How to Wait for Input in Arduino
-
Use the
Serial.avaiable()
Function to Set the Arduino to Wait for the Input -
Use the
digitalRead()
Function to Set the Arduino to Wait for the Input -
Timeout With
Serial.setTimeout()
- Define a Custom Delimiter
- Wait for a Fixed Length
- Conclusion
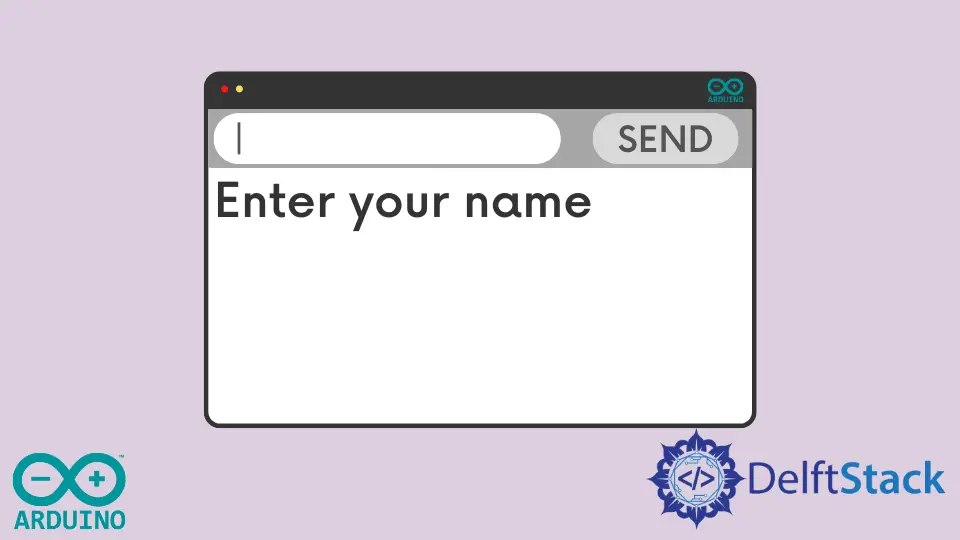
Serial communication is a vital component of Arduino programming, enabling seamless interaction between the microcontroller and external devices. In numerous Arduino projects, the need arises to set the microcontroller to wait for serial input, allowing for controlled data exchange and command reception.
This tutorial will discuss methods to set your Arduino to wait for the input. We will learn a method for serial ports or analog pins and another method for digital pins.
Use the Serial.avaiable()
Function to Set the Arduino to Wait for the Input
The Serial.available()
function is a straightforward way to check if there are incoming bytes in the serial buffer. It returns the number of bytes available for reading.
To read input from a serial port, you can use the Serial.available()
function to wait for the serial input.
This function gets the number of bytes present at the serial port. If there’s no input present, it will return zero.
void setup() { Serial.begin(9600); }
void loop() {
while (Serial.available() == 0) {
}
int mydata = Serial.read();
}
In the above code, if there is no input present at the serial port, then the Arduino will be stuck in a loop and will remain there. If an input arrives at the serial port, the loop will break, and the serial will read data using Serial.read()
and will store it in the variable mydata
.
Use the digitalRead()
Function to Set the Arduino to Wait for the Input
To read input from a digital pin, you can use the digitalRead()
function to wait for the serial input. This function reads the digital value of a digital pin which is either LOW
or HIGH
.
int valPin = 0;
int inputPin = 7;
void setup() { pinMode(inputPin, INPUT); }
void loop() {
while (digitalRead(inputPin) != LOW)
;
{}
valPin = digitalRead(inputPin);
}
In the above code, if there is no input present at the digital pin, then the Arduino will be stuck in a loop and will remain there. If an input arrives at the digital pin, the loop will break, and the serial will read data using digitalRead()
and store it in the variable valPin
.
Timeout With Serial.setTimeout()
Using a timeout with Serial.setTimeout()
allows you to set a maximum time to wait for serial input, providing flexibility in handling delays.
Example:
void setup() {
Serial.begin(9600);
Serial.setTimeout(5000); // Set a timeout of 5000 milliseconds (5 seconds)
}
void loop() {
if (Serial.available()) {
String input = Serial.readStringUntil('\n');
Serial.print("Received: ");
Serial.println(input);
}
}
In this program, Serial.setTimeout(5000)
sets a timeout of 5000 milliseconds. The Serial.readStringUntil('\n')
reads a string from the serial buffer until a newline character is encountered. The received string is then printed to the serial monitor.
Define a Custom Delimiter
Defining a custom delimiter allows you to wait for serial input until a specific character is received, providing control over the termination of input.
Example:
void setup() { Serial.begin(9600); }
void loop() {
if (Serial.find("X")) {
String input = Serial.readStringUntil('X');
Serial.print("Received: ");
Serial.println(input);
}
}
In this program, the Serial.find("X")
searches for the character X
in the serial buffer.
Serial.readStringUntil('X')
reads a string until the custom delimiter X
is encountered. The received string is then printed to the serial monitor.
Wait for a Fixed Length
Waiting for a fixed length of serial input is a reliable method, especially when dealing with known data packet sizes.
Example:
void setup() { Serial.begin(9600); }
void loop() {
const int expectedLength = 4;
if (Serial.available() >= expectedLength) {
char buffer[expectedLength];
Serial.readBytes(buffer, expectedLength);
Serial.print("Received: ");
Serial.println(buffer);
}
}
In this code, the Serial.available() >= expectedLength
checks if the required number of bytes are available. The Serial.readBytes(buffer, expectedLength)
reads a specified number of bytes into the buffer. The received bytes are then printed to the serial monitor.
Conclusion
Implementing waiting mechanisms for serial input in Arduino opens up possibilities for more dynamic and responsive projects.
Whether you choose to use Serial.available()
, set timeouts, define custom delimiters, or wait for a fixed length, these methods provide the flexibility to handle different scenarios. Choose the method that best fits your project’s requirements and enhances the reliability of serial communication in your Arduino-based applications.