How to Convert String to Char in Arduino
- Introduction to the toCharArray() Function
-
Convert
String
tochar
Using thetoCharArray()
Function in Arduino -
Convert Data to
char
Using thetoCharArray()
Function and the Append Operator in Arduino - Practical Examples
- Conclusion
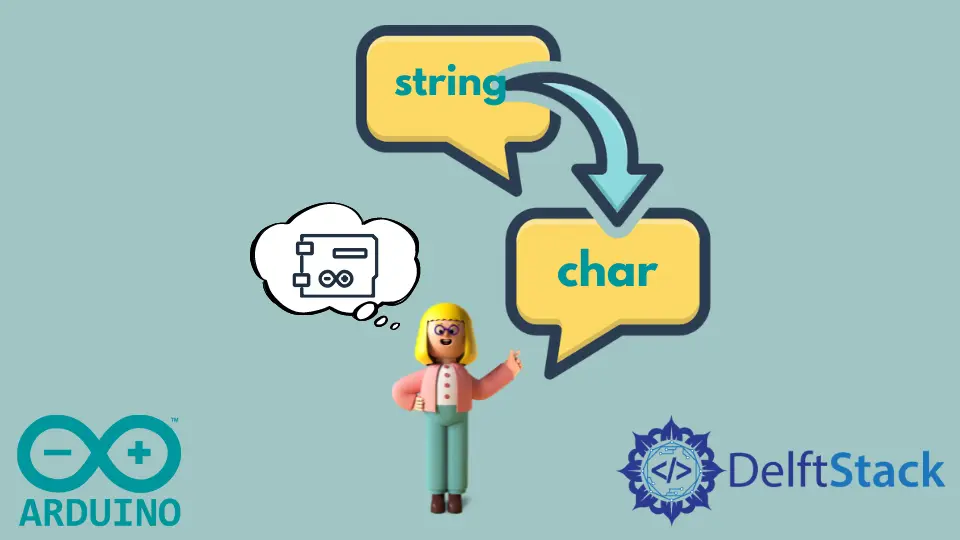
This tutorial will discuss a method to convert a string
into char
- the toCharArray()
function. It will also introduce how to convert other data types into char
using the toCharArray()
function and append operator.
Introduction to the toCharArray() Function
In Arduino programming, a String
is a flexible data type used to store character sequences. However, there are situations where you need to work with char
arrays, particularly when interacting with libraries or APIs that expect character arrays as inputs. This is where the toCharArray()
function comes to the rescue.
The toCharArray()
function allows you to convert the contents of a String
object into a char
array. It provides a seamless way to bridge the gap between these two data types.
Syntax of the toCharArray() Function
The syntax of the toCharArray()
function is straightforward:
stringObject.toCharArray(charArray, length);
stringObject
: TheString
object you want to convert.charArray
: The targetchar
array to store the converted characters.length
: The length of theString
object plus one for the null terminator.
Convert String
to char
Using the toCharArray()
Function in Arduino
This method copies the string’s characters to the supplied buffer. It requires two inputs, one is a buffer to copy the characters into, and the other is the buffer size.
void loop() {
String stringOne = "A string";
char Buf[50];
stringOne.toCharArray(Buf, 50);
}
In the above code, stringOne
is the String
object where the string is stored. Buf
is the char
array where the result is going to be saved. In this example, we use a buffer length of 50, but you can change that according to the given string. Check the link for more details.
Convert Data to char
Using the toCharArray()
Function and the Append Operator in Arduino
If you want to convert any other data type instead of String
, you can use it. First of all, you need to convert the other data type into String
using the append operator; then, you can use the above method to convert this String
into char
.
void loop() {
String stringOne = "A long integer: ";
stringOne += 1234;
char charBuf[50];
stringOne.toCharArray(charBuf, 50);
}
In the above code, we have an integer - 1234
and add it to an existing String
using the append operator to convert them into a char
array. Check this link for more information.
Practical Examples
Let’s explore a couple of practical examples to solidify your understanding.
Example 1: Sending Data over Serial
Suppose you want to send sensor readings over the serial port as a char
array. Here’s how you can do it:
void loop() {
int sensorValue = analogRead(A0);
String dataToSend = "Sensor reading: ";
// Append the sensor value to the String
dataToSend += sensorValue;
char charArray[dataToSend.length() + 1]; // +1 for the null terminator
dataToSend.toCharArray(charArray, dataToSend.length() + 1);
Serial.println(charArray); // Send the data over serial
}
Example 2: Displaying on an LCD Screen
Imagine you want to display a dynamic message on an LCD screen. Here’s how:
#include "LiquidCrystal.h"
LiquidCrystal lcd(12, 11, 5, 4, 3, 2);
void setup() { lcd.begin(16, 2); }
void loop() {
int sensorValue = analogRead(A1);
String message = "Sensor value: ";
// Append the sensor value to the String
message += sensorValue;
char charArray[message.length() + 1]; // +1 for the null terminator
message.toCharArray(charArray, message.length() + 1);
lcd.clear();
lcd.print(charArray); // Display the message on the LCD screen
}
Conclusion
Mastering the toCharArray()
function in Arduino empowers you to seamlessly convert String
objects to char
arrays and work with them effectively.
Whether you’re sending data over serial communication, displaying messages on an LCD screen, or interacting with external libraries, the ability to perform this conversion is a crucial skill.
By following the guidelines and practical examples in this guide, you’re now equipped to handle such scenarios with confidence.
Related Article - Arduino String
- Arduino strcmp Function
- Arduino Strcpy Function
- How to Concatenate Strings in Arduino
- How to Parse a String in Arduino
- How to Split String in Arduino
- How to Compare Strings in Arduino