How to Get Current Index in ngFor
- Understanding ngFor
- Accessing the Current Index in ngFor
- Using ngFor with Additional Features
- Conclusion
- FAQ
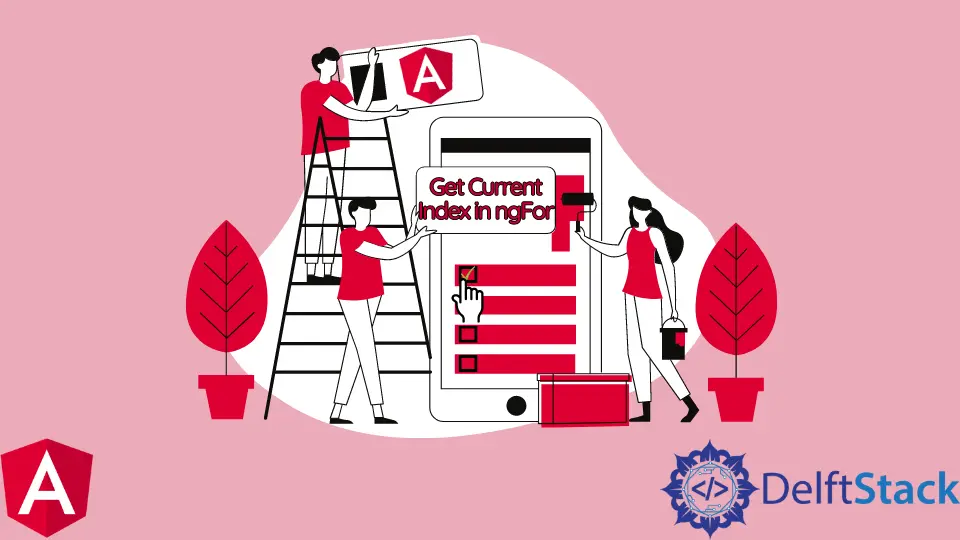
In the world of Angular, displaying lists of items is a common task, especially when working with dynamic data. One of the most useful features of Angular is the ngFor
directive, which allows you to iterate over a collection and render elements based on that collection. However, a challenge often arises when you need to access the current index of the item being rendered.
In this tutorial, we’ll explore how to effectively get the current index in ngFor
, particularly when displaying items in a tabular format. By the end of this guide, you’ll have a clear understanding of how to incorporate indices into your Angular templates seamlessly.
Understanding ngFor
Before diving into the specifics of getting the current index, let’s briefly discuss what ngFor
is and how it functions. The ngFor
directive is a structural directive in Angular that allows you to repeat a portion of the template for each item in a collection. This is particularly useful for rendering lists or tables where each item can be represented as a row or cell.
To use ngFor
, you typically define it within an HTML element, specifying the collection you want to iterate over. For instance, if you have an array of objects, you can easily render them in a table format.
<table>
<tr *ngFor="let item of items">
<td>{{ item.name }}</td>
</tr>
</table>
In this example, each item
in the items
array will generate a new row in the table. But how do we get the index of each item as we iterate? Let’s explore that next.
Accessing the Current Index in ngFor
To access the current index of the item being rendered in an ngFor
loop, Angular provides a simple syntax. You can use the let i = index
syntax within the ngFor
directive. This allows you to capture the index of the current iteration, which can then be used within your template.
Here’s how you can implement this in a table:
<table>
<tr *ngFor="let item of items; let i = index">
<td>{{ i + 1 }}</td>
<td>{{ item.name }}</td>
</tr>
</table>
In this code snippet, let i = index
captures the current index of the item in the items
array. The index is then displayed in the first cell of each row. We add 1 to the index to display it in a human-friendly format, starting from 1 instead of 0.
The output would look something like this:
Output:
1 | Item 1
2 | Item 2
3 | Item 3
Using the index in this way can be particularly helpful for creating more interactive and user-friendly interfaces. For instance, you can use the index to highlight selected items or to manage pagination.
Using ngFor with Additional Features
Angular’s ngFor
directive also allows you to combine it with other directives and features for enhanced functionality. For example, you can use the index to conditionally style elements or manage complex data structures.
Let’s say you want to highlight every even-indexed row in a table. You can achieve this by using the index in combination with Angular’s built-in ngClass
directive.
<table>
<tr *ngFor="let item of items; let i = index" [ngClass]="{'highlight': i % 2 === 0}">
<td>{{ i + 1 }}</td>
<td>{{ item.name }}</td>
</tr>
</table>
In this case, we use [ngClass]
to apply a CSS class called highlight
to every even-indexed row. The expression i % 2 === 0
evaluates to true for even indices, thus applying the class conditionally.
The output will show alternating row colors based on the applied styles, making the table easier to read and visually appealing.
Output:
1 | Item 1
2 | Item 2
3 | Item 3
By utilizing the current index effectively, you can create more dynamic and engaging user interfaces that respond to user interactions and data changes.
Conclusion
In this tutorial, we’ve explored how to get the current index in Angular’s ngFor
directive. We learned how to use the let i = index
syntax to access the index of items being rendered, enabling us to enhance our templates with additional features like conditional styling. By mastering these techniques, you can create more interactive and user-friendly applications. Whether you’re building simple lists or complex data tables, understanding how to work with indices in ngFor
will undoubtedly enhance your Angular development skills.
FAQ
-
How do I use ngFor to display a list of objects?
You can use the ngFor directive in Angular to iterate over an array of objects and display them in a template, such as a table or a list. -
Can I access the index of an item in ngFor?
Yes, you can access the index by using the syntaxlet i = index
within the ngFor directive. -
How can I style specific rows in a table using ngFor?
You can use Angular’s ngClass directive in combination with the index to conditionally apply styles to specific rows based on their index. -
Is it possible to start the index from 1 instead of 0 in ngFor?
Yes, you can simply add 1 to the index value when displaying it in the template to start counting from 1. -
What are some common use cases for using the index in ngFor?
Common use cases include creating numbered lists, highlighting selected items, and implementing pagination features.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn