Arduino memcpy and memmove
-
Copy Block of Memory Using the
memcpy()
Function in Arduino -
Copy Block of Memory Using the
memmove()
Function in Arduino
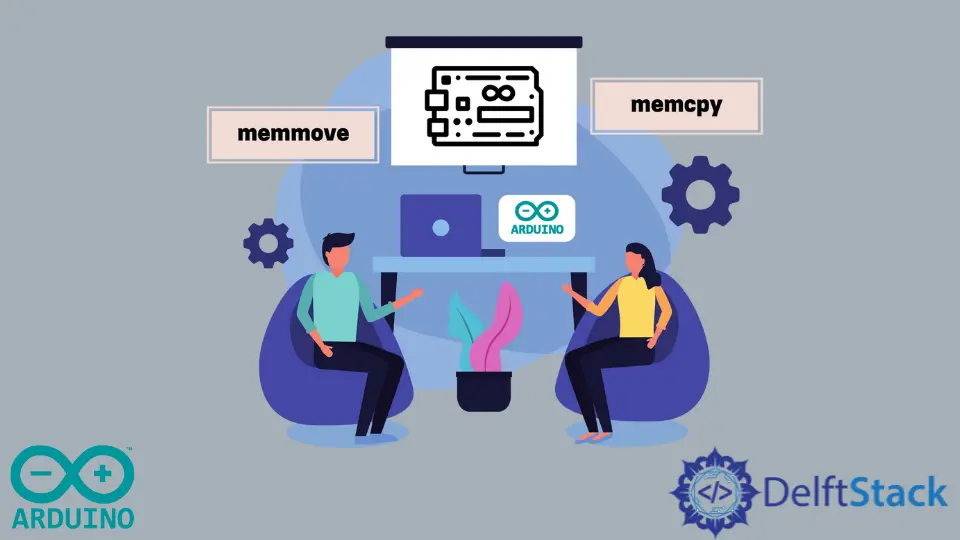
This tutorial will discuss how to copy a block of memory from one variable to another using the memcpy()
and memmove()
functions.
Copy Block of Memory Using the memcpy()
Function in Arduino
If you want to copy one variable’s content to another, you can do that easily using the memcpy()
function. This function takes three input arguments. The first argument is the variable where you want to paste data. The second parameter is the variable from which you want to copy data. The third parameter is the number of bytes
you need to copy from the second variable. For example, consider we want to copy data from one array into another. See the example code below.
int firstArray[10];
int secondArray[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0};
void setup() { memcpy(firstArray, secondArray, sizeof(secondArray)); }
In the above code, we have copied all the data present in the variable secondArray
into the variable firstArray
. Note that you can change the number of bytes you want to copy in the third argument. This function does not check for overflow, and you won’t receive the required output if the two variable’s addresses overlap. So, instead of using this function, you can use the memmove()
function.
Copy Block of Memory Using the memmove()
Function in Arduino
The memcpy()
function created problems when there is an overflow or in the case of the same memory addresses. You can use the memmove()
function instead of the memcpy()
function to solve the above problems. The memmove()
function performs the same task as the memcpy()
function but ignores the overflow. So it will solve many problems. Consider solving the same example as above with the memmove()
function.
int firstArray[10];
int secondArray[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 0};
void setup() { memmove(firstArray, secondArray, sizeof(secondArray)); }
In both of the above examples, the result will be the same, but you can use the memmove()
function to eliminate the chances of error.