Arduino if Statement
- Understanding the If Statement in Arduino
- Using Else If for Multiple Conditions
- Nesting If Statements for Complex Logic
- Conclusion
- FAQ
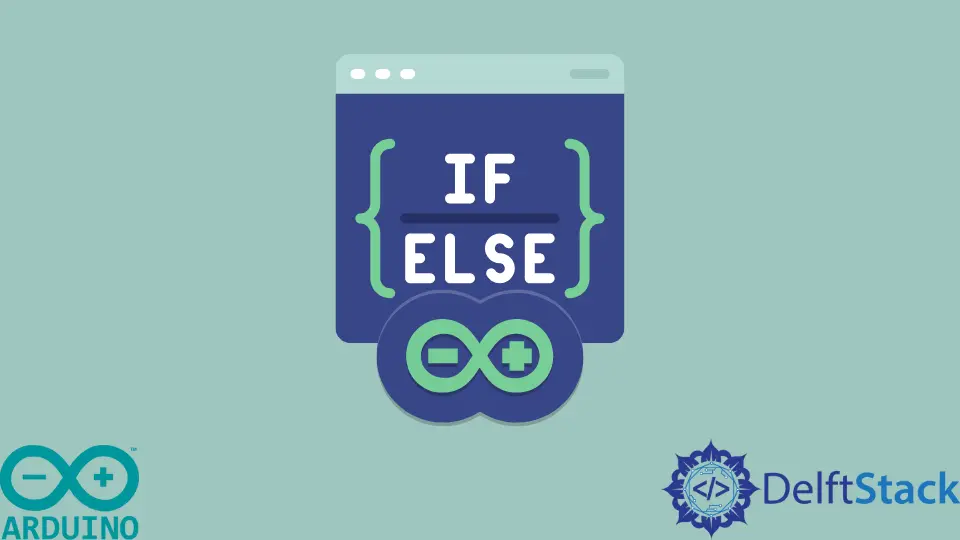
Arduino programming opens up a world of possibilities for hobbyists and professionals alike. One fundamental concept that every Arduino programmer should master is the use of the “if” statement. This powerful tool allows you to check for different conditions and execute specific actions based on those conditions. Whether you’re building a simple LED circuit or a complex robotic system, the if statement is essential for making your projects interactive and responsive.
In this article, we will explore the ins and outs of the Arduino if statement, providing you with clear examples and explanations to enhance your understanding. Let’s dive in!
Understanding the If Statement in Arduino
The if statement in Arduino programming allows you to control the flow of your program based on certain conditions. It evaluates a condition, and if that condition is true, it executes a block of code. This is particularly useful when you want your Arduino to respond to sensor inputs or user interactions.
For example, consider a situation where you want an LED to turn on only when a button is pressed. The if statement can check the state of the button and act accordingly. Here’s a simple code snippet to illustrate this concept:
const int buttonPin = 2;
const int ledPin = 13;
void setup() {
pinMode(buttonPin, INPUT);
pinMode(ledPin, OUTPUT);
}
void loop() {
int buttonState = digitalRead(buttonPin);
if (buttonState == HIGH) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
}
In this code, we declare two pins: one for the button and one for the LED. In the loop function, we read the state of the button. If the button is pressed (buttonState is HIGH), the LED turns on. If not, the LED remains off. This simple example demonstrates how the if statement can control hardware based on user input.
Output:
LED turns on when the button is pressed.
The if statement is versatile and can be used in various scenarios. You can check multiple conditions, nest if statements, or even use else and else if for more complex decision-making processes.
Using Else If for Multiple Conditions
Sometimes, you may want to check for multiple conditions in your Arduino projects. In such cases, the else if statement comes in handy. This allows you to evaluate a second condition if the first one is false.
For instance, let’s say you want to control the brightness of an LED based on the value read from a potentiometer. You can use the following code:
const int potPin = A0;
const int ledPin = 9;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
int potValue = analogRead(potPin);
if (potValue < 341) {
analogWrite(ledPin, 85);
} else if (potValue < 682) {
analogWrite(ledPin, 170);
} else {
analogWrite(ledPin, 255);
}
}
In this example, we read the value from a potentiometer connected to pin A0. Depending on the value, we adjust the brightness of the LED. If the potentiometer value is less than 341, the LED is set to a low brightness. For values between 341 and 682, the brightness is medium, and for values above 682, the LED shines at full brightness.
Output:
LED brightness changes based on potentiometer position.
This code demonstrates how the else if statement can help you create more dynamic and responsive projects. By evaluating multiple conditions, you can make your Arduino projects more interactive and engaging.
Nesting If Statements for Complex Logic
Nesting if statements is another powerful technique you can employ in your Arduino projects. This allows you to check for multiple layers of conditions, making your logic more sophisticated.
Consider a scenario where you want to monitor temperature and humidity using a DHT sensor. You can use nested if statements to control an LED based on both conditions. Here’s how you might implement this:
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
const int ledPin = 13;
void setup() {
pinMode(ledPin, OUTPUT);
dht.begin();
}
void loop() {
float humidity = dht.readHumidity();
float temperature = dht.readTemperature();
if (humidity > 70) {
if (temperature > 30) {
digitalWrite(ledPin, HIGH);
} else {
digitalWrite(ledPin, LOW);
}
} else {
digitalWrite(ledPin, LOW);
}
}
In this code, we first read the humidity and temperature values from the DHT sensor. If the humidity is greater than 70%, the code then checks the temperature. If the temperature is also above 30°C, the LED turns on; otherwise, it remains off. If the humidity is below 70%, the LED will also be off.
Output:
LED turns on only if humidity is high and temperature is above 30°C.
Nesting if statements is a great way to add depth to your logic. It allows you to create intricate conditions that can lead to more advanced functionality in your Arduino projects.
Conclusion
The if statement is an essential building block in Arduino programming. By mastering this concept, you can create interactive and responsive projects that react to various conditions. Whether you’re using simple if statements, exploring else if for multiple conditions, or nesting if statements for complex logic, the possibilities are endless. As you continue your journey with Arduino, remember that the if statement is your ally in bringing your ideas to life. So, start experimenting and let your creativity flow!
FAQ
-
What is an if statement in Arduino?
An if statement in Arduino is a programming construct that allows you to execute a block of code based on whether a specified condition is true. -
Can I use multiple conditions with if statements?
Yes, you can use else if to check multiple conditions sequentially, allowing for more complex decision-making. -
What is the purpose of nesting if statements?
Nesting if statements allows you to evaluate additional conditions within an existing if statement, enabling more complex logic in your programs. -
How do I read sensor values in an if statement?
You can read sensor values using functions like analogRead or digitalRead and then use those values in your if statements to control outputs. -
Are if statements only used for controlling LEDs?
No, if statements can control a wide range of outputs and behaviors, including motors, displays, and more, based on various sensor inputs.