Arduino Nested if Statement
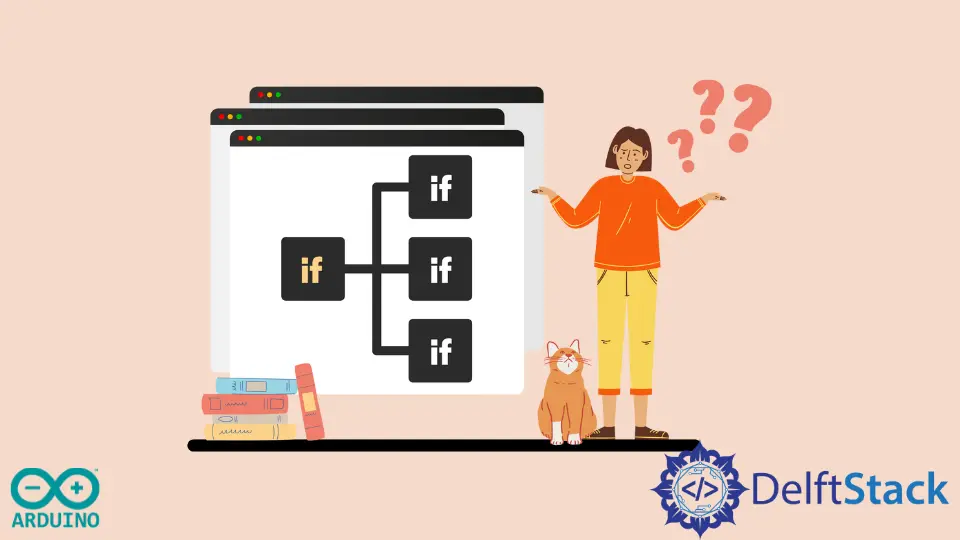
This tutorial will discuss adding an if
statement inside another using the nested if
statement in Arduino.
Arduino Nested if
Statement
The if
statement in Arduino defines conditions like doing a task if certain conditions are fulfilled. If we want to check one and it is true, we want to check another condition,
We can define the second if
statement inside the scope of the first if
statement.
Syntax:
if (condition1) {
if (condition2) {
// do something
} else {
// do something
}
}
In the above code, if the first condition is true, the program will check the second condition, and if it is also true, the code inside the second condition will be executed.
We can also add an if
statement inside the second if
statement in the above code.
For example, let’s create a nested if
statement, check the given number, and print a text according to the conditions.
Code:
long t1 = 11;
long t2 = 600;
long t3 = 600;
void setup() { Serial.begin(9600); }
void loop() {
if (t1 > 10) {
if (t2 < 500) {
Serial.println("Hello");
} else {
Serial.println("World");
}
} else if (t3 > 500) {
Serial.println("Something else");
}
delay(500);
}
Output:
World
The output shows that the text World
is printed on the serial monitor, which means the first condition is true and the second is false. We used the delay()
function to add some delay in the code.
If we want to check multiple conditions before doing a task, we can also use the &&
and ||
operators. The &&
symbol, also known as the AND
operator, is used only if all the given conditions are true when we execute a task.
The ||
symbol, also known as the OR
operator, is used when we want to execute a task even when one condition is true from all the given conditions.
For example, let’s define two conditions to check the range of a number and print a specific text.
See the code below.
long t1 = 15;
void setup() { Serial.begin(9600); }
void loop() {
if (t1 > 10 && t1 < 15) {
Serial.println("Hello");
}
if (t1 > 10 || t1 < 15) {
Serial.println("World");
}
delay(500);
}
Output:
World
In the above code, we have defined two if
statements, and each has two conditions but different operators. One has a &&
operator, and the other has a ||
operator.
As shown in the above output, the second if
statement is executed because one of the two statements is true, and the operator is the OR
operator. We can also define more than two conditions and use both operators inside a single if
statement.
We can also apply the switch
statement to check conditions in Arduino.
Syntax:
switch (variable) {
case A:
// do something when variable equals A
break;
case B:
// do something when variable equals B
break;
default:
// do the default and its optional
break;
}
The variable
contains the value we want to use to define conditions in the above syntax. If the first condition is satisfied, the code below will be executed, and then the program will not check the other cases because of the break
statement.
Note that the switch()
statement can only be used to check the equal condition. If we want to use the less than and greater than conditions, we must use the if-else
statement.
For example, let’s use a number, define three cases, and print a text in each case. If none of the given conditions is true, the default statement will be executed.
Code:
long t1 = 16;
void setup() { Serial.begin(9600); }
void loop() {
switch (t1) {
case 15:
Serial.println("t1 = 15");
break;
case 10:
Serial.println("t1 < 15");
break;
case 20:
Serial.println("t1 > 15");
break;
default:
Serial.println("One of the cases is true");
break;
}
delay(500);
}
Output:
None of the cases is true
As we can see in the output above, none of the cases in the above code is true. That is why the default statement is executed.