How to Get Arduino Length of Array using the sizeof() Function
- Arrays in Arduino
-
Using the
sizeof()
Function to Get Arduino Array Length - Getting Array Length
- Practical Example: Finding Array Length
- Conclusion
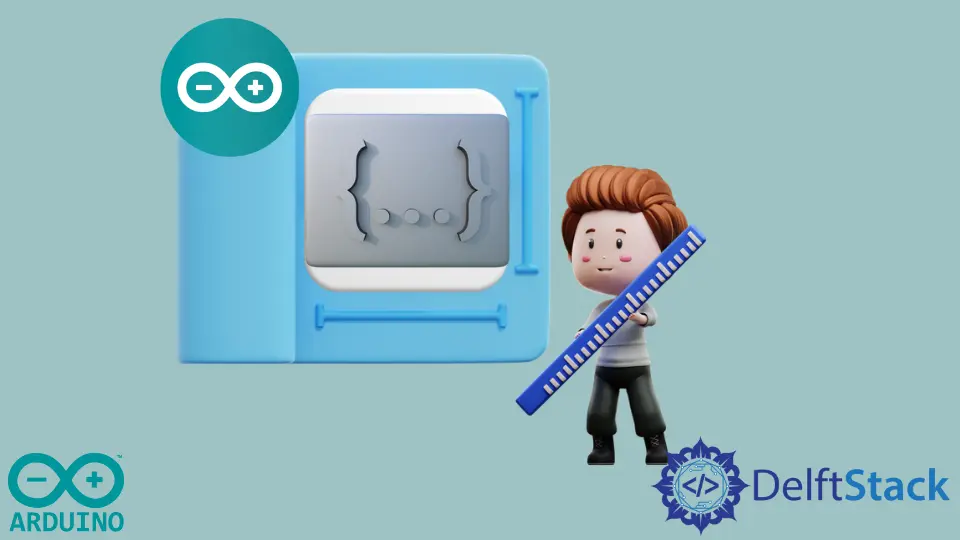
Arrays are fundamental data structures in programming, and when it comes to Arduino, they play a crucial role in storing and manipulating data. Often, you’ll find yourself needing to know the size or length of an array, especially when working on complex projects. In this article, we’ll introduce how to determine the length of an array in Arduino using the sizeof()
function.
Arrays in Arduino
Before delving into array length determination, let’s briefly understand what arrays are in Arduino.
Arrays are collections of variables that hold multiple values of the same data type under a single identifier. Each element in an array is accessed by an index number, which makes it easy to manage and manipulate large sets of data.
In Arduino, arrays are used for various purposes, from storing sensor readings to managing LED patterns and more. Being able to ascertain the length of an array is crucial for iterating through its elements, performing calculations, and ensuring that you don’t access elements beyond the array’s boundaries, which can lead to memory-related issues.
Using the sizeof()
Function to Get Arduino Array Length
The sizeof()
function is a valuable tool in Arduino for determining the size of a variable or an array in bytes. It tells you the number of bytes required to store a particular piece of data. To find the length of an array, you’ll use sizeof()
in a specific way.
Syntax of the sizeof()
Function
Here’s the basic syntax of the sizeof()
function in Arduino:
sizeof(variable)
In this syntax:
variable
: This is the name of the variable or data type for which you want to determine the size.sizeof()
returns the size in bytes as an integer value.
Getting Array Length
To find the length of an array in Arduino, you need to consider the number of bytes the array occupies and then divide it by the number of bytes used by each element in the array. Here’s how you can do it:
int myarray[5] = {19, 10, 8, 17, 9};
int arrayLength = sizeof(myarray) / sizeof(myarray[0]);
In the example above, myarray
is an integer array containing five elements. We determine its length by dividing the size of the array (sizeof(myarray)
) by the size of a single element in the array (sizeof(myarray[0])
). This division gives us the total number of elements in the array.
Be Mindful of Data Types
It’s essential to be mindful of the data type when using sizeof()
. The division should involve the size of a single element that matches the data type of the array. For instance, if you have a float
array, replace sizeof(myarray[0])
with sizeof(float)
.
Practical Example: Finding Array Length
Let’s put this knowledge into practice with a complete example:
void setup() {
Serial.begin(9600);
int myarray[5] = {19, 10, 8, 17, 9};
int arrayLength = sizeof(myarray) / sizeof(myarray[0]);
Serial.print("Array Elements: ");
for (int i = 0; i < arrayLength; i++) {
Serial.print(myarray[i]);
Serial.print(" ");
}
Serial.print("\nArray Length: ");
Serial.println(arrayLength);
}
void loop() {
// Code in the loop, if needed
}
In this example, we have an integer array myarray
with five elements. We calculate its length using the sizeof()
function and then print both the elements and the array’s length to the Serial Monitor.
Conclusion
Understanding how to find the length of an array is fundamental when working with Arduino. The sizeof() function provides a simple and reliable way to determine the size of an array. By dividing the size of the array by the size of a single element with the matching data type, you can confidently ascertain the array’s length.