How to Power Arduino Nano With Battery
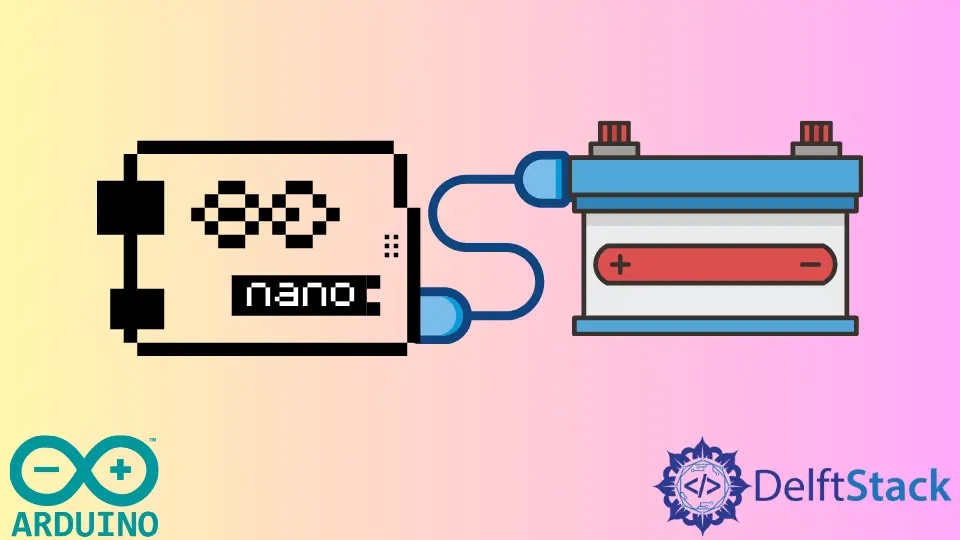
This tutorial will discuss two methods to power an Arduino Nano with a battery. One is using the Vin
pin and the other is using the 5v
pin present on the Arduino board.
Power Arduino Nano Using Vin
Pin
You can power Arduino Nano using a battery of voltage range between 7 to 12 volts. Connect the positive terminal or red wire of the battery with the Vin
pin of the Arduino Nano and the negative terminal or black wire of the battery with the GND
pin of Arduino Nano. Below is a picture of how you can power the board.
If the input voltage is above 12 volts it can damage the Arduino board. This method also has the advantage that you can get 5 volts output from the 5v
pin and use this in your circuit. Check this link for more information.
Power Arduino Nano Using 5v
Pin
If you want to power Arduino Nano with a 5 volts battery, you can use the 5v
pin present on the Arduino board. Connect the positive terminal or red wire of the battery with the 5v
pin of the Arduino Nano and the negative terminal or black wire of the battery with the GND
pin of Arduino Nano. Make sure the input is steady and regulated because the 5v
pin bypasses the voltage regulator and all the other safety measures present on the Arduino board. If input exceeds 5 volts it can damage the Arduino board. If you are powering Arduino using the 5v
pin then make sure no other power supply is connected to the Arduino board.