How to Select Pin for I2c on an Arduino Uno
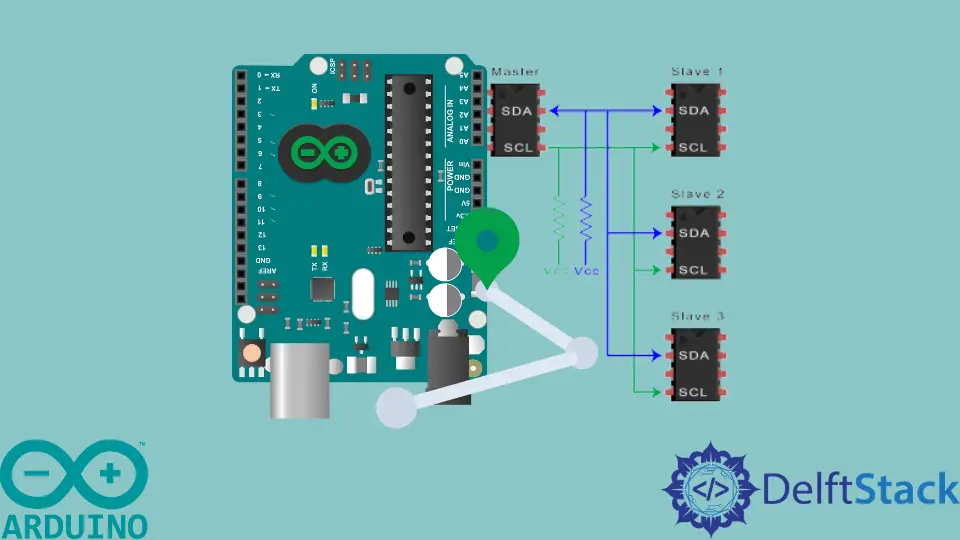
This tutorial introduces an easy step-by-step method to identify the pin layout for the I2C communication bus.
First of all, let’s go through what I2C communication is, shall we?
When you communicate with another individual, you need a mode, like a language that both understand. Consider these two people as Arduino boards, and the language to be the communication bus. However, there is a subtle difference; the relationship with these boards is a Master and Slave.
You can connect as many slave Arduino boards through the bus to the Master board, but only a single master must exist. It is like a CEO, who runs the company, and has many employees. I2C is used for short-distance communication.
As you must know to connect these buses, one must know the exact location of the pin. Usually, in the older Arduino boards, they did not have SDA and SCL connected together.
Now, the newer Uno boards have SDA/SCL connected together to pin A4 and A5 of Arduino UNO. The pin A4 and A5 are also known as PC4 and PC5 of port C.
The SDA pin is an abbreviation for serial data, which is used for communication between the master and slave Arduino Uno. Apart from that, the SCL pin is used to share the clock signal generated for the master and slave.