How to Print Char Array in Arduino
-
Define Using
int
and Print Char Array UsingSerial.println()
in Arduino -
Define Using
char
and Print Char Array UsingSerial.println()
in Arduino
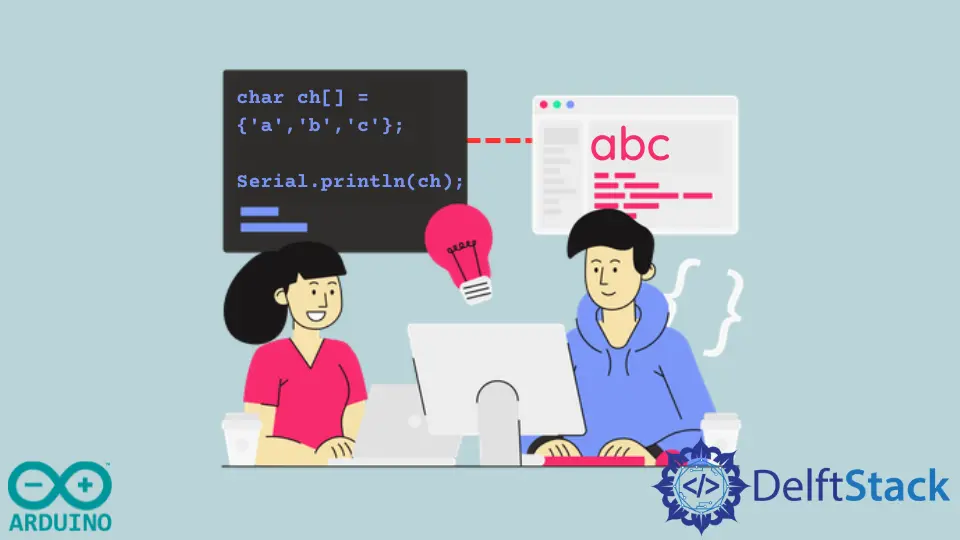
This tutorial will discuss printing a char array using a loop in Arduino.
Define Using int
and Print Char Array Using Serial.println()
in Arduino
In Arduino, if we initialize an array using the int
keyword, we must use a loop to print its elements.
The character array elements are placed on certain indexes, and to print them, we need to get each of them individually. We can do that using a loop in Arduino.
For example, we can use a for
loop that starts from the index 0 and ends at the array’s length, which we can get using the length()
function. Inside the loop, we will get each array element using its index and print it using the Serial.print()
or Serial.println()
function.
The Serial.print()
function prints the value on a single line but the Serial.println()
function prints the value and moves the cursor to the following line.
For example, let’s define a char array and print it on the serial monitor window using a loop in Arduino.
Example:
int ch[] = {'a', 'b', 'c'};
void setup() {
Serial.begin(9600);
for (int i = 0; i < 3; i++) {
char c = char(ch[i]);
Serial.println(c);
}
}
void loop() {}
Output:
a
b
c
Suppose we use int
to define a char array. In that case, the elements will be converted into their ASCII representation, and to print them, we have to convert them back to char using the char()
function; that is why we used the char()
function in the above code.
If we don’t use the char()
function in the above code, the ASCII values of the characters will be printed.
Define Using char
and Print Char Array Using Serial.println()
in Arduino
We can also define a char array using the char
keyword, and we don’t have to use a loop to print it. We also don’t have to use the char()
function because the array is already in the char data type.
For example, let’s define a loop using the char
keyword and print it on the serial monitor.
Example:
char ch[] = {'a', 'b', 'c'};
void setup() {
Serial.begin(9600);
Serial.println(ch);
}
void loop() {}
Output:
abc
If we want to print each element in a separate line, we have to use a loop just like we did in the above example. We can also define a char array as a string.
For example, to define the above char array as a string, we can use the below line of code.
char ch[] = "abc";
In the above examples, we can get any element of the char array using its index. For example, to get the first array element, we will use the below line of code.
char c = ch[0];
We used 0 because the first element is placed at index 0, and the character will be stored in the c
variable. We can also replace the characters present in the array.
We need to get the element we want to replace using its index and replace it with the new one.
For example, to replace the first element of the above array, we will use the below line of code.
ch[0] = 'd';
We can also use a loop to replace more than one array element.