Arduino strcmp Function
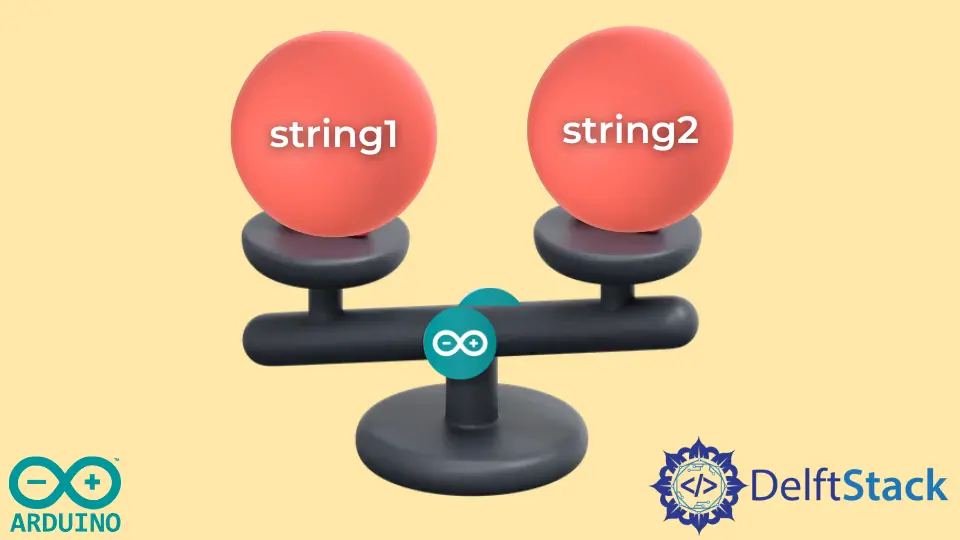
In this tutorial, we will discuss comparing two strings using the strcmp()
function in Arduino.
Arduino strcmp()
Function
The strcmp()
function compares two strings in Arduino. The strcmp()
function compares the ASCII values of characters present in the two strings and then returns three types of output values depending on the ASCII values of the characters.
The characters on a keyboard have unique ASCII values like the ASCII value of character a
is 65
. Below is the basic syntax of the strcmp()
function.
output = strcmp(string1, string2);
The above syntax will return 0
if all the characters present in the two strings are the same, it will return a negative number if the character in the first string which does not match with the character of the second string has a lower ASCII value than the character present in the second string, and it will return a positive number if the non-matching character of the first string has greater ASCII value than the character of the second string.
If the difference of ASCII values of the first two characters is zero, the strcmp()
function will move to the next characters and so on, and when all the characters have been compared, it will also return 0
, which will indicate the two strings are equal.
If the ASCII value of two characters is not equal to zero, the function will stop, returning the difference of ASCII values of the current non-matched characters.
For example, let’s define two same character strings and compare them using the strcmp()
function in Arduino.
See the code below.
int output;
void setup() {
char* string1 = "hello";
char* string2 = "hello";
output = strcmp(string1, string2);
Serial.begin(9600);
Serial.println(output);
}
void loop() {}
Output:
0
The inputs of the strcmp()
function should be a constant character string. In the above code, we used the serial monitor of Arduino to show the output of the strcmp()
function.
The Serial.begin()
function is used to initialize the serial monitor, and the Serial.println()
function prints the given value on the serial monitor window.
We can also use the output of the strcmp()
function in a conditional statement like the if
statement to do a specific task like if the output is equal to zero, we can print on the serial monitor that the two strings are equal.
We can also use other functions of Arduino to compare two strings like the compareTo()
and the equals()
function. Check this link for more details about string comparison in Arduino.