Ng-Keypress in AngularJS
- Understanding ng-keypress
- Implementing ng-keypress in AngularJS
- Best Practices for Using ng-keypress
- Conclusion
- FAQ
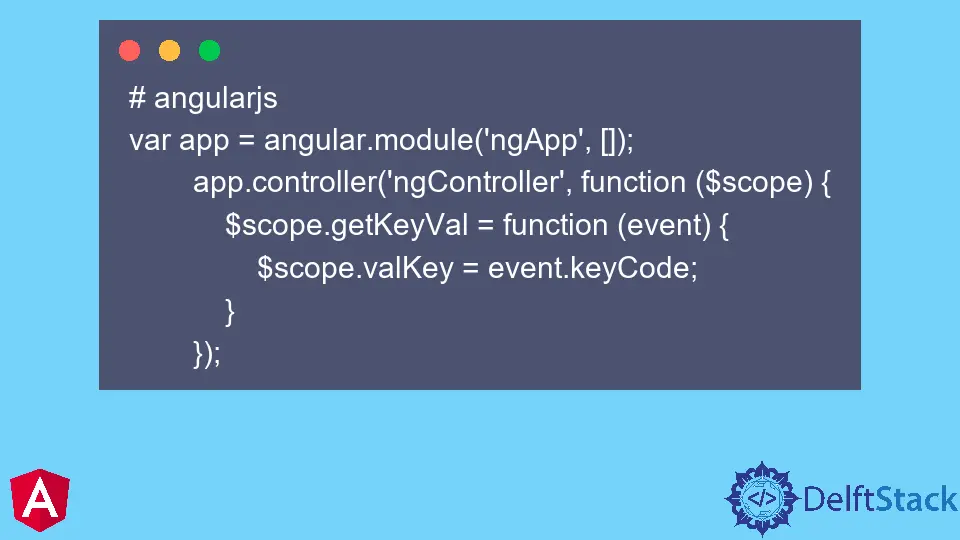
In the world of web development, AngularJS stands out as a powerful framework for building dynamic web applications. One of the lesser-known features that can significantly enhance user experience is the ng-keypress
directive. This directive allows developers to capture keyboard events seamlessly, enabling a variety of functionalities like form validation, real-time search, and enhanced accessibility.
In this article, we will explore how to effectively use ng-keypress
in AngularJS. Whether you’re a seasoned developer or just starting, this guide will provide you with practical insights and code examples to implement ng-keypress
in your applications.
Understanding ng-keypress
The ng-keypress
directive in AngularJS is used to bind an event to the keypress action on an input field. When a user types in the input field, the ng-keypress
directive captures the event and executes a specified function. This functionality is particularly useful for handling user input in real-time, such as validating form fields or triggering search results as the user types.
To use ng-keypress
, you simply need to add the directive to your HTML element, along with the function you want to execute when a key is pressed. The syntax is straightforward and integrates seamlessly with AngularJS’s two-way data binding.
Here’s a basic example of how to implement ng-keypress
in your AngularJS application:
<div ng-app="myApp" ng-controller="myCtrl">
<input type="text" ng-model="userInput" ng-keypress="handleKeypress($event)">
<p>You typed: {{userInput}}</p>
</div>
In this example, we have a simple text input bound to the model userInput
. The ng-keypress
directive calls the handleKeypress
function whenever a key is pressed.
Output:
You typed: (text input by user)
The handleKeypress
function will process the keypress event, allowing you to implement specific logic based on the user’s input.
Implementing ng-keypress in AngularJS
To implement ng-keypress
, you first need to set up an AngularJS application. Here’s how to do it step-by-step:
-
Create an AngularJS Application: Start by including the AngularJS library in your HTML file. You can use a CDN link or download it locally.
-
Define Your Module and Controller: In your JavaScript file, create an AngularJS module and controller. Within the controller, define the function that will handle keypress events.
-
Use ng-keypress in Your HTML: Add the
ng-keypress
directive to your input fields and bind it to the function you defined in your controller.
Here’s a complete example:
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular.min.js"></script>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.userInput = '';
$scope.handleKeypress = function(event) {
if (event.which === 13) {
alert('You pressed Enter: ' + $scope.userInput);
}
};
});
</script>
</head>
<body ng-controller="myCtrl">
<input type="text" ng-model="userInput" ng-keypress="handleKeypress($event)">
<p>You typed: {{userInput}}</p>
</body>
</html>
Output:
You typed: (text input by user)
In this example, the handleKeypress
function checks if the Enter key (key code 13) is pressed. If so, it displays an alert with the current user input. This demonstrates how you can capture specific key events and implement custom logic.
Best Practices for Using ng-keypress
When using ng-keypress
, there are several best practices to keep in mind to ensure your application remains efficient and user-friendly.
-
Debouncing Input: If you are using
ng-keypress
for real-time search or similar functionalities, consider implementing a debounce mechanism. This prevents the function from being called too frequently, which can lead to performance issues. -
Accessibility: Always ensure that your application is accessible. Provide alternative methods for users who may rely on assistive technologies.
-
Avoiding Default Actions: If you want to prevent the default action of a keypress (like submitting a form), you can call
event.preventDefault()
within your handler function. -
Testing: Always test your
ng-keypress
functionality across different browsers and devices. Ensure that your application behaves consistently and that all key events are captured as expected.
By following these best practices, you can enhance the user experience and maintain the performance of your AngularJS applications.
Conclusion
The ng-keypress
directive in AngularJS is a powerful tool that allows developers to create dynamic and interactive user experiences. By capturing keyboard events, you can implement features such as real-time validation, search functionality, and more. In this article, we explored how to use ng-keypress
, provided code examples, and discussed best practices to ensure your application remains efficient and user-friendly. Whether you’re building a simple form or a complex web application, mastering ng-keypress
can significantly enhance your development toolkit.
FAQ
-
What is ng-keypress in AngularJS?
ng-keypress is a directive in AngularJS that allows developers to capture keyboard events when a user types in an input field. -
How do I use ng-keypress?
You can use ng-keypress by adding it to an input element and binding it to a function in your AngularJS controller that processes the keypress event. -
Can I prevent default actions with ng-keypress?
Yes, you can prevent default actions by calling event.preventDefault() within your keypress handler function. -
Is ng-keypress suitable for real-time search functionalities?
Absolutely! ng-keypress is ideal for implementing real-time search as it captures user input immediately. -
What are some best practices for using ng-keypress?
Best practices include debouncing input, ensuring accessibility, avoiding default actions, and thorough testing across different devices.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn