How to Round A Float Number to The Nearest Integer in Arduino
Ammar Ali
Feb 02, 2024
Arduino
Arduino Math
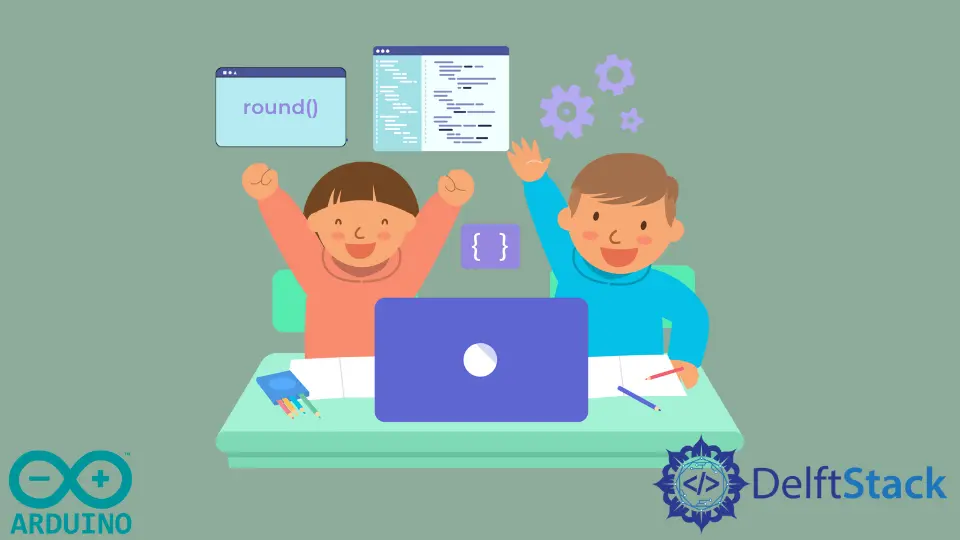
In this tutorial, we will discuss how to round a float
to an int
using the round()
function.
Round a float
to Nearest int
Using the round()
Function
The round()
function rounds a float
number to nearest int
. This function takes a variable of type float
as an input and returns a variable of type int
. If the input variable’s decimal value is less than .5
, the return int
value will be less than the input variable; otherwise, the return int
value will be greater than that of the input variable. For example, see the below code.
void loop() {
float myFloat = 5.4;
int myInt = round(myFloat);
}
In the above code, the float
variable will be converted to 5 since the decimal value is less than .5. See another example.
void loop() {
float myFloat = 5.6;
int myInt = round(myFloat);
}
In the above code, the float
variable will be converted into 6 since the decimal value is greater than .5.
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
Author: Ammar Ali