How to Use One-Time Data Binding in Angular 2+
- Understanding One-Time Data Binding
- Implementing One-Time Data Binding in Angular
- Advantages of One-Time Data Binding
- Conclusion
- FAQ
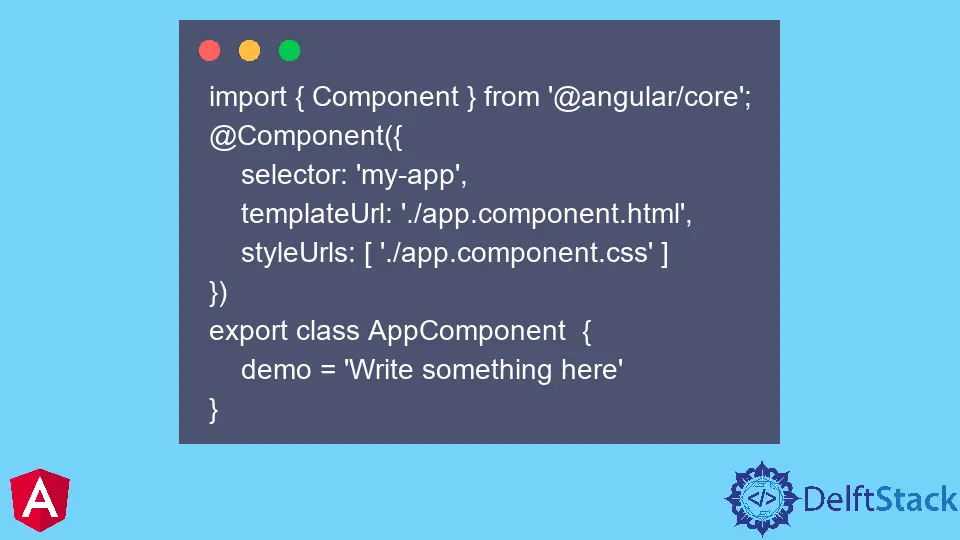
In the world of Angular 2+, understanding data binding is essential for building dynamic applications. One-time data binding is a unique feature that allows developers to optimize their applications by ensuring that the view is updated only when there’s a change in the model. This can lead to improved performance, especially in scenarios where data doesn’t change frequently.
In this article, we’ll explore how to implement one-time data binding in Angular 2+, highlighting its advantages and providing practical examples. Whether you’re a beginner or an experienced Angular developer, mastering this concept can significantly enhance your development skills.
Understanding One-Time Data Binding
One-time data binding in Angular 2+ is a technique that allows you to bind data to the view in such a way that it only updates when the model changes. This is particularly useful for static data that doesn’t require constant updates. Instead of Angular continuously checking for changes, one-time binding tells Angular to set the value once and ignore it thereafter. This can lead to performance gains, especially in large applications.
In Angular, you can implement one-time data binding using the ::
syntax. For instance, if you have a property in your component that you want to display in your template, you can use one-time binding like this: {{ ::propertyName }}
. This will ensure that even if the property changes later, the view will not reflect those changes, thus optimizing rendering.
Implementing One-Time Data Binding in Angular
To implement one-time data binding in Angular 2+, you need to follow a few simple steps. Let’s go through a practical example that showcases how to use this feature effectively.
Step 1: Setting Up Your Angular Component
First, you need to create a component that will utilize one-time data binding. Here’s a simple implementation:
typescriptCopyimport { Component } from '@angular/core';
@Component({
selector: 'app-one-time-binding',
template: `
<h1>One-Time Data Binding Example</h1>
<p>Name: {{ ::name }}</p>
<button (click)="changeName()">Change Name</button>
`
})
export class OneTimeBindingComponent {
name: string = 'John Doe';
changeName() {
this.name = 'Jane Doe';
}
}
In this component, we have a property called name
initialized to “John Doe”. The template uses one-time data binding to display the name. When the button is clicked, the changeName
method updates the name
property.
Output:
textCopyName: John Doe
When you click the button, the name changes in the model, but the view remains unchanged due to one-time binding.
Step 2: Understanding the Template Syntax
The template syntax {{ ::name }}
is crucial for one-time data binding. The ::
operator tells Angular to bind the name
property only once. After the initial binding, any subsequent changes to the name
property will not affect the view.
This approach is particularly beneficial in scenarios where data does not change often. It reduces the overhead of Angular’s change detection mechanism, allowing your application to run more efficiently.
Step 3: Testing the Application
Once you have set up your component, you can test it by running your Angular application. You should see the initial name displayed, and clicking the button to change the name will not update the displayed value.
Output:
textCopyName: John Doe
This demonstrates the effectiveness of one-time data binding in Angular 2+. It allows you to optimize performance by avoiding unnecessary updates to the view.
Advantages of One-Time Data Binding
One-time data binding offers several advantages that can enhance the performance of your Angular applications. Here are some key benefits:
-
Performance Optimization: By reducing the frequency of updates to the view, you decrease the load on Angular’s change detection mechanism, leading to better performance, especially in large applications.
-
Simplicity: One-time data binding simplifies your code by making it clear which properties are intended to be static. This can help other developers understand your intentions better.
-
Reduced Complexity: With one-time binding, you can avoid complex logic that handles frequent updates, making your codebase cleaner and easier to maintain.
-
Memory Efficiency: Since one-time bindings do not require Angular to watch for changes, they can lead to lower memory consumption, which is especially beneficial for mobile applications.
By leveraging one-time data binding, you can create more efficient and maintainable Angular applications.
Conclusion
One-time data binding in Angular 2+ is a powerful technique that can significantly enhance the performance of your applications. By understanding how to implement and utilize this feature, you can create more efficient and responsive user interfaces. Whether you are working with static data or optimizing existing applications, mastering one-time data binding is a valuable skill for any Angular developer. Start incorporating this technique into your projects and experience the benefits of improved performance and cleaner code.
FAQ
-
What is one-time data binding in Angular 2+?
One-time data binding is a technique that updates the view only when there is a change in the model, optimizing performance. -
How do I implement one-time data binding in my Angular application?
You can implement one-time data binding using the{{ ::propertyName }}
syntax in your templates. -
What are the advantages of using one-time data binding?
The advantages include performance optimization, simplicity, reduced complexity, and memory efficiency. -
Can I use one-time data binding with dynamic data?
One-time data binding is best suited for static data. For dynamic data, regular two-way binding is recommended. -
Is one-time data binding available in all versions of Angular?
One-time data binding is available in Angular 2+ and continues to be supported in later versions.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook