How to Program Arduino With C++
- Setting Up the Arduino IDE
- Writing Your First C++ Program for Arduino
- Understanding Arduino Libraries and Functions
- Debugging Your Arduino Code
- Conclusion
- FAQ
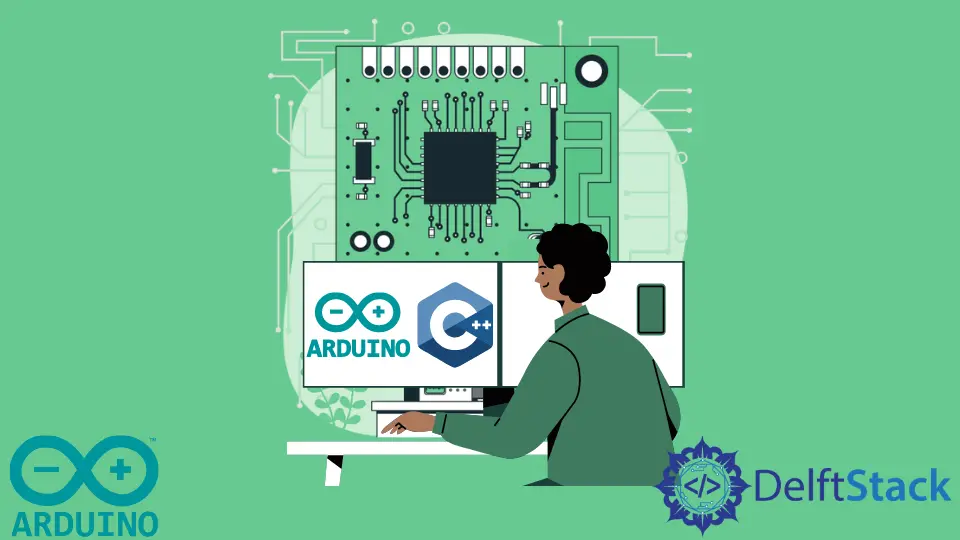
Programming an Arduino can be a thrilling experience, especially when you dive into the world of C++. The Arduino platform, known for its versatility and ease of use, allows hobbyists and professionals alike to create impressive projects.
In this tutorial, we will explore how to program an Arduino using C++ through the Arduino Integrated Development Environment (IDE). Whether you are looking to control LEDs, read sensors, or build complex systems, understanding the basics of C++ for Arduino is essential. Let’s get started on your journey to becoming an Arduino programming pro!
Setting Up the Arduino IDE
Before you can start programming your Arduino, you need to set up the Arduino IDE. This software is the gateway to writing and uploading your C++ code to the Arduino board.
-
Download the Arduino IDE: Visit the official Arduino website and download the IDE for your operating system (Windows, macOS, or Linux).
-
Install the IDE: Follow the installation instructions provided on the website. The process is straightforward, and you should have the IDE up and running in no time.
-
Connect Your Arduino Board: Use a USB cable to connect your Arduino board to your computer. The IDE should automatically recognize the board.
-
Select the Board and Port: In the Arduino IDE, go to Tools > Board and select your Arduino model. Then, navigate to Tools > Port and select the appropriate COM port.
Once you have completed these steps, you are ready to start programming your Arduino with C++.
Writing Your First C++ Program for Arduino
Now that your IDE is set up, let’s write a simple C++ program. We will create a basic sketch that blinks an LED connected to pin 13 of the Arduino board. This is often the first program that many Arduino users write, and it serves as a great introduction to C++ on the platform.
void setup() {
pinMode(13, OUTPUT);
}
void loop() {
digitalWrite(13, HIGH);
delay(1000);
digitalWrite(13, LOW);
delay(1000);
}
Output:
LED connected to pin 13 will blink every second.
In this code, the setup
function runs once when you power up the board. It sets pin 13 as an output pin. The loop
function runs repeatedly, turning the LED on for one second and then off for another second. The delay(1000)
function pauses the program for 1000 milliseconds (1 second). This simple program demonstrates the structure of an Arduino sketch and the basics of C++ syntax.
Understanding Arduino Libraries and Functions
One of the strengths of programming Arduino with C++ is the extensive libraries available. Libraries are collections of pre-written code that simplify complex tasks. For instance, if you want to use a temperature sensor, you can include a library specifically designed for that sensor, making your code cleaner and easier to understand.
To include a library, you simply use the #include
directive at the top of your sketch. Here’s an example of how to use the DHT
library for a temperature and humidity sensor.
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
void setup() {
Serial.begin(9600);
dht.begin();
}
void loop() {
delay(2000);
float h = dht.readHumidity();
float t = dht.readTemperature();
Serial.print("Humidity: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print("Temperature: ");
Serial.print(t);
Serial.println(" *C");
}
Output:
Humidity: 45.00 % Temperature: 24.00 *C
In this example, we first include the DHT library. We define the pin and type of the sensor, then create an instance of the DHT class. In the setup
function, we initialize the serial communication and the sensor. The loop
function reads the humidity and temperature every two seconds, printing the values to the Serial Monitor. Libraries like DHT make it easy to interact with hardware without having to write complex code from scratch. For fine-tuning memory operations in your Arduino projects, you might consider exploring the Arduino memset() Function to set or clear data efficiently.
Debugging Your Arduino Code
Debugging is an essential skill for any programmer, and Arduino programming is no exception. When things don’t work as expected, you need to find the root of the problem. Here are some tips for effective debugging in Arduino:
-
Use Serial Monitor: The Serial Monitor is a powerful tool for debugging. You can print variable values and messages to the Serial Monitor using
Serial.print()
andSerial.println()
. This allows you to track the flow of your program and identify where things might be going wrong. -
Check Connections: Sometimes, the issue might not be in your code but in your hardware connections. Ensure that all components are connected properly and securely.
-
Simplify Your Code: If you encounter a problem, try simplifying your code. Remove or comment out parts of your program to isolate the issue.
-
Consult Documentation: Arduino has extensive documentation and community forums. If you’re stuck, searching online can often lead to a solution.
By employing these debugging techniques, you can streamline your programming process and enhance your understanding of how your code interacts with the hardware.
Conclusion
Programming an Arduino with C++ opens up a world of possibilities for both beginners and experienced developers. With the Arduino IDE, you can easily write, compile, and upload your code to the board. By understanding the basic structure of an Arduino sketch, utilizing libraries, and mastering debugging techniques, you can create impressive projects that respond to the physical world around you. Whether you want to build a simple LED blink program or a complex sensor network, the skills you learn will serve you well in your Arduino journey.
FAQ
-
How do I install libraries in the Arduino IDE?
You can install libraries by going to Sketch > Include Library > Manage Libraries in the Arduino IDE. Search for the library you need and click Install. -
What is the difference between setup() and loop() functions?
Thesetup()
function runs once when the Arduino is powered on, while theloop()
function runs continuously aftersetup()
has finished. -
Can I use C++ features like classes and inheritance in Arduino programming?
Yes, you can use C++ features, including classes and inheritance, in your Arduino sketches, allowing for more complex and organized code. -
How can I debug my Arduino code effectively?
You can use the Serial Monitor to print variable values and messages, check hardware connections, simplify your code, and consult documentation for help. -
What are some common libraries used in Arduino programming?
Some popular libraries include the DHT library for temperature and humidity sensors, the Servo library for controlling servos, and the LiquidCrystal library for LCD displays.