How to Check for Empty Variables in Batch
- Method 1: Using IF Statement
- Method 2: Using the ERRORLEVEL Variable
- Method 3: Using SETLOCAL and ENDLOCAL
- Conclusion
- FAQ
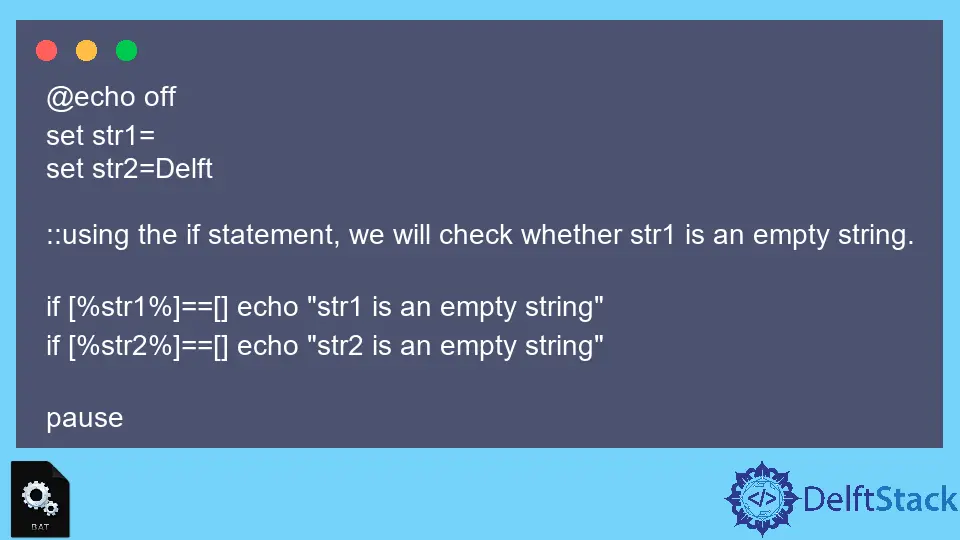
When working with Batch scripting, it’s essential to manage your variables effectively. One common task is checking whether a variable is empty or not. This simple yet crucial operation can prevent errors and ensure your scripts run smoothly.
In this article, we will explore various methods to check for empty variables in Batch. Whether you’re a seasoned developer or just starting, understanding how to handle empty variables can significantly improve your scripting skills. We’ll break down the process into easy-to-follow steps, providing clear examples and explanations. So, let’s dive in and learn how to check for empty variables in Batch!
Method 1: Using IF Statement
The most straightforward way to check if a variable is empty in Batch is by using the IF statement. This method allows you to evaluate the variable directly and execute commands based on its state.
@echo off
set myVar=
if "%myVar%"=="" (
echo The variable is empty.
) else (
echo The variable is not empty.
)
Output:
The variable is empty.
In this example, we first set myVar
to an empty value. The IF statement then checks if myVar
is equal to an empty string. If it is, the script echoes that the variable is empty; otherwise, it confirms that the variable contains a value. This method is effective for quick checks and is easy to implement in your scripts.
Method 2: Using the ERRORLEVEL Variable
Another method to check for empty variables in Batch involves utilizing the ERRORLEVEL variable. This approach can be particularly useful when you want to streamline your scripts and avoid additional commands.
@echo off
set myVar=
if not defined myVar (
echo The variable is empty.
) else (
echo The variable is not empty.
)
Output:
The variable is empty.
In this case, we use the if not defined
command to check if myVar
is defined. If it is not defined, it implies that the variable is empty. This method is concise and efficient, allowing you to quickly determine the state of your variable without needing to compare it to an empty string explicitly. It’s a great option for those who prefer a cleaner syntax in their Batch scripts.
Method 3: Using SETLOCAL and ENDLOCAL
For more complex scripts, you might want to manage variable scope using SETLOCAL and ENDLOCAL. This method ensures that your variable checks are contained within a specific block of code, preventing unintended side effects.
@echo off
setlocal
set myVar=
if "%myVar%"=="" (
echo The variable is empty.
) else (
echo The variable is not empty.
)
endlocal
Output:
The variable is empty.
In this example, we use setlocal
to create a local environment for our variable. The variable myVar
is checked within this local scope, and once we reach endlocal
, any changes made to myVar
will not affect the global environment. This method is particularly useful in larger scripts where variable management is crucial. It helps to avoid conflicts and keeps your code organized.
Conclusion
Checking for empty variables in Batch is a fundamental skill that can enhance the reliability of your scripts. By utilizing methods such as the IF statement, ERRORLEVEL variable, and SETLOCAL/ENDLOCAL, you can effectively manage your variables and avoid potential pitfalls. Remember that understanding how to check for empty variables not only improves your coding efficiency but also contributes to cleaner and more maintainable scripts. With these techniques in your toolkit, you’re better equipped to handle various scripting challenges.
FAQ
- How do I check if a variable is defined in Batch?
You can use the commandif defined myVar
to check if a variable is defined.
-
What happens if I try to use an empty variable in Batch?
Using an empty variable may lead to unexpected results or errors, as Batch scripts rely on variable values for execution. -
Can I check for whitespace in a Batch variable?
Yes, you can use string comparison methods to check for whitespace by trimming the variable before comparison. -
Is it possible to combine multiple checks for variables in Batch?
Absolutely! You can chain multiple IF statements or use logical operators to combine checks. -
What are some common pitfalls when working with variables in Batch?
Common pitfalls include not quoting variable expansions, which can lead to errors, and failing to manage variable scope properly.
John is a Git and PowerShell geek. He uses his expertise in the version control system to help businesses manage their source code. According to him, Shell scripting is the number one choice for automating the management of systems.
LinkedIn