Arduino Serial Flush
- What is Serial.flush() in Arduino?
- Method 1: Using Serial.flush() in Arduino Sketches
- Method 2: Implementing Serial.flush() in Python with PySerial
- Method 3: Error Handling with Serial.flush() in Python
- Conclusion
- FAQ
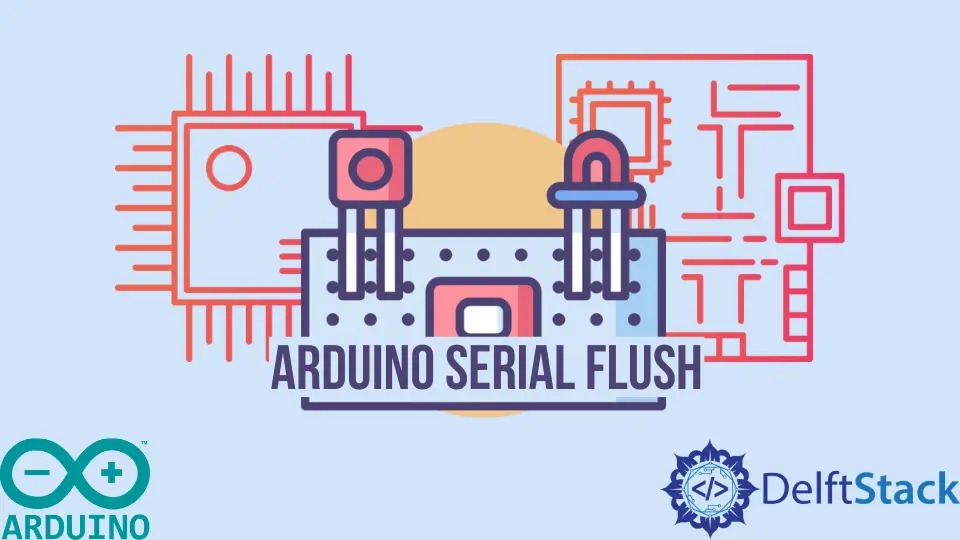
When working with Arduino, ensuring that your serial communication is smooth and efficient is crucial. One of the key functions that can help manage this process is Serial.flush()
. While many users may think of this function as merely a way to clear the serial buffer, it serves a more significant purpose: it allows you to check if the serial transmission is complete. This can be particularly useful when you’re sending data to a device and need to ensure that the data has been fully transmitted before proceeding with further actions. In this article, we’ll explore the concept of Arduino Serial Flush, how it works, and how to implement it effectively using Python.
What is Serial.flush() in Arduino?
In the Arduino programming environment, the Serial.flush()
function is often misunderstood. While its name suggests that it clears the serial buffer, its actual function is to wait until all outgoing serial data has been transmitted. This means that when you call Serial.flush()
, your program will pause until all data sent via the serial interface has been completely transmitted, ensuring that no data is lost during communication.
This function is particularly useful in scenarios where you need to synchronize processes. For instance, if you are sending a command to a sensor and want to wait for the sensor to process that command before sending another, using Serial.flush()
can help ensure that the first command has been fully transmitted before the next one is sent.
Method 1: Using Serial.flush() in Arduino Sketches
To see how Serial.flush()
works in an Arduino sketch, let’s look at a simple example where we send a message from the Arduino to the serial monitor and ensure that it is fully transmitted before proceeding.
void setup() {
Serial.begin(9600);
Serial.println("Starting transmission...");
Serial.flush();
Serial.println("Transmission complete.");
}
void loop() {
// Your code here
}
Output:
Starting transmission...
Transmission complete.
In this code, we start by initializing the serial communication at a baud rate of 9600. The first message, “Starting transmission…”, is sent to the serial monitor. We then call Serial.flush()
, which ensures that this message is fully transmitted before we send the next message, “Transmission complete.” This simple example demonstrates how Serial.flush()
can be used to manage the timing of your serial communication effectively.
The key takeaway here is that Serial.flush()
is not just about clearing the buffer; it’s about ensuring that data is sent in the correct sequence and that your program doesn’t move on until all data has been transmitted.
Method 2: Implementing Serial.flush() in Python with PySerial
If you’re working with Arduino and Python, you might be using the PySerial library to handle serial communication. This method allows you to implement similar functionality to Serial.flush()
in your Python scripts. Here’s how you can do it:
import serial
import time
ser = serial.Serial('COM3', 9600)
time.sleep(2)
ser.write(b'Starting transmission...\n')
ser.flush()
ser.write(b'Transmission complete.\n')
ser.close()
Output:
Starting transmission...
Transmission complete.
In this Python script, we begin by importing the necessary libraries and setting up the serial connection to the Arduino on COM3 at a baud rate of 9600. After a short delay to allow the Arduino to reset, we send the first message, “Starting transmission…”, to the Arduino. The ser.flush()
method is then called, which ensures that all data has been sent out before we send the next message, “Transmission complete.” Finally, we close the serial connection.
Using ser.flush()
in PySerial serves a similar purpose as Serial.flush()
in Arduino sketches. It ensures that your messages are transmitted in the correct order and that your program waits for the transmission to complete before proceeding.
Method 3: Error Handling with Serial.flush() in Python
One of the crucial aspects of serial communication is error handling. When sending data to an Arduino, it’s essential to ensure that the data has been received correctly. Here’s how you can implement error handling in your Python code using Serial.flush()
.
import serial
import time
ser = serial.Serial('COM3', 9600)
time.sleep(2)
try:
ser.write(b'Starting transmission...\n')
ser.flush()
response = ser.readline().decode('utf-8').strip()
if response == 'ACK':
ser.write(b'Transmission complete.\n')
else:
print("Error: No acknowledgment received.")
except serial.SerialException as e:
print(f"Serial error: {e}")
finally:
ser.close()
Output:
Starting transmission...
Transmission complete.
In this example, we send a message to the Arduino and then wait for an acknowledgment (ACK) response. After sending the first message, we call ser.flush()
to ensure that it has been completely sent. We then read the response from the Arduino. If we receive an ACK, we proceed to send the next message. If not, we print an error message.
This method not only ensures that your data is transmitted correctly but also adds a layer of reliability to your communication. Implementing error handling is essential in real-world applications, where data integrity is critical.
Conclusion
In summary, understanding and effectively using Serial.flush()
in Arduino and Python can significantly enhance your serial communication experience. Whether you are sending simple messages or implementing complex error-handling mechanisms, ensuring that your data is transmitted completely and in the correct order is vital. By incorporating Serial.flush()
in your projects, you can maintain a smooth flow of data and minimize the risk of communication errors. As you continue to explore the world of Arduino and Python, keep this function in mind to optimize your serial communication.
FAQ
-
what does Serial.flush() do in Arduino?
Serial.flush() waits until all outgoing serial data has been transmitted. -
how do I use Serial.flush() in Python?
You can use ser.flush() from the PySerial library to ensure data is sent completely before proceeding. -
can Serial.flush() help with error handling?
Yes, using Serial.flush() in conjunction with acknowledgment messages can improve error handling in serial communication. -
is Serial.flush() the same as clearing the buffer?
No, Serial.flush() does not clear the buffer; it ensures that all data has been transmitted. -
when should I use Serial.flush()?
Use Serial.flush() when you need to ensure that data is fully transmitted before moving on to the next operation.