How to Use jQuery in Angular 2
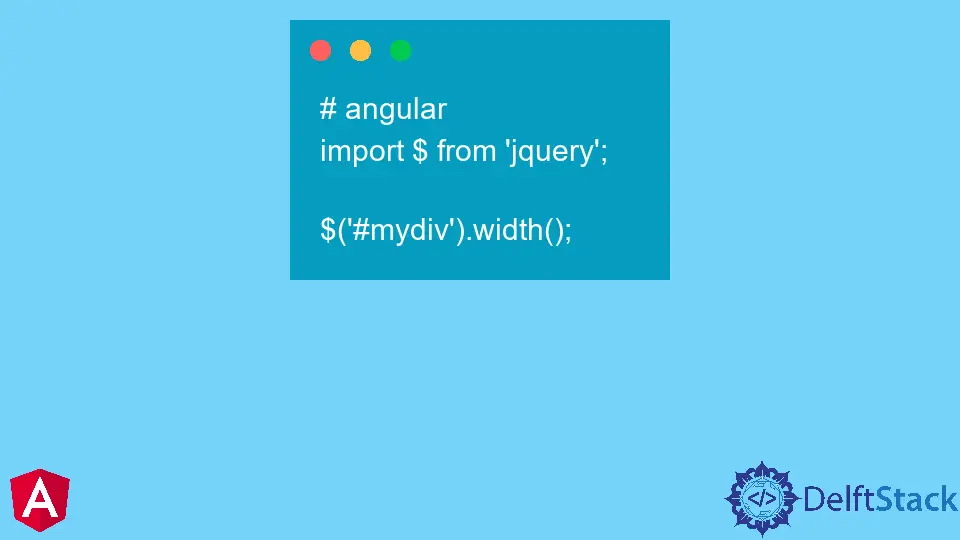
We will introduce how to use jQuery in angular 2.
jQuery in Angular
jQuery is a lightweight JavaScript library that makes it easier to use JavaScript on websites. If we have to use some library that uses jQuery as a requirement, we can easily install jQuery in our Angular 2 application and use it. But it is not a good idea to use it without any dependency. jQuery doesn’t give any major problems when we use it or integrate it into our Angular 2 application. But it doesn’t provide us anything out of the box if we use it without any dependency.
It is pretty easy to install jQuery in your Angular 2 application using the CLI command.
# angular
npm install --save jquery
After installing jQuery, we need to import it into our App files where we want to use it.
# angular
import $ from 'jquery';
$('#mydiv').width();
We can easily install and use jQuery in our angular applications without any problem with these two simple steps.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn