Server-Side Rendering in AngularJS
- Server Side Rendering in AngularJS
-
the
Angular Universal
- Implement Server Side Rendering in AngularJS
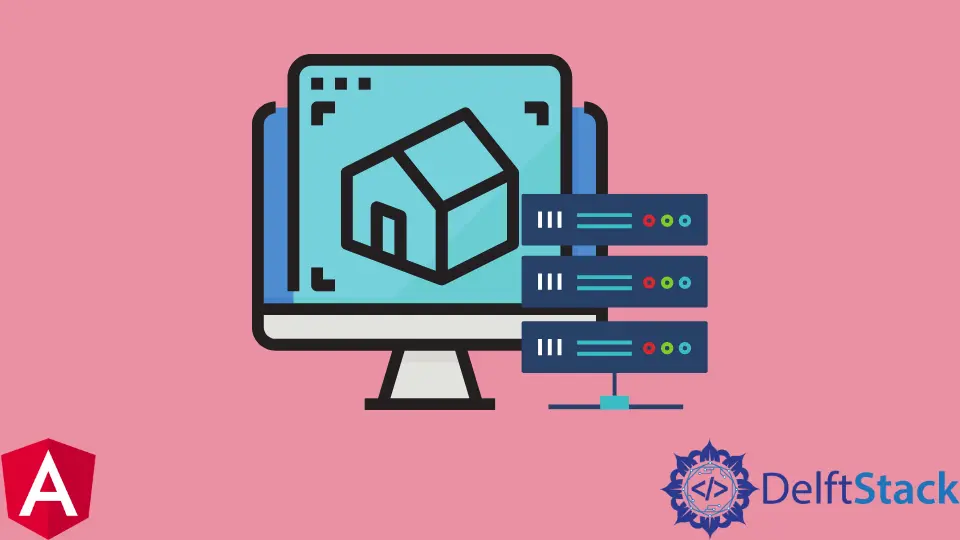
AngularJS is a front-end web application framework to build dynamic web pages. Angular Universal generates static pages on the server-side, then transmits them to the client browser to render.
As a result, Angular Universal speeds up the application’s rendering and allows users to see the layout.
This article will discuss Server-Side Rendering in AngularJS.
Server Side Rendering in AngularJS
Server-Side Rendering (SSR) refers to pre-rendering content on the server before delivering it to the client’s browser. It has several advantages that make it worth adopting.
It provides a faster loading time for the users and improves SEO by loading content as soon as possible. It also helps reduce costs by not using client-side rendering libraries.
Server-Side Rendering solves the slow initial page load by pre-rendering the server page before returning it to the user as HTML, rather than sending it as an empty document.
Server-Side Rendering can be achieved with AngularJS using its built-in module called ngRoute
.
the Angular Universal
Angular should be able to render in the server to support SSR. Angular provides a unique technology called Angular Universal
to make this possible.
It is a relatively new technology that is constantly evolving.
Implement Server Side Rendering in AngularJS
To implement Server-Side Rendering in AngularJS, we need to make sure that we have the server-side code and change some configuration settings of our project.
A common misconception about SSR is that it requires a complete rewrite of an application to implement. The truth is that SSR can be implemented incrementally by optimizing existing codebases with some minor changes.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook