Arduino strtok
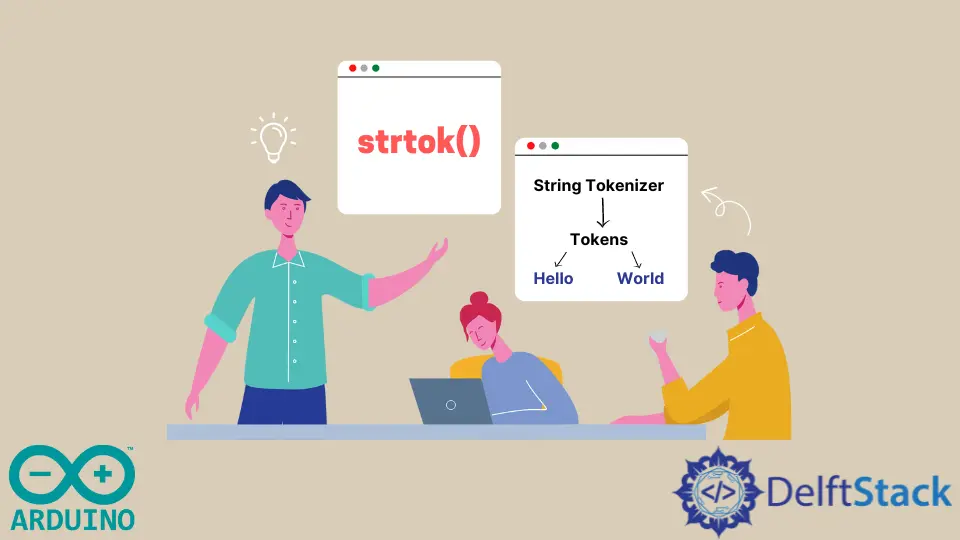
In this tutorial, we will discuss the tokenizing of a string using the strtok()
function present in Arduino.
Tokenize a String Using the strtok()
Function in Arduino
Consider, you have a char
array
of animal names separated by a comma, and you want to separate each name from the char
array
. In this case, you can use the strtok()
function to separate the animal names from the char
array
. The strtok()
function takes two variables as input. The first variable is of type char
in which the animal names are stored, and the second variable is a delimiter for separation like a comma. This function returns a variable of type char
separated based on a delimiter. For example, suppose we need to separate an animal’s first name from a list of animal names.
void setup() {
char AnimalNames[] = "Bog,Cat";
char firstName = strtok(AnimalNames, ",");
}
In the above code, the animal names are stored in the variable AnimalNames
of type char
, and the result will be stored in the variable firstName
of type char
. You can change the variables according to the given variables. Now consider another example, we want to extract all the names of animals present in a list and print them on the serial monitor of Arduino. To do this, we have to use a loop that will iterate the list until it’s empty.
char AnimalNames[] = "dog,cat,donkey,horse";
char *name = NULL;
void setup() {
name = strtok(AnimalNames, ",");
Serial.begin(9600);
while (name != NULL) {
Serial.println(name);
name = strtok(NULL, ",");
}
}
In the above code, the animal names are stored in the variable AnimalNames
of type char
and the result will be stored in the variable name
of type char
. You can change the variables according to the given variables. The names of the animals will be displayed on the serial monitor using the Serial.print()
function.