Arduino millis() Function
-
Use the
millis()
Function to Check the Time Passed in Arduino -
Use the
millis()
Function to Blink an LED in Arduino -
Use the
millis()
Function to Change the Brightness of an LED in Arduino
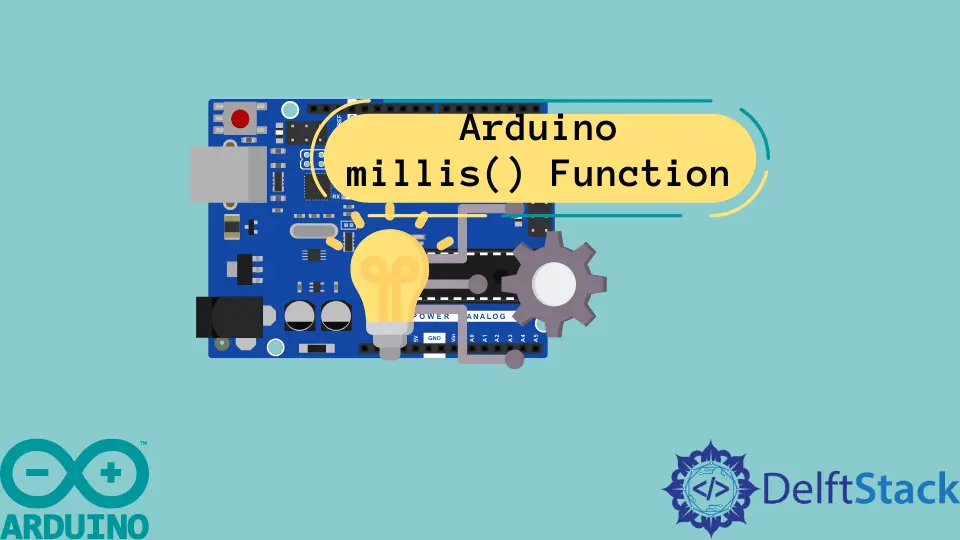
This tutorial will discuss the use of the millis()
function in different applications in Arduino. This tutorial will also discuss some examples better to understand the millis()
function.
Use the millis()
Function to Check the Time Passed in Arduino
The millis()
function returns an unsigned variable of type unsigned long
, which contains the number of milliseconds passed since the Arduino board started running the code. As the returned variable is of type unsigned long
, the number will overflow after 49 days and reset to zero.
unsigned long currentTime;
void setup() { Serial.begin(19200); }
void loop() {
Serial.print("Time Stamp: ");
currentTime = millis();
Serial.println(currentTime);
delay(1000);
}
In the above code, currentTime
is a variable of type unsigned long
to store the time. Check this link to get more information about millis()
function.
Use the millis()
Function to Blink an LED in Arduino
In this example, we will blink an LED using the millis()
function. Consider you have to blink an LED for a specific period, like one second. In this case, you can use the millis()
function to blink the LED for a specific time. If you use the delay()
function to blink the LED, it will also pause your code till the delay time is over. So instead of using the delay()
function, you can use the millis()
function, and it will not pause your code.
unsigned long startTime;
unsigned long currentTime;
const unsigned long period = 1000;
const byte ledPin = 13;
void setup() {
pinMode(ledPin, OUTPUT);
startTime = millis(); // initial start time
}
void loop() {
currentTime = millis();
if (currentTime - startTime >= period) // test whether the period has elapsed
{
digitalWrite(ledPin,
!digitalRead(ledPin)); // if so, change the state of the LED.
startTime = currentTime;
}
}
In this example, the LED will be on and off for precisely one second. You can change the blink period by changing the value of the variable period in the above code.
Use the millis()
Function to Change the Brightness of an LED in Arduino
In this example, we will use the millis()
function to change an LED’s brightness. We will set a period after which the LED’s brightness will be increased. For this purpose, we need to connect an LED with the PWM pin of the Arduino. We can write the brightness to the LED using the analogWrite()
function. We can change the LED brightness in the range of 0 to 255 using the analogWrite
function.
unsigned long startTime;
unsigned long currentTime;
const unsigned long period = 10;
const byte ledPin = 10; // using an LED on a PWM pin.
byte LedBrightness = 0; // initial brightness
byte LedIncrement = 1; // amount to change the brightness
void setup() {
pinMode(ledPin, OUTPUT);
startTime = millis(); // initial start time
}
void loop() {
currentTime = millis();
if (currentTime - startTime >= period) {
analogWrite(ledPin, LedBrightness); // set the brightness
LedBrightness += LedIncrement;
startTime = currentTime;
}
}
In this example, the LED brightness will increment every 10 milliseconds. You can change the increment time by changing the variable period in the above code.