How to Convert Byte to Integer in Arduino
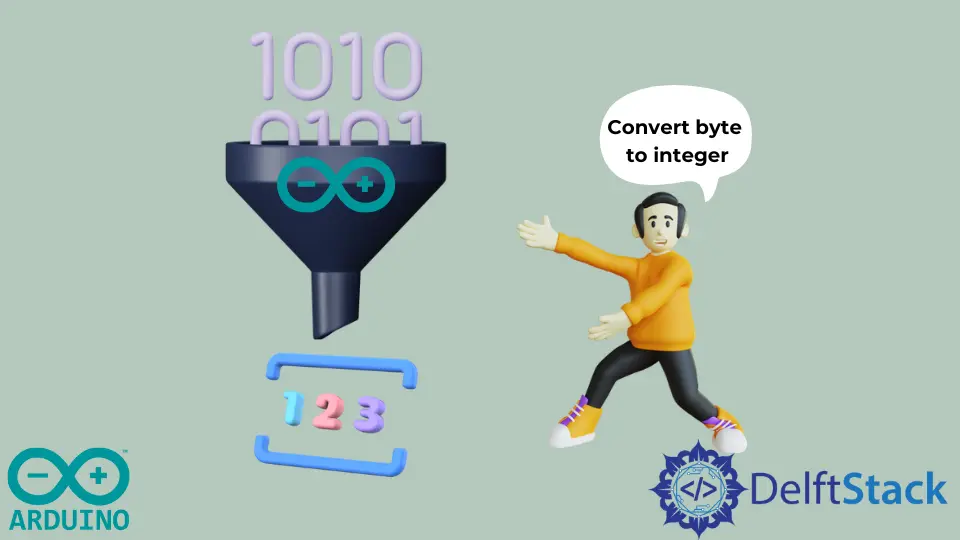
This tutorial will discuss converting a byte variable into an integer variable using the int()
function in Arduino.
Arduino Byte to Integer Conversion
A byte consists of 8 bits, and the value of each bit can be 0 or 1. To store an integer, we need 4 bytes of memory.
The integer data type consists of mostly decimal numbers, and when we store them, they are converted into bits because a computer only understands and works with bits of data in the form of zeros and ones.
The byte and integer data types are available in Arduino, and we can convert each data type to another using specific functions.
To convert a byte variable to an integer variable, we can use the int()
function of Arduino. For example, let’s define a byte variable and then convert it into an integer using the int()
function and print the result using the serial monitor of Arduino.
Code:
byte b = 524;
void setup() {
int i = int(b);
Serial.begin(9600);
Serial.println(i);
}
void loop() {}
Output:
12
The Serial.begin()
function initializes the serial monitor with the given baud rate or speed and prints the variable on the serial monitor window.
Note that the variable that we want to convert should be in byte data type, and if it is not in byte data type, we have to store it inside a byte to convert it into an integer.
If we define the byte in the above code as an integer data type, it will not be converted to an integer as it is already into an integer data type.
From the output, the byte value 524
is equal to the integer value 12
. Arduino functions return data in bytes, and it is recommended that we look at the reference of a function on the Arduino website before using it.
Using the byte()
function, we can also convert an integer or other data types to byte using the byte()
function.