How to Toggle Pin in Arduino
- Understanding the Basics of Arduino Digital Pins
- Method 1: Simple Toggle Using a Loop
- Method 2: Toggling with a Button Press
- Method 3: Using Interrupts for Responsive Toggling
- Conclusion
- FAQ
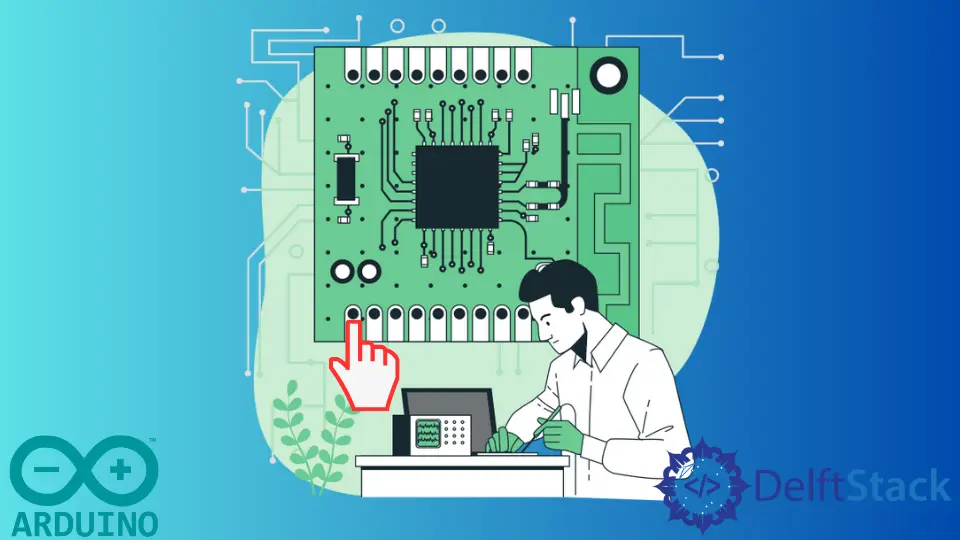
Toggling a digital pin in Arduino is a fundamental skill that every maker and hobbyist should master. Whether you’re controlling an LED, interfacing with sensors, or managing other electronic components, understanding how to switch pins on and off is crucial.
In this tutorial, we will explore how to use the digitalWrite()
function in Arduino to toggle a digital pin effectively. We will break down the process step-by-step, ensuring that both beginners and experienced users can follow along with ease. By the end of this guide, you will have a solid grasp of toggling pins and how to implement this in your own projects.
Understanding the Basics of Arduino Digital Pins
Before diving into the code, it’s essential to understand what digital pins are and how they function in the Arduino environment. Digital pins on an Arduino board can be set to either HIGH or LOW. Setting a pin to HIGH sends a voltage signal, while setting it to LOW turns it off. This binary state allows you to control various components, such as LEDs, motors, and relays. If you are interested in expanding your programming skills, you might consider looking into How to Program Arduino with C++ to enhance your projects further.
In Arduino, the digitalWrite()
function is used to set the state of a digital pin. The syntax is straightforward:
digitalWrite(pin, value);
- pin: The number of the pin you want to control.
- value: Either HIGH (to turn on) or LOW (to turn off).
Now that we’ve covered the basics, let’s look at how to toggle a pin using this function.
Method 1: Simple Toggle Using a Loop
The simplest way to toggle a pin is by using a loop with a delay. This method continuously changes the pin state, creating a blinking effect for an LED or similar component.
Here’s a basic example that toggles pin 13 every second:
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
Output:
Pin 13 turns ON for 1 second, then OFF for 1 second, repeating indefinitely.
In this example, we first set pin 13 as an output in the setup()
function. The loop()
function then alternates the pin state between HIGH and LOW, with a one-second delay in between. This creates a simple blinking LED effect. You can change the pin number and delay duration to customize your project.
Method 2: Toggling with a Button Press
Another practical method for toggling a pin is by using a button. This method is particularly useful for interactive projects where user input is required.
Here’s a simple example that toggles pin 13 when a button connected to pin 2 is pressed:
const int buttonPin = 2;
const int ledPin = 13;
int buttonState = 0;
void setup() {
pinMode(ledPin, OUTPUT);
pinMode(buttonPin, INPUT);
}
void loop() {
buttonState = digitalRead(buttonPin);
if (buttonState == HIGH) {
digitalWrite(ledPin, !digitalRead(ledPin));
delay(200);
}
}
Output:
Pin 13 toggles ON or OFF each time the button is pressed.
In this code, we define two pins: one for the button and one for the LED. The setup()
function initializes both pins, setting the button pin as an input. In the loop()
function, we read the button state. If the button is pressed (HIGH), we toggle the LED state using the digitalWrite()
function. The !digitalRead(ledPin)
statement checks the current state of the LED and switches it to the opposite state. The delay prevents multiple toggles from occurring with a single button press.
Method 3: Using Interrupts for Responsive Toggling
For more advanced projects, using interrupts can provide a responsive way to toggle pins. Interrupts allow your Arduino to react to events, such as a button press, without constantly checking the pin state in the main loop.
Here’s how to implement pin toggling with interrupts:
const int buttonPin = 2;
const int ledPin = 13;
volatile bool ledState = LOW;
void setup() {
pinMode(ledPin, OUTPUT);
pinMode(buttonPin, INPUT);
attachInterrupt(digitalPinToInterrupt(buttonPin), toggleLED, RISING);
}
void loop() {
digitalWrite(ledPin, ledState);
}
void toggleLED() {
ledState = !ledState;
}
Output:
Pin 13 toggles ON or OFF with each button press without delay.
In this example, we use the attachInterrupt()
function to link a button press on pin 2 to the toggleLED()
function. This function changes the ledState
variable, which is then used in the loop()
to set the LED state. This method is efficient because it allows the Arduino to perform other tasks while waiting for the button press, making it ideal for responsive applications.
Conclusion
Toggling a pin in Arduino is a fundamental skill that opens the door to countless projects and applications. Whether you’re creating simple blinking LEDs or more complex interactive systems, understanding how to use the digitalWrite()
function is crucial. In this tutorial, we explored three methods: a simple loop, a button press, and using interrupts. Each method has its use cases, and by mastering them, you can enhance your Arduino projects significantly. Don’t hesitate to experiment with the code and customize it to suit your needs!
FAQ
- What is pin toggling in Arduino?
Pin toggling refers to changing the state of a digital pin from HIGH to LOW or vice versa, often used to control components like LEDs.
-
How can I toggle a pin without using delays?
You can use interrupts to toggle a pin in response to events, allowing for more responsive applications without blocking the main loop. -
Can I toggle multiple pins at once?
Yes, you can toggle multiple pins by callingdigitalWrite()
for each pin within the same loop or function. -
What is the difference between digitalWrite() and digitalRead()?
digitalWrite()
is used to set the state of a pin, whiledigitalRead()
is used to read the current state of a pin. -
How do I debounce a button when toggling a pin?
Debouncing can be achieved by adding a small delay after detecting a button press or using a more sophisticated debouncing algorithm.