How to Convert Integer to String in Arduino
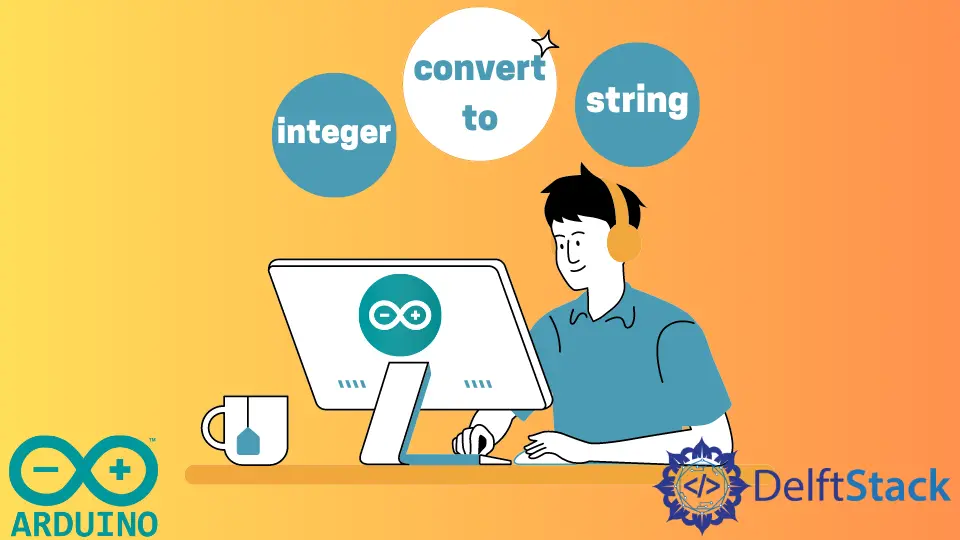
Many novice programmers find it hard to convert integers into strings and vice-versa due to having a rote memory of a particular example in a book. We will create a simple yet practical road map on solving problems like these in the future.
First of all, let us define the necessary terms before proceeding with the code.
An integer is a mathematical word defining a set of all-natural numbers. For instance, a negative number like -2 is an integer, 0 is an integer, although it is neither positive nor negative. Furthermore, all positive natural numbers are also integers. However, be careful a rational, irrational, and imaginary numbers do not come under its umbrella. In a programming language, the int
variable stores integers.
A string in C++, on the other hand, is just a one-dimensional array of characters. For example, the word HU
is a string of two characters. The string variable stores characters. String class also exists, but that is a bit complex at this stage.
Let’s explain it in a simple code.
Serial.begin(9600); // Baud rate for communication, sending bits at a rate of
// 9600 bits/sec.
char a[] = {72, 85}; // define two integer in an array.
Serial.println(a); // Prints the output a.
Before we start explaining, it is necessary to note that this code requires a live Arduino USB attached to the PC for the serial monitor to display the results.
The bit rate is 9600, which is necessary. Increasing it may disrupt the flow, and the output will become erratic. The whole code is an integer, character, and strings in 3 lines. The 72 and 85, two integers, are processed using the char variable and turned into words using the ASCII code. After retrieving this data from the library, the Serial.println
command sends output to the serial monitor. These two characters or alphabets here would make up a string but not necessarily define it.
The monitor will print HU
for display. You can try changing the value and see the result for yourself.
int i = 72; // Assigns an integer value to i
char b[] = {i}; // the integer value has been assign as a variable to b
Serial.println(b);
Now, this example is not your typical one. It is a simple integer to character conversion and, you can further elaborate the code to introduce strings if you want to go complex.
Integer, string and Char Variable explanation
The last example is a bit more sophisticated:
int h = 72; // Assigning integer.
int j = 85;
char c[] = {h,
j}; // Inputting the variable h and j into the character variable c
String(z) = c; // Producing a string.
Serial.println(z);
Let us start with the first line, assigning a simple integer value to h
. So is for j
. Furthermore, the char variable c
then subsets these two variables and converts them from integer to HEX.
Related Article - Arduino String
- Arduino strcmp Function
- Arduino Strcpy Function
- How to Concatenate Strings in Arduino
- How to Parse a String in Arduino
- How to Split String in Arduino
- How to Compare Strings in Arduino