Arduino printf Function
-
Get Same Output as
prinf()
Usingsprintf()
andSerial.print()
Functions Together in Arduino -
Get Same Output as
prinf()
Using OnlySerial.print()
Function in Arduino
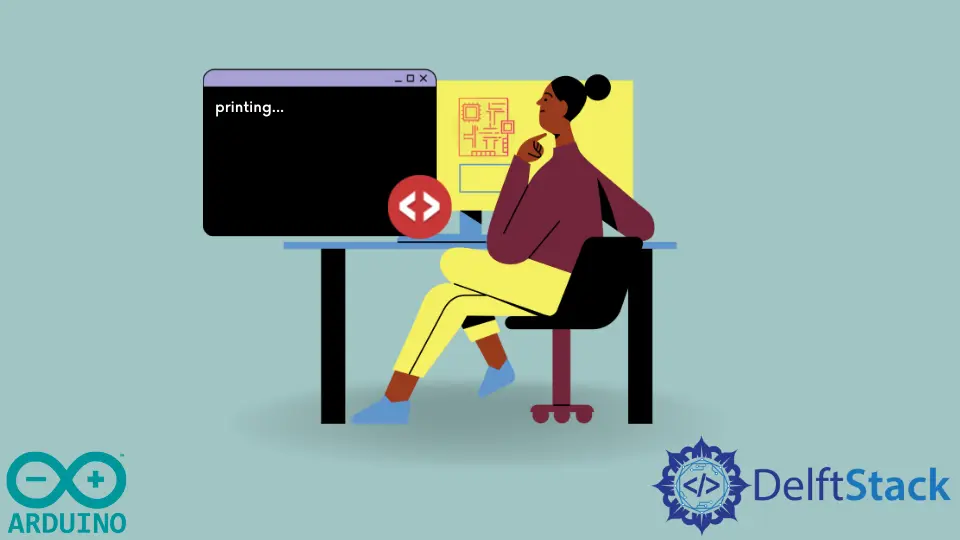
This tutorial will discuss a method to get the same result as the printf()
function in Arduino.
Get Same Output as prinf()
Using sprintf()
and Serial.print()
Functions Together in Arduino
Arduino does not provide the printf()
function. But if you want to get an output like the printf()
function, you can get it using the sprintf()
and Serial.print()
function together. First, you need to use the sprintf()
function to format your output and store it in a char
variable. Then use the Serial.print()
function to output the variable onto the serial monitor.
int time = 0;
char buff[50];
void setup() { Serial.begin(9600); }
void loop() {
sprintf(buff, "the value is %d seconds", time++);
Serial.println(buff);
}
In the above code, the buff
is a variable of type char
to store the formatted output that you want to show on the serial monitor. Note that this method is not encouraged to use because it will take a lot of code space. Instead, you can use the Serial.print()
function two or three times to get the same result as the printf()
function. Also, this method does not work for floating-point numbers. You need to convert them to a string
for floating-point numbers, and then you can use this method.
Get Same Output as prinf()
Using Only Serial.print()
Function in Arduino
If the above method does not work for you, here is a better approach to use. Instead of using the above method, you can use the Serial.print()
function only to get the same result as the printf()
function. But this function will not work for floating-point numbers. For floating-point numbers, you need to convert them to a string and then you can use this function.
int time = 0;
void setup() { Serial.begin(9600); }
void loop() {
Serial.print("the value is ");
Serial.print(time++);
Serial.println(" seconds");
}
This method will also give you the same output as the above method, but it is better and easy to use.