How to Print New Line in Arduino
-
Print New Line Using the
Serial.print()
Function in Arduino -
Print New Line Using the
Serial.println()
Function in Arduino
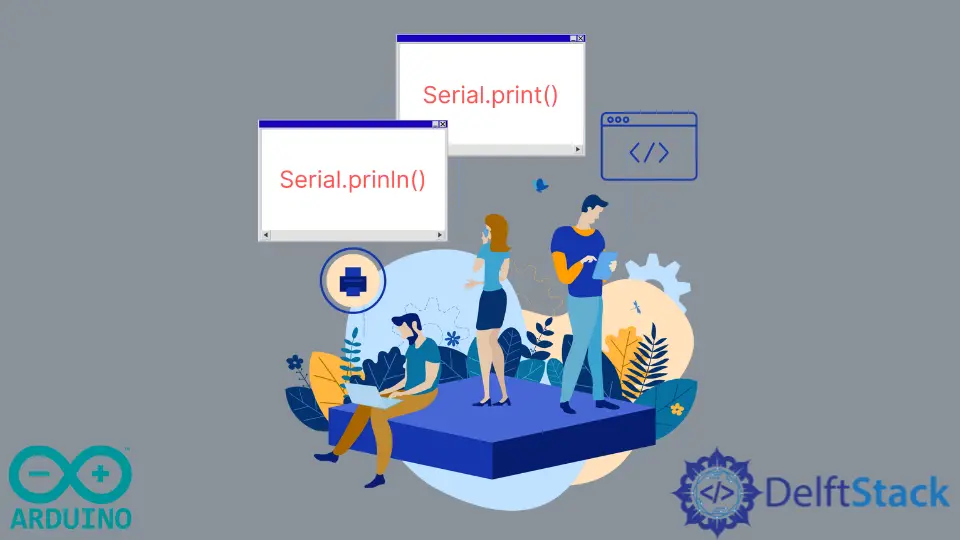
In this tutorial, we will discuss how to print a variable on the serial monitor and then add a new line using the Serial.print()
and Serial.prinln()
function in Arduino.
Print New Line Using the Serial.print()
Function in Arduino
The Serial.print()
function prints a variable on the serial monitor in Arduino. We can also use this function to add a new line on the serial monitor. See the code below.
void setup() {
Serial.begin(9600);
Serial.print('Something');
Serial.print('\n');
}
In the above code, we print Something
on the serial monitor, and after that, we print a new line. But as you can see, we have to use the Serial.print()
function two times, but we can also do that using a single Serial.println()
function.
Print New Line Using the Serial.println()
Function in Arduino
The Serial.println()
function performs the same function as the Serial.print()
function but it adds a new line after printing the variable value.
void setup() {
Serial.begin(9600);
Serial.println('Something');
}
In the above code, we used the Serial.println()
function only one time, and the output is the same as the previous code. So instead of using the Serial.print()
function twice, you can use the Serial.println()
function only one time if you want to add a new line on the serial monitor. If you want to print on the same line, then you can use the Serial.print()
function.