How to Make a Counter in Arduino
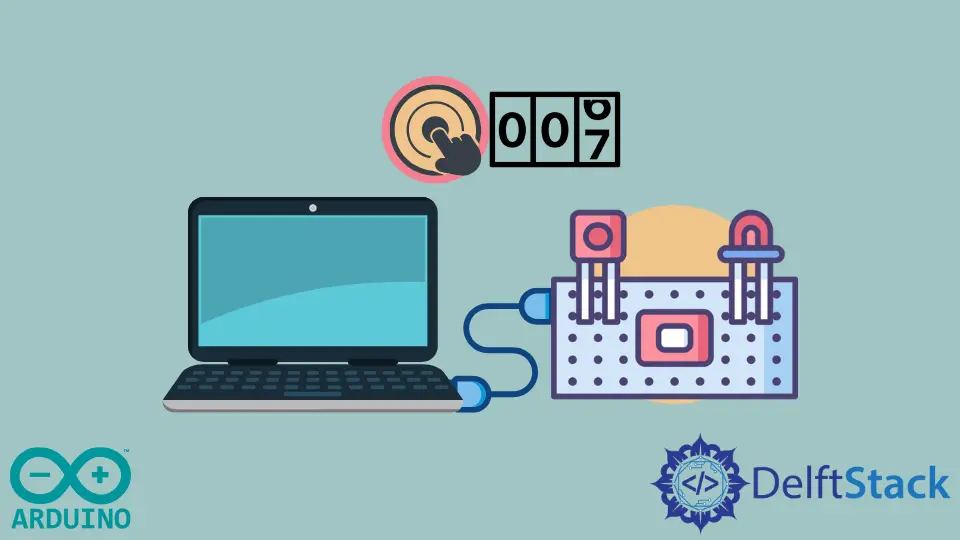
This tutorial will discuss making a counter using a loop in Arduino.
Make a Counter in Arduino
Counters are used to count a process, like the number of times a button is pressed. A counter can also carry out an operation a specific number of times.
We can use a loop to make a counter in Arduino. For example, if we want an LED to blink 10 times, we can use a for
or a while
loop to turn an LED ON and OFF multiple times.
We have to attach the positive terminal of the LED with a digital pin of Arduino and the negative terminal to the negative of a battery. We can also use a resistor to limit the current flow through the LED.
We can use the digitalWrite()
function of Arduino to turn an LED ON and OFF. We have to pass the digital PIN where the LED is attached as the first argument of the function and the status that we want to give to the LED.
If we want to turn the LED ON, we can pass HIGH
as the second argument, and if we’re going to turn the LED OFF, we can pass LOW
in the digitalWrite()
function. We also have to give a delay to see the blinking of the LED using the delay()
function.
Check the example code below.
int LED_pin = 13;
void setup() { pinMode(LED_pin, OUTPUT); }
void loop() {
for (int count = 0; count < 10; count = count + 1) {
digitalWrite(LED_pin, HIGH);
delay(500);
digitalWrite(LED_pin, LOW);
delay(500);
}
}
We have to use PIN 13 of the Arduino and the pinMode()
function to set the mode of the pin to output. In the for
loop, the first argument is the starting value of the count, and the second argument is the condition that will break the loop if true.
The third argument sets the increment in the count. Inside the loop, we set the LED pin to high for 250 milliseconds and then to low for 250 milliseconds.
The loop will repeat until the count variable becomes 10. Instead of a for
loop, we can also use a while
loop to make a counter.
If we want to blink the LED under certain conditions, like when a button is pressed, we can use the digitalRead()
function to read input from a button, and then we can use the if-else
statement to blink the LED.
For example, if the button is pressed, the LED will blink 10 times, and if not, the LED will remain OFF.