The general format for finding the time in Batch is the following:
ECHO %TIME%
In our below example, we will write a program in Batch that will provide us with the current time. Our code will look like the following:
@echo off
ECHO Current time is - %TIME%
When you run the program, it will show the output below.
Output:
Current time is - 14:07:23.65
Get the Date in Batch Script
The general format for finding the date in Batch is shown below:
ECHO %DATE%
In our below example, we will write a program in Batch that will provide us with the current time. Our code will look like this:
@echo off
ECHO Today is - %DATE%
Now, when you run the program, it will show the below output.
Output:
Today is - Wed 06/01/2022
This code provided the date in a general format: MM/DD/YYYY
. If you want the date in a different format, such as DD/MM/YYYY
or YYYY/MM/DD
, below is a bit advanced example that enables you to customize the date format.
@ECHO off
SET YY=%DATE:~-4%
SET MM=%DATE:~-7,2%
SET DD=%DATE:~-10,2%
ECHO In format YYYY/MM/DD - %YY%-%MM%-%DD%
ECHO In format DD/MM/YYYY - %DD%-%MM%-%YY%
The whole data is provided as a string. To organize the data in our own format, we have to trim the Day, Month, and Year parts from the string, and in our example, we already did that on our first three lines.
First, we trimmed the Year part, then the Month part, and lastly, the Day part. At last, we organize the data in our format.
You will get an output like the following when you run the example.
Output:
In format YYYY/MM/DD - 2022-01-06
In format DD/MM/YYYY - 06-01-2022
Remember, all methods discussed here are written in Batch Script and will only work in Windows CMD.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn