How to Filter Options in a Select List in Angular
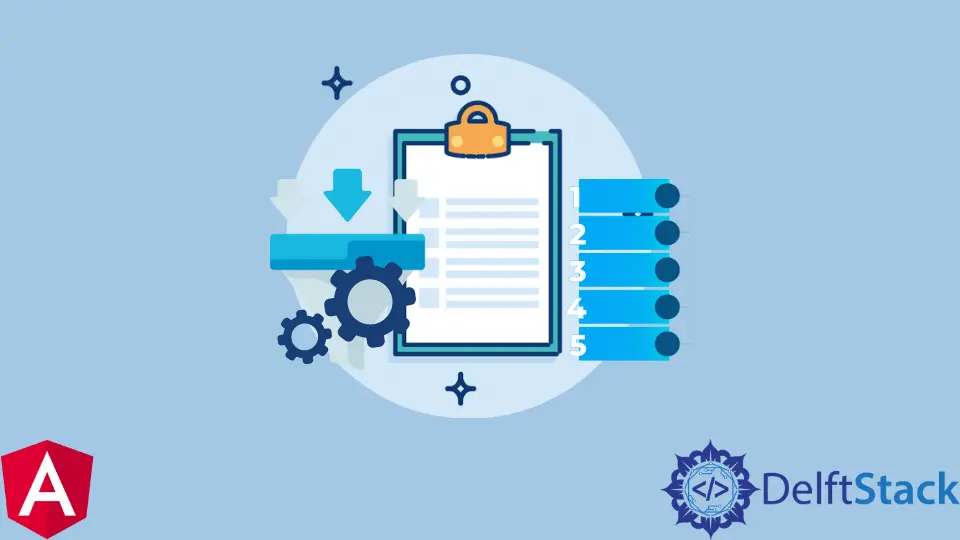
ng-options
is a directive that makes it easier to create an HTML dropdown box for selecting an item from an array that will be saved in a model. It dynamically constructs a list of option elements for the select element by analyzing the ng-options
evaluation expression and returning an array or object.
Moreover, the ng-options
directive creates a select element in Angular. It can filter a list of items by the user’s input.
Steps to Filter Options in a Select List in Angular
The steps are as follows:
-
First, create an Angular project by running the command below in your terminal.
ng new angular_filter_select
-
Next, create a component for the
select
that will be filtered byng-options
.ng g c SelectFilter
-
Finally, add a
select
to your component and pass it an expression that will be used for filtering. The expression is passed using an attribute calledng-model
which should match the variable name being filtered.
Using the above steps, let’s see an example of the ng-option
filter.
TypeScript code:
var app = angular.module('plunker', []);
app.controller('MainCtrl', function($scope) {
$scope.CountryName =[{"countryName":"England","isDisabled":false},
{"countryName":"Netherlands","isDisabled":false},
{"countryName":"USA","isDisabled":false},
{"countryName":"China","isDisabled":false},
{"countryName":"India","isDisabled":false}];
});
HTML code:
<html ng-app="plunker">
<head>
<script>document.write('<base href="' + document.location + '" />');</script>
<script data-require="angular.js@1.5.x" src="https://code.angularjs.org/1.5.8/angular.js" data-semver="1.5.8"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<H2>
Example of Using Filter with ng-options
</H2>
<select
ng-options="country.countryName as country.countryName disable when country.isDisabled for country in CountryName |filter:{isDisabled:false}"
ng-model="myCountry">
<option value="">Select a Country</option>
</select>
</body>
</html>
Click here to check the live demonstration of the code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook