Arduino LED Resistor
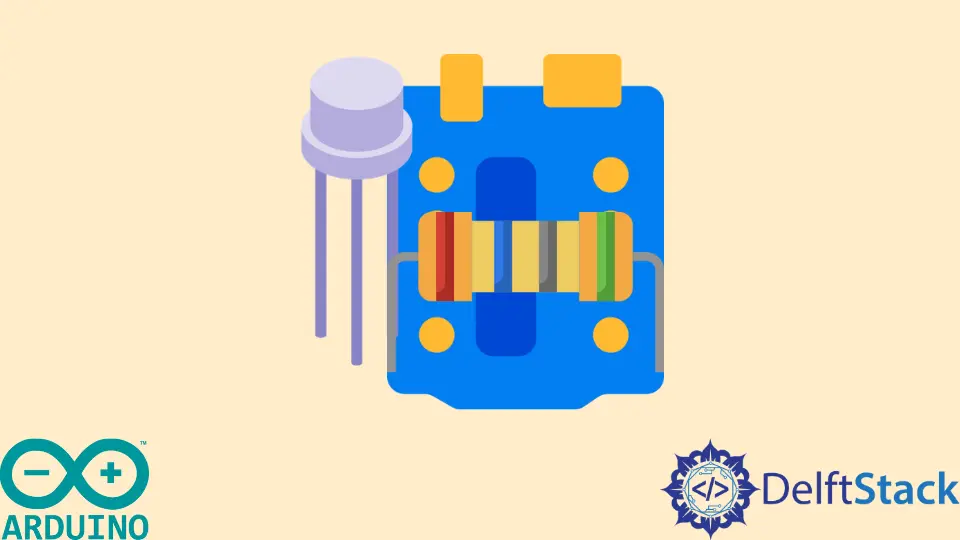
In this tutorial, we will discuss how to use the ohms law to calculate the value of the resistor to protect an LED and Arduino pin.
Use Ohms Law to Calculate the Value of Resistor to Protect an LED and Arduino Pin
The whole purpose of connecting a resistor with an LED is to limit the current flow through the LED to protect the LED and the Arduino. To calculate the resistor value, you have to check the maximum current and voltage drop on the LED from its datasheet.
For example, the standard 5mm red LED has a maximum current of 20mA and a voltage drop of around 1.7 to 2 volts. It means the LED will work properly if we pass around 15mA current through it. So using ohms law R=V/I
, we can calculate the value of the resistor. The voltage we are going to use is the voltage of the Arduino pin, which is 5 volts minus the voltage drop of LED, which is around 1.8 volts. So the voltage will be around 3.2 volts. Dividing the voltage with a 15mA current gives us a resistor value of 213 ohms. That means we can use a resistor above this value which is easily available, like 330 ohms.
Note that before you calculate the value of the resistor for your LED, make sure to check its maximum current and voltage drop and select a resistor value higher than the calculated value to make sure the safety of LED and Arduino.