How to Download File in Angular
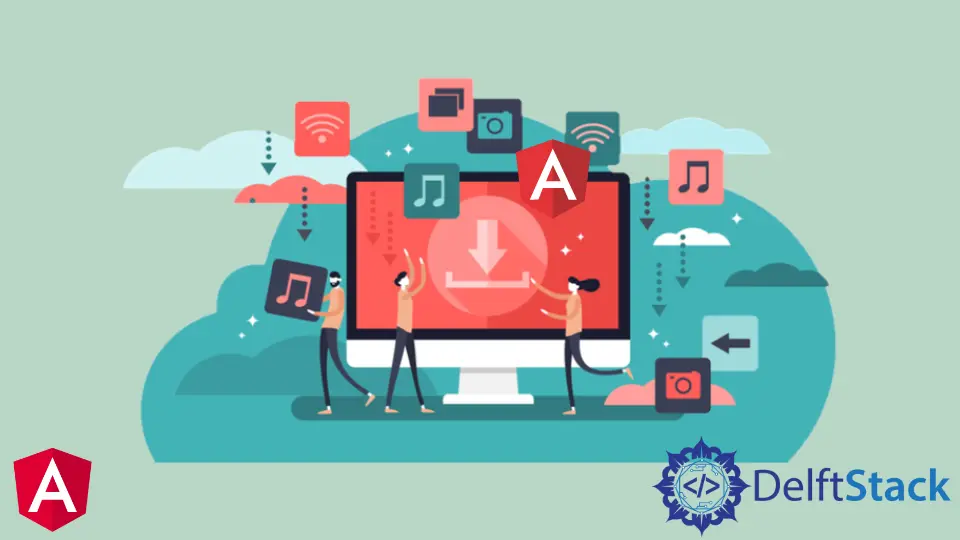
We will introduce how to download a file in Angular on a button click and show an example.
File Download in Angular
It is straightforward to download a file in Angular. We can download a file using the HTML5 download
attribute.
# angular
<a href="FilePath.csv"
class="btn"
target="_blank"
download="FileName.csv"
>Download Now</a>
Example
First, we will create a assets
folder in the src
folder, and we will store the file we want to download in the assets
folder.
We will add the following code in app.component.html
.
# angular
<hello name="{{ name }}"></hello>
<a href="assets/download.csv"
class="btn btn-default"
target="_blank"
download="download.csv"
>Download Now</a>
Output:
We will style our button by adding the following code in the app.component.css
.
# angular
p {
font-family: Lato;
}
.btn{
padding: 10px;
background-color: blueviolet;
color: white;
border-radius: 5px;
text-decoration: none;
}
Output:
When we click on the Download Now
button, it will download the file stored in the assets
folder.
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn