Two-Way Data Binding in Angular 2
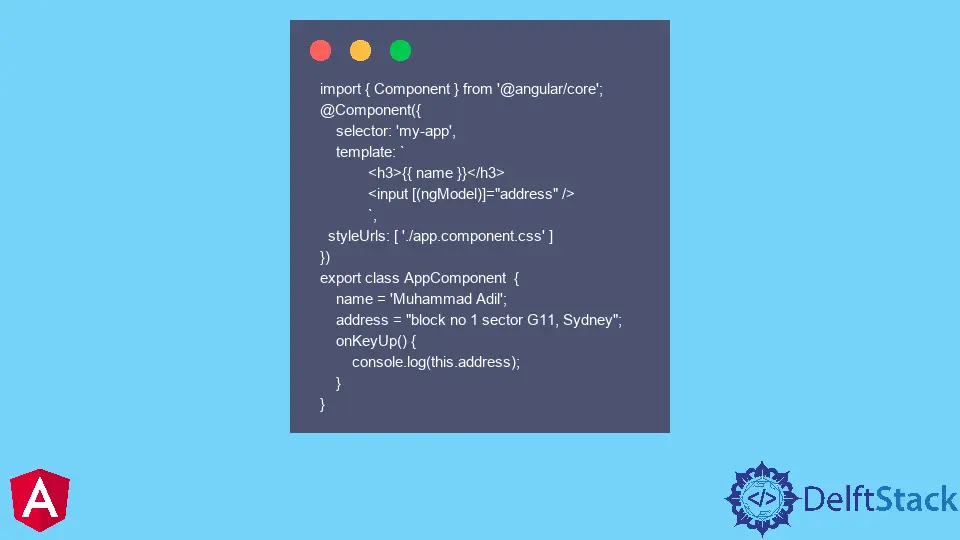
This tutorial will discuss implementing the two-way data binding in Angular 2.
Two-Way Data Binding in Angular
The two-way data binding is one of the most powerful features of Angular. It allows data to flow from the component to the view and vice versa.
It presents information to the user and enables them to change the underlying data through the user interface.
Data binding helps us manage our application state by keeping track of which parts of the UI are dependent on which data and updating whenever that data changes.
The critical difference between one-way and two-way binding is that with two-way binding, any changes made in either direction will automatically propagate to the other side without any intervention on our part.
Angular 2 doesn’t employ two-way data binding by default. It uses unidirectional data binding by definition, but it provides a simple syntax for two-way data binding if necessary.
To mimic two-way binding in Angular 2, we need to use the ngModel
directive and ngModelChange
event.
The ngModel
directive binds a property in your component’s template to a property in its class. The ngModelChange
event fires every time there’s a change in the value of that bound property.
Implement Two-Way Data Binding in Angular 2
ngModel
is a two-way data binding directive in Angular 2, which means it updates both the view and the model when there are changes made. We can set up an event handler on each binding side.
As a result, the corresponding property value automatically updates anytime the user modifies it on the user interface.
ngModel
can be used as an attribute or class on an HTML tag.
Let’s discuss an example of two-way data binding using ngModel
in Angular 2.
TypeScript code:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<h3>{{ name }}</h3>
<input [(ngModel)]="address" />
`,
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Muhammad Adil';
address = "block no 1 sector G11, Sydney";
onKeyUp() {
console.log(this.address);
}
}
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook