How to Use The for Loop in Angular
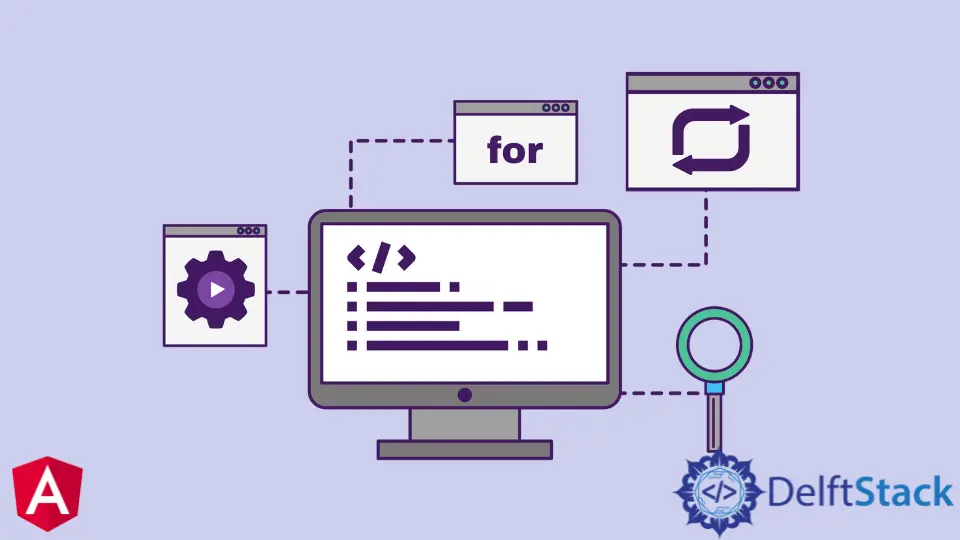
We will introduce the for
loop and display data using the for
loop in AngularJs.
the for
Loop in Angular
The for
loop is convenient, and it is used to repeat a code a number of times either to display data.
To understand it, let’s go through an example of for
loop in AngularJs. First of all, we will create a template.
# angularjs
<div ng-app='ForApp' ng-repeat="n in [] | repeat:5">
<h1>{{n}}</h1>
</div>
In the code above, we used ng-repeat
. Now, let’s write a for
loop to display the value of n
.
# angularjs
var myApp = angular.module('ForApp', []);
myApp.filter('repeat', function() {
return function(input, range) {
range = parseInt(range);
for (var i=0; i<range; i++)
input.push(i);
return input;
};
});
The code above will output n
value five times.
As you can see in the image, the above code shows the value of n
five times. We can also use the for
loop to repeat a particular HTML code a number of times.
# angularjs
<div ng-app='ForApp' ng-repeat="n in [] | repeat:5">
<h1>Repeated for {{n}} times</h1>
</div>
Output:
In this way, we can use the for
loop in AngularJs.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn