onFocusEvent in Angular
- Understanding onFocusEvent in Angular
- Implementing onFocusEvent in Angular
- Advanced Usage of onFocusEvent in Angular
- Conclusion
- FAQ
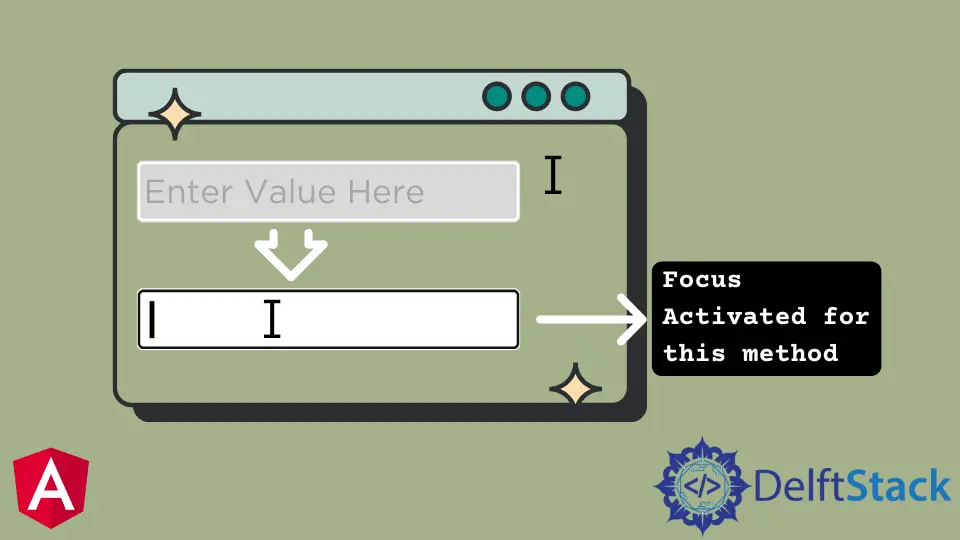
In the world of web development, Angular stands out for its powerful features and flexibility. One such feature is the onFocusEvent, which allows developers to handle focus events seamlessly.
This tutorial will walk you through the ins and outs of the onFocusEvent in Angular, demonstrating how it works and how you can effectively use it in your applications. Whether you’re a beginner or an experienced developer, understanding this event can enhance user interaction within your Angular applications. By the end of this article, you’ll have a solid grasp of how to implement and utilize the onFocusEvent for better user experience. Let’s dive in!
Understanding onFocusEvent in Angular
The onFocusEvent in Angular is an event binding that triggers when an HTML element gains focus. This is particularly useful for input fields, buttons, and other interactive elements. By capturing focus events, you can create dynamic user interfaces that respond to user actions, enhancing usability and engagement.
For instance, when a user clicks on a text input field, it gains focus, and you might want to highlight it or display additional information. Angular makes it easy to handle these events through its rich templating syntax. You can bind the focus event directly to a method in your component, allowing for clean and maintainable code.
Implementing onFocusEvent in Angular
To implement the onFocusEvent in Angular, you can use the following approach in your component and template. Here’s a straightforward example to get you started.
Step 1: Set Up Your Angular Component
First, create a component where you want to handle the focus event. Let’s assume we have a simple input field that we want to monitor for focus.
import { Component } from '@angular/core';
@Component({
selector: 'app-focus-event',
templateUrl: './focus-event.component.html',
})
export class FocusEventComponent {
message: string = '';
onFocus() {
this.message = 'Input field is focused!';
}
onBlur() {
this.message = 'Input field lost focus.';
}
}
In this TypeScript code, we define a component with two methods: onFocus
and onBlur
. The onFocus
method sets a message when the input field gains focus, while the onBlur
method does the opposite.
Step 2: Create the Template
Next, we need to create the HTML template for our component.
<div>
<input type="text" (focus)="onFocus()" (blur)="onBlur()" />
<p>{{ message }}</p>
</div>
In this template, we bind the focus and blur events of the input field to the respective methods in our component. When the input gains focus, the onFocus
method is triggered, updating the message displayed to the user.
Output:
Input field is focused!
When the input loses focus, the onBlur
method is executed.
Output:
Input field lost focus.
This simple implementation showcases how easy it is to handle focus events in Angular. By using event binding, you can enhance the interactivity of your applications, providing a better user experience.
Advanced Usage of onFocusEvent in Angular
Once you’re comfortable with the basics, you can explore more advanced uses of the onFocusEvent in Angular. For instance, you might want to perform additional actions when the input field gains focus, such as validating the input or displaying help text.
Example: Validating Input on Focus
Let’s modify our previous example to include input validation when the field gains focus.
import { Component } from '@angular/core';
@Component({
selector: 'app-focus-validation',
templateUrl: './focus-validation.component.html',
})
export class FocusValidationComponent {
message: string = '';
isValid: boolean = true;
onFocus() {
this.message = 'Please enter your name.';
}
onBlur(inputValue: string) {
this.isValid = inputValue.length > 0;
this.message = this.isValid ? 'Thank you!' : 'Name cannot be empty.';
}
}
In this code, we added a validation check in the onBlur
method. It checks if the input value is empty and updates the message accordingly.
Updated Template
Now, let’s update the HTML template to pass the input value to the onBlur
method.
<div>
<input type="text" (focus)="onFocus()" (blur)="onBlur($event.target.value)" />
<p>{{ message }}</p>
</div>
In this template, we use $event.target.value
to get the current input value when the field loses focus. This allows us to validate the input dynamically.
Output when the input is focused:
Please enter your name.
Output when the input is blurred with a valid entry:
Thank you!
Output when the input is blurred with an empty entry:
Name cannot be empty.
This advanced example demonstrates how you can integrate validation logic with focus events, further enhancing the user experience in your Angular applications.
Conclusion
In conclusion, mastering the onFocusEvent in Angular can significantly improve the interactivity of your applications. By capturing focus events, you can provide immediate feedback to users, guiding them through forms and other input elements. Whether you’re displaying messages, validating inputs, or enhancing user engagement, the onFocusEvent is a valuable tool in your Angular toolkit. With the examples provided, you now have a solid foundation to implement focus events in your projects. Happy coding!
FAQ
-
What is the onFocusEvent in Angular?
The onFocusEvent in Angular is an event binding that triggers when an HTML element gains focus, allowing developers to respond to user interactions. -
How do I implement the onFocusEvent in my Angular application?
You can implement the onFocusEvent by binding it to a method in your component using Angular’s event binding syntax in your template. -
Can I perform validation using the onFocusEvent?
Yes, you can perform validation and other actions when an input field gains or loses focus by using the onFocus and onBlur methods in your component. -
What is the difference between focus and blur events?
The focus event occurs when an element gains focus, while the blur event occurs when it loses focus. Both can be used to manage user interactions effectively. -
Is the onFocusEvent compatible with all HTML elements?
The onFocusEvent is primarily used with interactive elements like input fields, buttons, and links. However, it may not be applicable to all HTML elements.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn