How to Fix Arduino Exit Status 1 Error
-
Solving
Exit Status 1
Error UsingPreferences
in Arduino IDE -
Check Syntax and Typos to Fix Arduino
Exit Status 1
Error -
Check Library Dependencies to Fix Compilation Error:
Exit Status 1
in Arduino - Verify Board and Port Settings
- Update Arduino IDE and Cores
- Check Memory Usage
-
Review
PlatformIO
Configuration - Conclusion
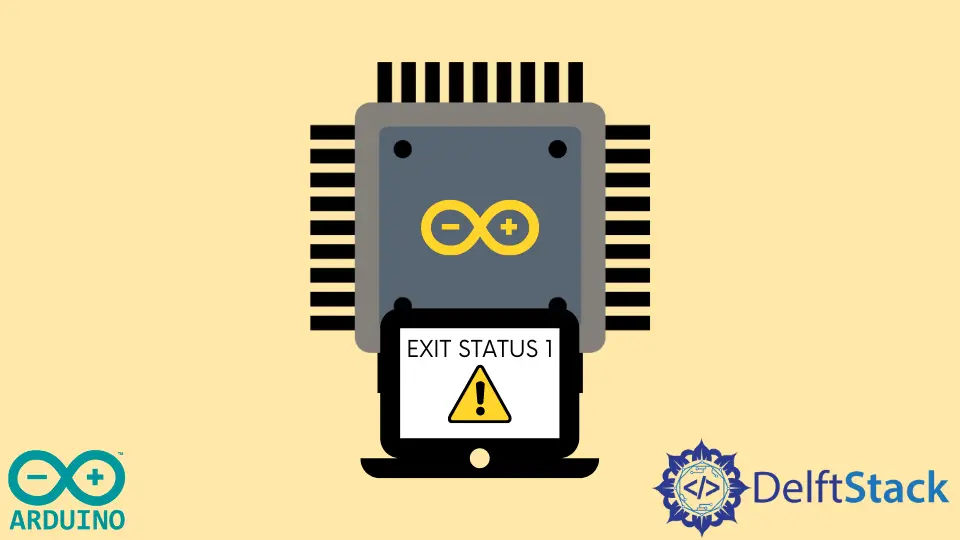
The Arduino Exit Status 1
is a compile-time error that’s a common challenge faced by Arduino developers and hobbyists. This error message can be cryptic, and finding the root cause may seem daunting.
In this guide, we will explore various methods to diagnose and fix the Arduino Exit Status 1
error, providing detailed explanations and example codes for each approach.
Solving Exit Status 1
Error Using Preferences
in Arduino IDE
To get more information about the compilation process, you can increase the compiler’s verbose output.
Navigate to "File"
> "Preferences"
and check the "Show verbose output during"
compilation box. After enabling verbose output, review the console messages for clues about the specific error.
When you get this error, you will also see a description of the error present in your code that you can now fix easily. This error also arises when someone uses a function from a library and forgets to include it in the Arduino code.
So, make sure you have included all the libraries whose functions you are using in the code. If you are using a library that is not present in the Arduino IDE or some .h
file in your code, you have to put that library in the same folder where your Arduino code is present.
For example, check the below code:
#include <LiquidCrystal.h>
void setup() { LiquidCrystal lcd(8, 9, 4, 5, 6, 7); }
In the above code, we have included a library, LiquidCrystal.h
, and then we have used it in our code. If we do not include this library, we will get a compile-time error.
So, make sure to include all the libraries before you use them in your code. If it still shows an error, install the included library.
Check Syntax and Typos to Fix Arduino Exit Status 1
Error
One of the most common causes of the Exit Status 1
error is a syntax error or a typo in your Arduino code. Ensure that your code follows the correct syntax and that there are no misspelled words or missing semicolons.
Here’s an example:
void setup() { pinMode(LED_BUILTIN, OUTPUT); }
void loop() {
digitalWrite(LED_BUILTIN, HIGH); // Correct statement
delay(1000);
digitalWrite(LED_BUILTIN, LOW); // Correct statement
delay(1000);
}
In this example, make sure there are no typos in function names, variable names, or missing punctuation marks.
Check Library Dependencies to Fix Compilation Error: Exit Status 1
in Arduino
If your code relies on external libraries, make sure they are correctly installed. Incorrect or missing libraries can lead to the Exit Status 1
error.
Verify that you have included the necessary libraries in your sketch. Here’s an example:
#include <Wire.h> // Correct library inclusion
void setup() {
// Your setup code here
}
void loop() {
// Your loop code here
}
Ensure that all library dependencies are correctly specified at the beginning of your sketch.
Verify Board and Port Settings
Make sure you have selected the correct board and port in the Arduino IDE. Incorrect settings can lead to compilation errors.
Navigate to "Tools"
> "Board"
and select the appropriate board. Also, verify the selected port under "Tools"
> "Port"
.
// Your code here
void setup() {
// Your setup code here
}
void loop() {
// Your loop code here
}
Ensure that the board and port settings match your hardware configuration.
Update Arduino IDE and Cores
Ensure that you are using the latest version of the Arduino IDE and board cores. Outdated software may contain bugs that have been addressed in newer releases.
Update your IDE and board cores to the latest versions to eliminate potential compatibility issues.
Check Memory Usage
Large or inefficient code can exceed the available memory on your Arduino board, leading to compilation errors. Check the memory usage of your sketch using the following example:
// Your code here
void setup() {
// Your setup code here
Serial.begin(9600);
Serial.println("Free memory: " + String(freeMemory()) + " bytes");
}
void loop() {
// Your loop code here
}
int freeMemory() {
extern int __heap_start, *__brkval;
int v;
return (int)&v - (__brkval == 0 ? (int)&__heap_start : (int)__brkval);
}
This code snippet includes a function to calculate free memory. If your sketch is pushing the memory limits, consider optimizing your code or upgrading to a board with more memory.
Review PlatformIO
Configuration
If you are using PlatformIO
with Visual Studio Code instead of the Arduino IDE, ensure that your platformio.ini
configuration file is correct. Verify that the board, framework, and other settings are properly configured.
[env:myboard]
platform = ...
board = ...
framework = ...
Review and adjust the platformio.ini
file to match your hardware and project requirements.
Conclusion
The Arduino Exit Status 1
error can be caused by various factors, ranging from simple syntax errors to more complex issues like memory limitations.
By following the methods outlined in this guide and carefully reviewing your code, libraries, and settings, you can troubleshoot and resolve the Exit Status 1
error efficiently. Always remember to check for updates and leverage the resources available in the Arduino community for further assistance.