Arduino map() Function
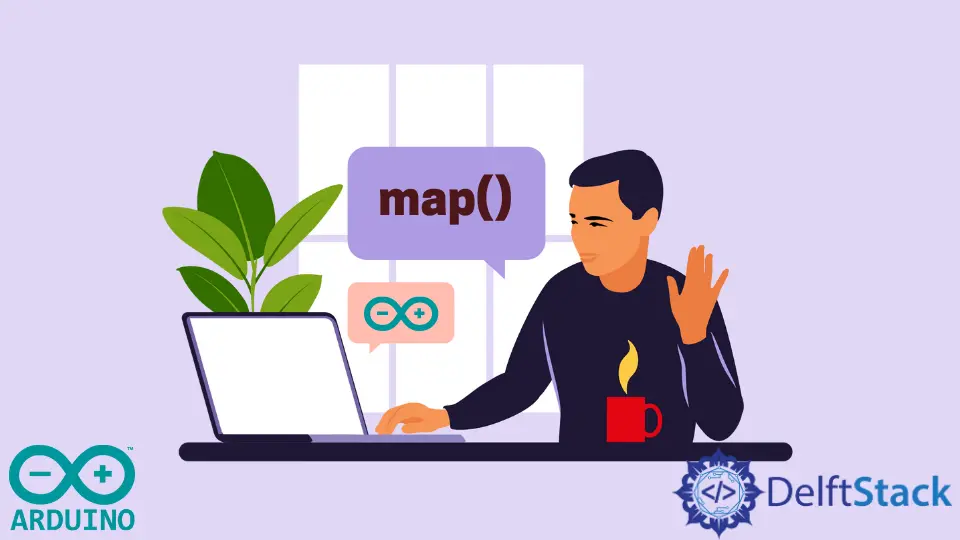
In this tutorial, we will discuss how to use the map()
function in Arduino to map a number from one range to another.
Map a Number From One Range Into Another Using the map()
Function in Arduino
If you want to map a number from one range to another, you can use the map()
function in Arduino. For example, if you want to map a number from a range of 1-1000 to a range of 1-10, you can do that easily using the map()
function.
This function takes five variables of type int
as input parameters.
- The first parameter is the variable whose range you want to convert.
- The second parameter is the original minimum value of the variable’s range.
- The third parameter is the original maximum value of the variable’s range.
- The fourth parameter is the minimum value of the variable’s new range.
- The fifth parameter is the maximum value of the variable’s new range.
For example, let’s map an analog value to 6 bits. Check the code below.
void loop() {
int myValue = analogRead(0);
myValue = map(myValue, 0, 1023, 0, 64);
}
In the above code, we read data from an analog pin 0
using the analogRead()
function. The analogRead()
function return a value whose range is from 0 to 1023. We are converting the range of the value to 0-64, which is the new range. You can change the new range according to the given value. Note that this function only works for integer values. If you want to map fractions, don’t use this function, you have to make your own conversion function.