How to Set Baud Rate in Arduino Serial Communication
- Understanding Baud Rate
- Setting Baud Rate in Arduino Code
- Troubleshooting Baud Rate Issues
- Conclusion
- FAQ
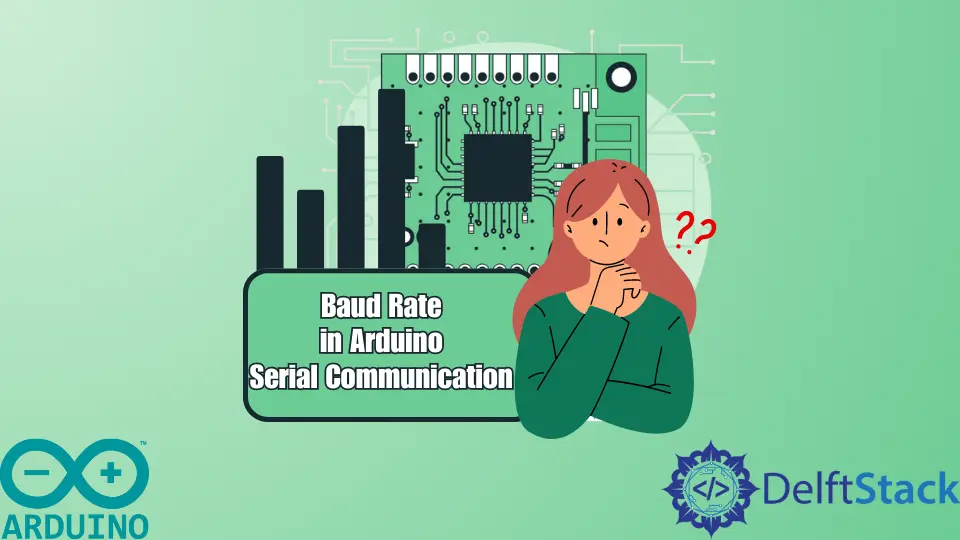
Arduino’s serial communication is a powerful tool that allows for effective data exchange between devices. One of the critical aspects of this communication is the baud rate, which determines how fast data is transmitted. Setting the correct baud rate is essential for ensuring that your Arduino can communicate effectively with other devices, such as computers or sensors.
In this article, we’ll dive deep into understanding baud rates in Arduino, how to set them, and the implications of different settings. Whether you’re a beginner or an experienced developer, this guide will provide you with the insights you need to optimize your Arduino projects.
Understanding Baud Rate
Baud rate is the rate at which information is transferred in a communication channel. In the context of Arduino, it defines the speed of serial communication, measured in bits per second (bps). Common baud rates include 9600, 115200, and 57600 bps. The baud rate must be set consistently on both the transmitting and receiving devices; otherwise, data corruption may occur.
When setting the baud rate in your Arduino code, you typically use the Serial.begin()
function. For example, if you want to set the baud rate to 9600 bps, you would write:
Serial.begin(9600);
This line of code initializes the serial communication at the specified baud rate. It’s crucial to match this baud rate with the settings of the device you’re communicating with, such as a computer or another microcontroller.
Understanding the implications of different baud rates is essential. Higher baud rates allow for faster data transmission but may also lead to increased errors, especially over longer distances or with poor-quality connections. Conversely, lower baud rates are more reliable but can slow down communication. For those interested in deeper control over memory, you might explore using the Arduino memset() Function to handle memory setting tasks efficiently.
Setting Baud Rate in Arduino Code
To set the baud rate in your Arduino sketch, you will primarily use the Serial.begin()
function in the setup()
section of your code. Here’s a simple example of how to implement this:
void setup() {
Serial.begin(9600);
}
void loop() {
Serial.println("Hello, Arduino!");
delay(1000);
}
In this code snippet, the setup()
function initializes serial communication at 9600 bps. The loop()
function sends a message to the serial monitor every second.
Output:
Hello, Arduino!
Hello, Arduino!
Hello, Arduino!
...
By setting the baud rate to 9600, the Arduino can effectively communicate with the serial monitor on your computer. You can adjust the baud rate to match your specific needs, but remember that both devices must use the same rate to ensure accurate data transmission.
Troubleshooting Baud Rate Issues
If you encounter issues with serial communication, the baud rate is often the first thing to check. Here are some troubleshooting tips:
- Mismatch: Ensure that the baud rate set in your Arduino code matches the baud rate in the serial monitor or the device you are communicating with. If issues persist, you may need to learn How to Read String in Arduino Serial Port for resolving discrepancies.
- Noise and Interference: If you are using longer cables or working in an electrically noisy environment, consider lowering the baud rate to improve reliability.
- Incorrect Settings: Double-check your Arduino IDE settings to ensure that the correct port and board are selected.
By paying attention to these details, you can significantly improve the reliability of your Arduino’s serial communication.
Conclusion
Setting the baud rate in Arduino serial communication is a fundamental skill that can significantly impact the performance of your projects. By understanding how to configure and troubleshoot baud rates, you can ensure that your Arduino communicates effectively with other devices. Whether you’re sending simple messages or complex data, getting the baud rate right is essential for successful communication.
FAQ
-
What is baud rate?
Baud rate is the speed of data transmission in a communication channel, measured in bits per second (bps). -
How do I set the baud rate in Arduino?
You set the baud rate in Arduino using theSerial.begin()
function in your code. -
What happens if the baud rates do not match?
If the baud rates do not match between devices, it can lead to data corruption and communication failures. -
What are common baud rates used in Arduino?
Common baud rates include 9600, 115200, and 57600 bps. -
Can I use any baud rate I want?
While you can choose various baud rates, it’s essential to select one that both devices support and can reliably communicate at.