How to Generate Random Numbers in Arduino
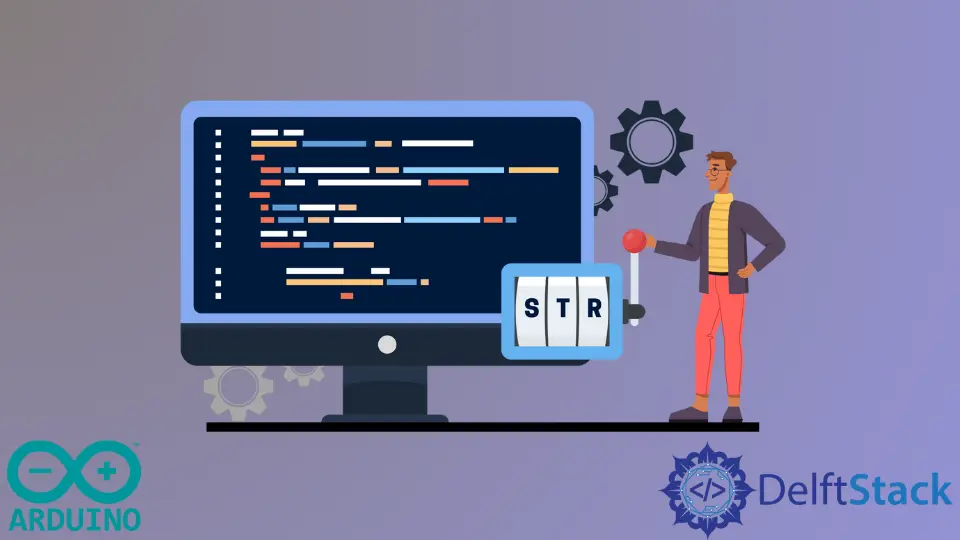
This tutorial will discuss generating random numbers using the random()
function in Arduino.
Use the random()
Function to Generate Random Numbers in Arduino
We use a random number generator to generate a random number between a given range of numbers. For example, we can use it to pick a winner for a giveaway.
We can use the random()
function to generate random numbers in Arduino.
Syntax:
random(maxVlaue);
random(minValue, maxValue);
In the first line of the code, if we only pass the maximum value of the range, the function will use zero as the minimum value. We can also define minimum and maximum ranges using the first and second arguments of the random()
function.
The minimum value for the range is inclusive, while the maximum value is exclusive. For example, if we define the range from 0 to 10, the random number sequence will contain 0 but not 10.
Before using the random()
function, we must initialize it using the randomSeed()
function. We must pass a random number of long
data types inside the randomSeed()
function to initialize the random number generator.
Suppose we want the random the same sequence of numbers as the previous one. We must pass the same number inside the randomSeed()
function.
If we want a different sequence of numbers, we have to use a different number each time we initialize the random number generator. In this case, we can use any of the analogue pins of Arduino.
When no input is connected with an analogue pin, the pin will have a floating value or a random value. We can read that random value using the analogRead()
function.
For example, let’s use the random()
to generate random numbers between 0 to 100.
long MyRnd;
void setup() {
Serial.begin(9600);
randomSeed(analogRead(0));
}
void loop() {
MyRnd = random(100);
Serial.println(MyRnd);
delay(500);
}
Output:
21
17
20
11
46
51
41
71
2
74
The random numbers will keep on generating because we placed the random number generator inside the loop()
function. If we want to generate the random number a specific number of times, we can use a loop inside the setup()
function, which only runs once.
The range values we define inside the random()
function can be 32-bit. If a bigger value is used, the function will not give an error, but the result will differ from what is expected.
If we use the same number like 1
inside the randomSeed()
function and restart the code, the random number sequence will be the same.