Arduino dtostrf() Function
-
Overview of the Arduino
dtostrf
Function -
Syntax of Arduino
dtostrf
-
Basic Conversion Using the
dtostrf
Function -
Convert Double to ASCII Using the
dtostrf()
Function - Handling Negative Numbers
- Handling Large Numbers
- Tips and Considerations
- Conclusion
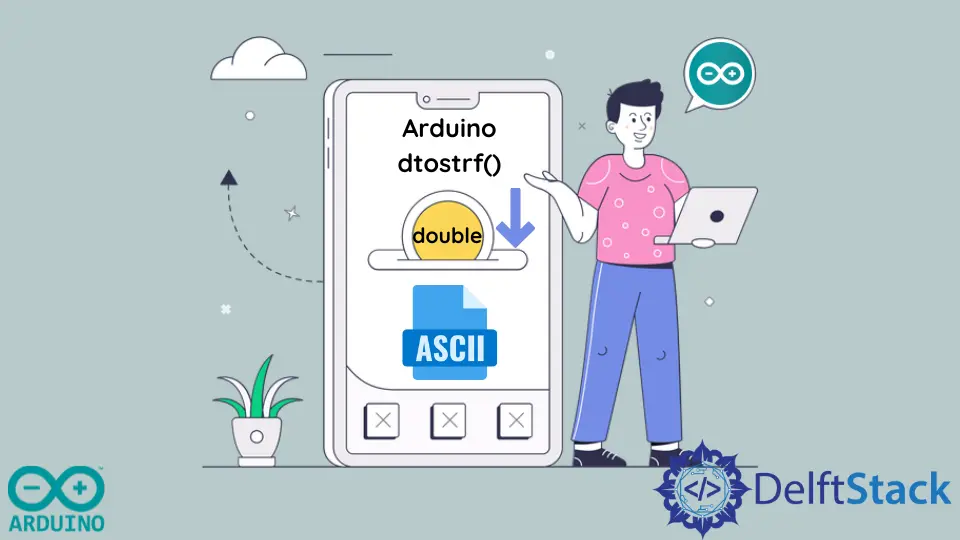
Arduino, with its user-friendly environment, has become a go-to platform for hobbyists and professionals alike in the field of embedded systems and microcontroller programming. When dealing with numeric data in Arduino, formatting and converting values to strings is a common requirement.
The dtostrf
function plays a crucial role in achieving this task. In this article, we will explore the dtostrf
function, its purpose, and syntax and provide detailed examples to illustrate its usage.
We will discuss how to use the dtostrf()
function to convert a variable of type double into its ASCII representation and store it as a string.
Overview of the Arduino dtostrf
Function
The dtostrf
function is primarily used for converting floating-point numbers to strings in Arduino. The name dtostrf
stands for “double to string format”, indicating its purpose of converting double-precision floating-point numbers to a string representation with specified formatting.
This function is particularly useful when you have to display or transmit numeric values as strings, a common scenario in applications involving sensors, displays, or communication protocols.
Syntax of Arduino dtostrf
The syntax of the Arduino dtostrf
function is as follows:
char* dtostrf(double val, signed char width, unsigned char precision, char* s);
Parameters:
val
: The double-precision floating-point number to be converted to a string.width
: The minimum number of characters to be printed. If the resulting string is shorter than this number, it will be padded with spaces.precision
: The number of digits to be printed after the decimal point.s
: Achar
array (buffer) where the resulting string will be stored. This array should be large enough to accommodate the converted string.
Basic Conversion Using the dtostrf
Function
void setup() {
Serial.begin(9600);
double temperature = 25.6789;
char str[10]; // Allocate a character array to store the result
// Convert the double to a string with 2 decimal places and a minimum width of
// 7 characters
dtostrf(temperature, 7, 2, str);
// Print the result
Serial.println("Temperature: " + String(str));
}
void loop() {
// Your main code here
}
In this example, we have a double variable temperature
with a value of 25.6789
. We want to convert this to a string with 2 decimal places and a minimum width of 7 characters.
The result is stored in the character array str
and then printed to the Serial
monitor.
The output will be:
Temperature: 25.68
Convert Double to ASCII Using the dtostrf()
Function
The dtostrf()
function is a part of the Arduino Standard Peripheral Library (SPL). It is specifically designed to convert double-precision floating-point numbers to ASCII strings.
The dtostrf()
function takes four input parameters:
- The first is a variable of type
double
, which we want to convert. - The second is a variable of type
char
used to set the width of the output variable or number of digits. - The third is a variable of type
char
used to set the number of digits after the decimal place. - The fourth is a variable of type
char
to which the conversion will be stored.
For example, see the below code:
double a = 123.123;
char x[8];
void setup() {
Serial.begin(9600);
dtostrf(a, 5, 2, x);
Serial.println(x);
}
void loop() {}
In the above code, a
is a variable of type double
to store the given variable, and x
is a variable of type char
to store the result of conversion. The result of this conversion will be 123.12
.
Handling Negative Numbers
void setup() {
Serial.begin(9600);
double voltage = -12.345;
char buffer[10];
dtostrf(voltage, 8, 3, buffer);
Serial.println(buffer);
}
void loop() {
// Nothing in the loop for a one-time execution
}
In this code, voltage
is a negative double-precision floating-point number.
8
is the minimum width of the resulting string. 3
is the number of digits after the decimal point.
The output will be:
-12.345
Handling Large Numbers
void setup() {
Serial.begin(9600);
double largeNumber = 12345.6789;
char buffer[15];
dtostrf(largeNumber, 10, 4, buffer);
Serial.println(buffer);
}
void loop() {
// Nothing in the loop for a one-time execution
}
In this code, largeNumber
is a double-precision floating-point number.
10
is the minimum width of the resulting string. 4
is the number of digits after the decimal point.
The output will be:
12345.6790
Tips and Considerations
- Buffer Size: Ensure that the
char
array used as a buffer (s
in the function) is large enough to accommodate the converted string, considering the specified width and precision. - Floating-Point Precision: Be mindful of the precision parameter. Adjust it based on your specific requirements to avoid unnecessary trailing zeros or loss of precision.
- Library Dependencies: The
dtostrf
function is part of the Arduino core library. No additional libraries need to be included for its usage. - Memory Usage: Converting floating-point numbers to strings can be memory-intensive. Consider the available memory on your Arduino board, especially when dealing with large arrays of such conversions.
Conclusion
The dtostrf
function is a valuable tool in the Arduino programmer’s toolkit when dealing with floating-point to string conversions. Whether you are displaying sensor readings, communicating with other devices, or simply logging data, understanding and mastering this function empowers you to handle numeric data effectively in string format.
With the examples provided, you can now confidently incorporate dtostrf
into your Arduino projects, enhancing their functionality and versatility.