How to Read String in Arduino Serial Port
-
Reading String Using
Serial.readString()
Function in Arduino -
Reading String Using
Serial.readStringUntil()
Function in Arduino
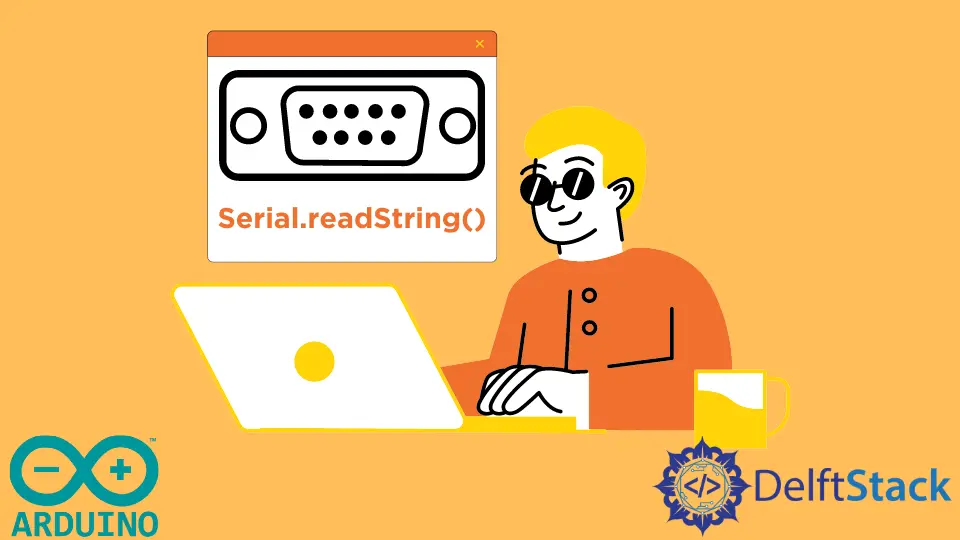
In this tutorial, we will discuss how to read a string from the serial port using the Serial.readString()
function and Serial.readStringUntil()
function in Arduino.
Reading String Using Serial.readString()
Function in Arduino
The Serial.readString()
function reads characters from the serial and stores them into a string. It will terminate if it times out. See setTimeout()
to set the timeout of the Serial.readString()
function. See the below example.
String myString;
void setup() { Serial.begin(9600); }
void loop() {
if (Serial.available()) {
myString = Serial.readString();
Serial.println(myString);
}
}
In the above code, myString
is a variable of type String
to store the string from the serial port. The Serial.available()
function is used to check if data is available on the serial port or not. if data is available at the serial then we will read it into a string and after that, we are printing the received string on the serial monitor.
Reading String Using Serial.readStringUntil()
Function in Arduino
The Serial.readStringUntil()
function reads characters from the serial port until a specific character arrives and stores them into a string. It will terminate if it times out. See setTimeout()
to set the timeout of the Serial.readStringUntil()
function. See the below example.
String myString;
char myChar = 'a';
void setup() { Serial.begin(9600); }
void loop() {
if (Serial.available()) {
myString = Serial.readStringUntil(myChar);
Serial.println(myString);
}
}
In the above code, myString
is a variable of type String
to store the string from the serial port, and myChar
is a variable of type char used to store the terminator character. The Serial.available()
function is used to check if data is available on the serial port or not. If data is available at the serial, then we will read it into a string, and after that, we print the received string on the serial monitor. Note that the Serial.readStringUntil()
only reads a string up to the terminator character.
Related Article - Arduino String
- Arduino strcmp Function
- Arduino Strcpy Function
- How to Concatenate Strings in Arduino
- How to Parse a String in Arduino
- How to Split String in Arduino
- How to Compare Strings in Arduino