Arduino Natural Log
Ammar Ali
Oct 12, 2023
Arduino
Arduino Math
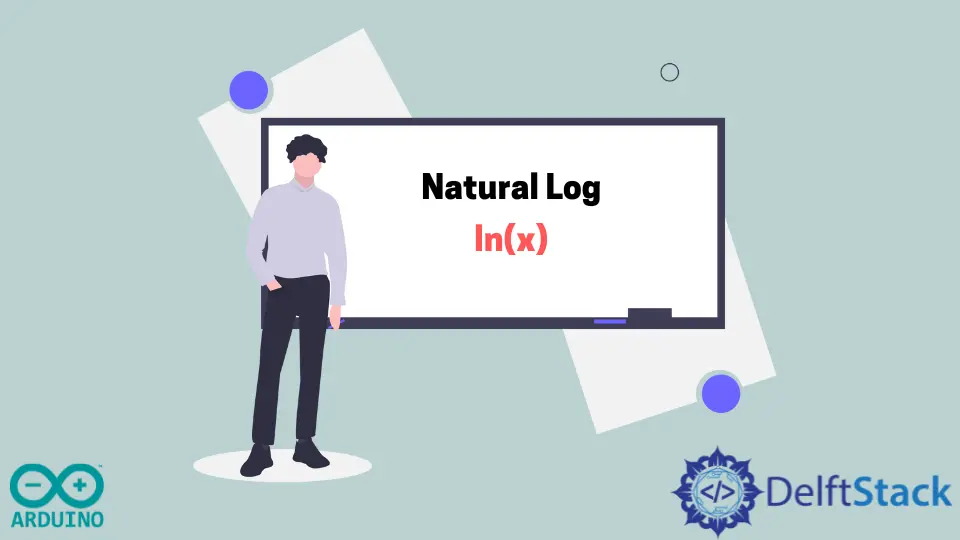
In this tutorial, we will discuss how to take the natural log of a given number using the log()
function in Arduino.
Calculate Natural Log of a Number Using the log()
Function in Arduino
To calculate the natural log of a given number, you can use the log()
function present in Arduino. This function takes an input argument of type double
which contains the given number and returns the natural log of that number. For example, see the below code.
#include <math.h>
void setup() {
double number = 30.5;
double logOfNumber = log(number);
}
In the above code, number
is a variable of type double
to store the given number and logOfNumber
is a variable of type double
to store the result of the natural log. You can change these variables according to your given number. If you want to calculate the log of a number in base 10, you can use the log10()
function.
#include <math.h>
void setup() {
double number = 30.5;
double logOfNumber = log10(number);
}