How to Reset Arduino
- Reset Arduino Using the Reset Button
-
Reset Arduino Using the
Softwarereset
Library -
Reset Arduino Using the Adafruit
SleepyDog
Library
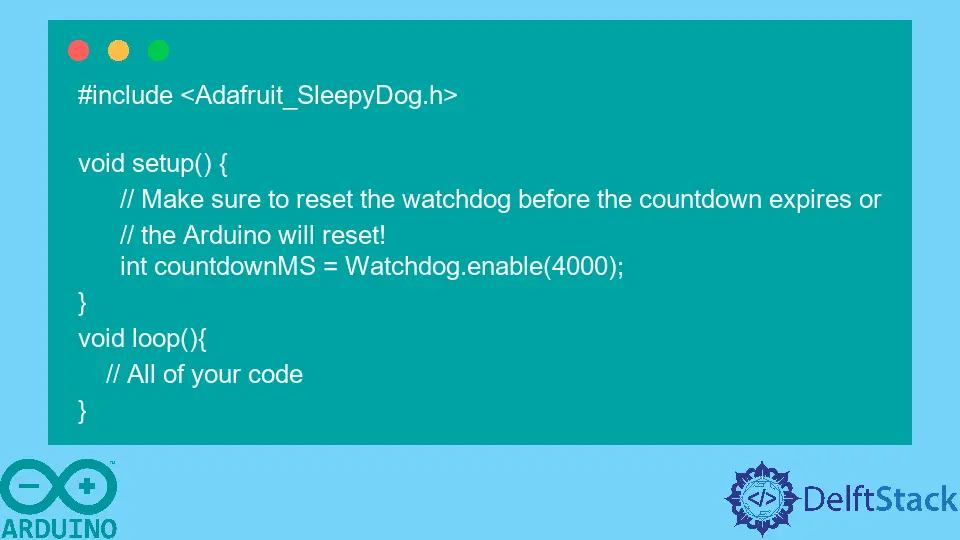
This tutorial will discuss three methods to reset an Arduino. The first method is the reset button present on the Arduino. The second method is the Softwarereset
library, and the third is the Adafruit SleepyDog
library.
Reset Arduino Using the Reset Button
If you are using Linux, there is a bug that stops your Arduino IDE from talking to the Arduino board. As a result, you cannot upload a code in your Arduino, and it will give an error. In this case, you can use this method to reset your Arduino.
First of all, make sure to connect your Arduino directly to your computer without a hub. Using a hub sometimes will give you errors. Now power down the Arduino, press and hold the reset button meanwhile power it up again. This will reset your Arduino, and you can easily upload another code without any errors.
Reset Arduino Using the Softwarereset
Library
If you want to reset your Arduino using a sketch
, you can easily reset it using the Softwarereset
library . This library is compatible with the AVR
architecture so that you can use it with Arduino Uno, Mega, Yun, Nano, and Leonardo boards. To use this library, you need to use the Library Manager
available in the Arduino IDE to install it.
This library has two methods to reset the Arduino. One is the standard method that will reset Arduino using the watchdog
timer. The other method is the simple method which will simply restart the program.
#include <SoftwareReset.h>
void loop() {
// All of your code
softwareReset::standard(); // Reset using the standard method
softwareReset::simple(); // Restart the program
}
Note that any line of code written below the reset code will not be executed. So make sure to use reset after you have completed your code. For more information read the library Documentation.
Reset Arduino Using the Adafruit SleepyDog
Library
The above library works only for five Arduino boards. If your Arduino is not one of them, then you can use this library because it supports almost all the Arduino boards. Use this link to check if your Arduino is compatible with this library.
#include <Adafruit_SleepyDog.h>
void setup() {
// Make sure to reset the watchdog before the countdown expires or
// the Arduino will reset!
int countdownMS = Watchdog.enable(4000);
}
void loop() {
// All of your code
}
In the above code, the Arduino will reset in 4 seconds. You can reset the watchdog using the reset method. For more information read the library documentation.