How to Add Delay in Microseconds in Arduino
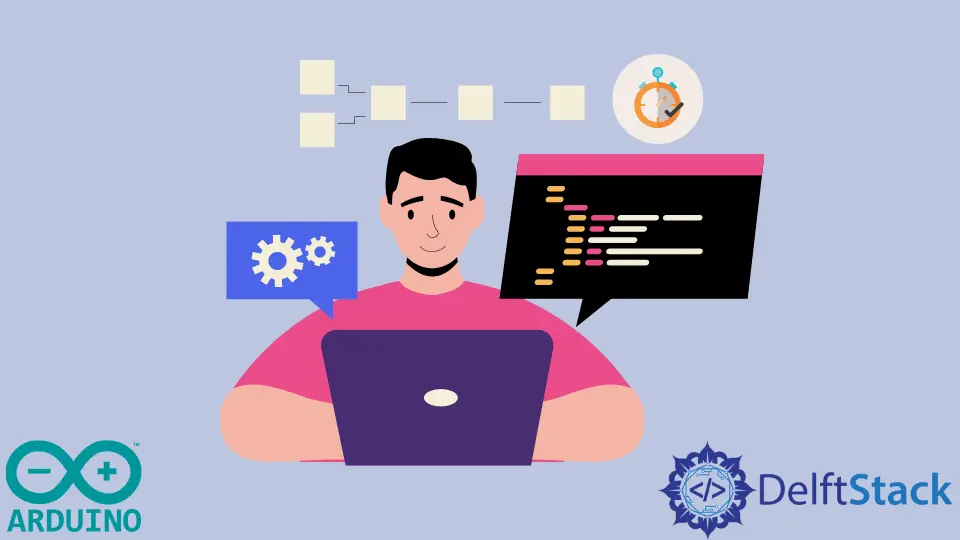
When working with Arduino, precise timing is often crucial for achieving desired functionality. Whether you’re controlling motors, reading sensors, or communicating with other devices, you may need to introduce short delays in your code. Fortunately, Arduino provides a straightforward way to add delays in microseconds using the delayMicroseconds()
and micros()
functions.
In this article, we will delve into these functions, explore their applications, and learn how to implement them effectively in your Arduino projects. By the end, you’ll have a solid understanding of how to manage time-sensitive tasks with ease.
To further enhance your Arduino skills, you might also be interested in learning how to make a counter in Arduino, which can be useful for applications where counting operations are critical.
Understanding delayMicroseconds() Function
The delayMicroseconds()
function is designed to pause the execution of your program for a specified number of microseconds. This function is particularly useful for tasks that require precise timing, such as generating PWM signals or controlling the timing of sensor reads. The syntax is simple:
delayMicroseconds(microseconds);
Here, microseconds
is the duration of the delay in microseconds. This function can handle delays from 1 to 16383 microseconds, which is ideal for many applications.
Let’s look at an example where we use delayMicroseconds()
to blink an LED with a very short delay:
const int ledPin = 13;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
digitalWrite(ledPin, HIGH);
delayMicroseconds(500); // LED ON for 500 microseconds
digitalWrite(ledPin, LOW);
delayMicroseconds(500); // LED OFF for 500 microseconds
}
In this code, we set up an LED on pin 13. The LED turns on for 500 microseconds, then off for the same duration, creating a rapid blinking effect.
Output:
LED blinks rapidly on and off
The delayMicroseconds()
function is efficient for short delays and ensures that your Arduino can perform other tasks during the wait. However, keep in mind that while the function is running, other code will be paused, which can affect the performance of time-sensitive applications.
Using micros() Function for Timing
While delayMicroseconds()
is great for introducing delays, the micros()
function can be useful for measuring elapsed time in microseconds. This function returns the number of microseconds since the Arduino board began running the current program. The syntax is straightforward:
unsigned long currentMicros = micros();
You can use micros()
to create your own timing loops, allowing for more complex timing control without blocking the execution of your program. Here’s an example that demonstrates how to use micros()
for timing:
const int ledPin = 13;
unsigned long previousMicros = 0;
const long interval = 1000; // interval in microseconds
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
unsigned long currentMicros = micros();
if (currentMicros - previousMicros >= interval) {
previousMicros = currentMicros;
digitalWrite(ledPin, !digitalRead(ledPin)); // toggle LED
}
}
In this example, we toggle the LED state every 1000 microseconds (1 millisecond). We use micros()
to check the elapsed time since the last toggle. This non-blocking approach allows the Arduino to perform other tasks while still managing precise timing.
Output:
LED toggles every 1 millisecond
Using micros()
provides greater flexibility in timing control. It allows your program to remain responsive while still managing time-sensitive tasks. This method is particularly advantageous in applications where multiple processes need to run concurrently.
If you are interested in serial communication with Arduino, understanding how to set baud rate in Arduino serial communication is essential for ensuring the accuracy and reliability of data transmission.
Conclusion
Adding delays in microseconds using Arduino is a straightforward process that can greatly enhance your projects. Whether you choose to use delayMicroseconds()
for simple delays or micros()
for more complex timing control, both functions serve essential roles in ensuring your code runs smoothly. Mastering these functions enables you to create responsive and precise applications, whether you’re controlling hardware or managing data. With these tools at your disposal, you’re well on your way to becoming an Arduino timing expert.
FAQ
-
What is the maximum delay I can set using delayMicroseconds?
The maximum delay you can set with delayMicroseconds is 16383 microseconds. -
Can I use delayMicroseconds in the loop function?
Yes, you can use delayMicroseconds in the loop function, but be aware that it will block other code from executing during the delay. -
How accurate is the micros function?
The micros function is generally accurate to within a few microseconds, but it may vary depending on the specific Arduino board and the current load on the processor. -
Can I use delayMicroseconds for longer delays?
For delays longer than 16383 microseconds, you can use a combination of delay() and delayMicroseconds for greater flexibility. -
Is it possible to use both delayMicroseconds and micros in the same program?
Yes, you can use both functions in the same program to manage different timing needs effectively.