How to Set Default Option Value of Select From Typescript in AngularJs
-
Select Default Value by
Value
Attribute in AngularJS -
Select Default Value by
ngModel
Selector in AngularJS
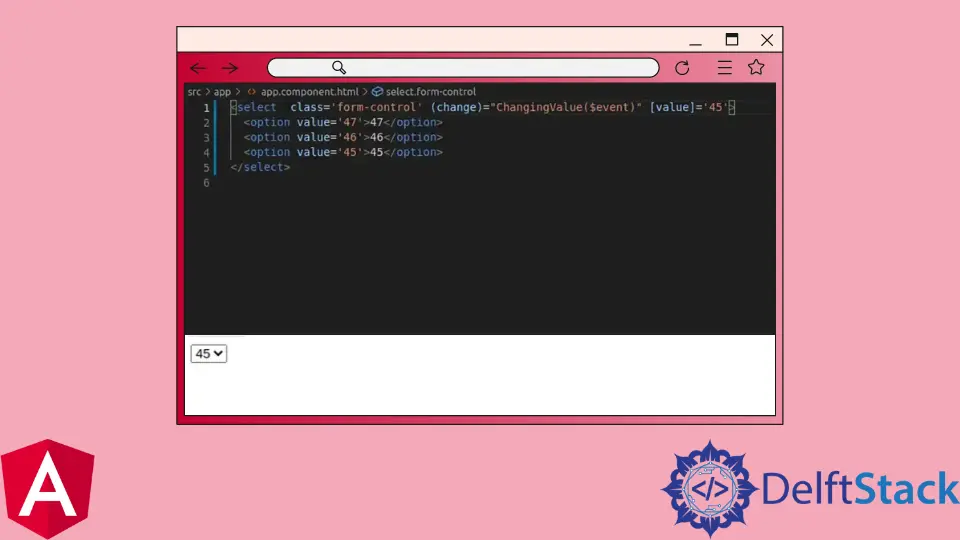
select
is an HTML tag that contains n
number of options child tags that contain the value prop.
This tutorial guide will provide how it can pick up the select default option value from TypeScript in AngularJs if the user does not select any particular defined value.
Select Default Value by Value
Attribute in AngularJS
We could define a default value for the select
tag by setting up the value
attribute inside an array parenthesis and assigning it a hard-coated value.
The default value
of the select
tag can only be assigned from an option value. This value can also be handled using the valueChanging
function called on change event.
The parameter passed to the valueChanging
function can be assigned with a default option.
<select class='form-handler'
(change)="valueChanging($event)" [value]='69'>
<option value='68'>68</option>
<option value='69'>69</option>
<option value='70'>70</option>
</select>
Output:
By default, select the default select
option. It would help if you remembered only putting the value as the default option within the select
option values.
Select Default Value by ngModel
Selector in AngularJS
We can use the ngModel
selector, a directive in AngularJs that binds input
, select
, textarea
, and saves the user
value in a variable.
The select
tag contains the ngModel
directive with a selectValue
attribute, and inside an app.component.ts
file, it is assigned with a default value same as it is present inside the option
tags.
app.component.html
:
<select [(ngModel)]='selectValue' class='form-control'>
<option value='47'>47</option>
<option value='46'>46</option>
<option value='45'>45</option>
</select>
app.component.ts
:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
selectValue:number = 47;
}
Output:
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn