How to Implement Error Handling in Batch Script
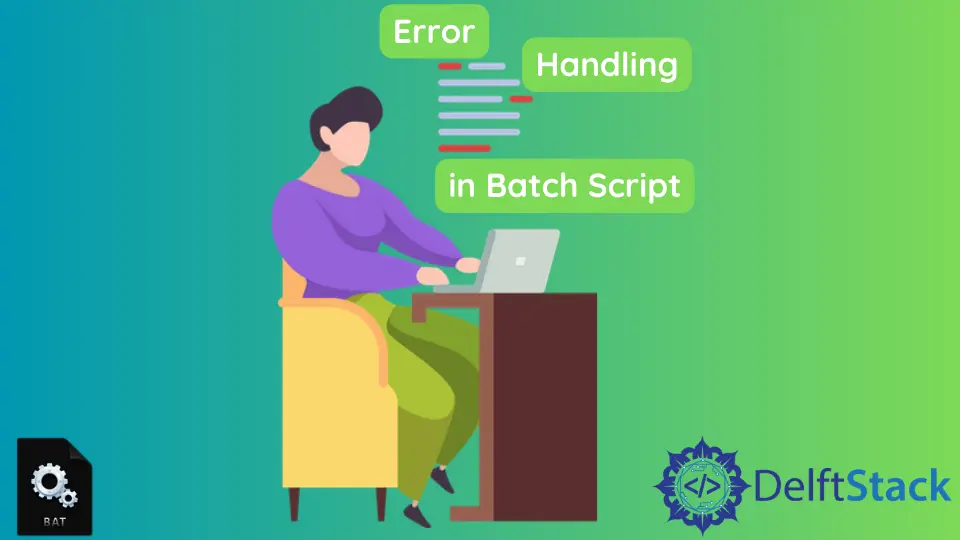
Every scripting and programming language contains an error handler like Java contains try-catch
for error handling. In a Batch script, there is no direct way to do this, but we can create an error handler in the Batch script using a built-in variable of the Batch script name %ERRORLEVEL%
.
This article will show how we can create a Batch script to handle errors and failures. Also, we are going to some examples that make the topic easier.
Error Handling in Batch Script
When a command successfully executes, it always returns an EXIT CODE
that indicates whether the command successfully executed or failed to execute. So, to create an error handling in a Batch file, we can use that EXIT CODE
in our program.
You can follow below general format to create an error handler:
@Echo off
SomeCommand && (
ECHO Message for Success
) || (
ECHO Message for Failure or Error
)
We can also do that by checking the variable named %ERRORLEVEL%
. If the variable contains a value not equal to 0
, then there might be a problem or error when executing the command. To test the %ERRORLEVEL%
variable, you can follow the below example codes:
@ECHO off
Some Command Here !!!
IF %ERRORLEVEL% NEQ 0 (Echo Error found when running the command &Exit /b 1)
You must note that the keyword NEQ
means Not Equal. And the variable %ERRORLEVEL%
will only contain a non-zero value if there is a problem or error in the code.
An Example That Contains Errors
Below, we shared an example. We will run a Batch file named Your_file.bat
from a location.
We intentionally removed that file from the directory. So it’s an error command.
The code for our example will be:
@echo off
ECHO Running a Batch file
CD G:\BATCH\
CALL Your_file.bat
IF errorlevel 1 GOTO ERROR
ECHO The file run successfully.
GOTO EOF
:ERROR
ECHO The file didn't run successfully.
CMD /k
EXIT /b 1
:EOF
Now, as the file doesn’t exist in the directory, it will show an error, and you will get the below output when you run the code shared above.
Output:
Running a Batch file
The system cannot find the path specified.
'Your_file.bat' is not recognized as an internal or external command,
operable program or batch file.
The file didn't run successfully.
An Error-Free Code Example That Runs Successfully
In the example above, we made a mistake on the code intentionally to understand how the code works. If we correct it like below:
@echo off
ECHO Running a Batch file
CALL "G:\BATCH\Yourfile.bat"
IF errorlevel 1 GOTO ERROR
ECHO The file runs successfully.
GOTO EOF
:ERROR
ECHO The file didn't run successfully.
CMD /k
EXIT /b 1
:EOF
Then we will get an output like this:
Running a Batch file
This is from the first file
The file runs successfully.
Remember, all commands we discussed here are only for the Windows Command Prompt or CMD environment.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn