How to Create a Navbar in Angular
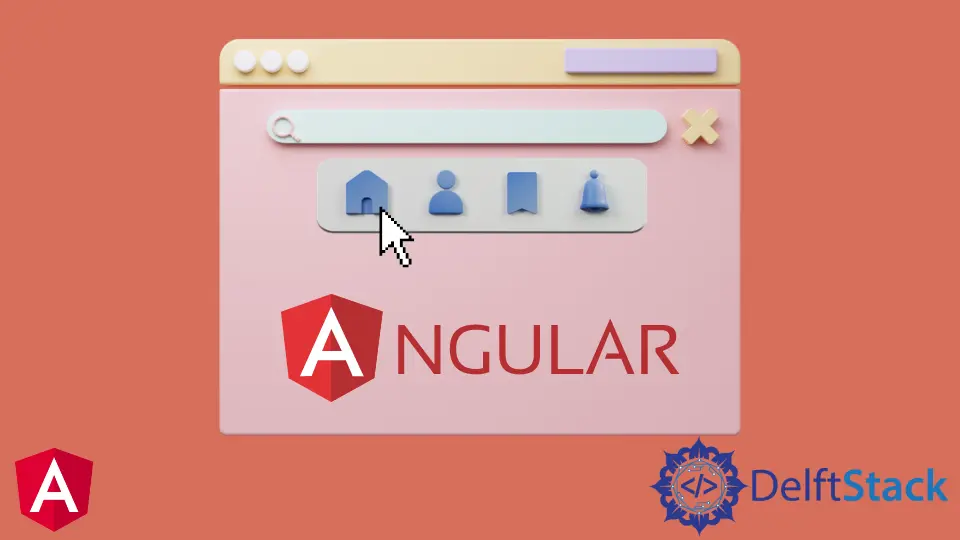
This tutorial will introduce how to create a navbar in Angular and use it for navigation in Angular applications.
The Navbar in Angular
Navbar is one of the essential parts of any web application. Even when building a single-page application (SPA) consisting of only one page but different sections, we still use the navbar to navigate from one section to another.
Navbar allows users to easily find what they are looking for on your web applications.
There are many methods for navigation to achieve simple to complex routing. Angular provides a separate module that helps us create a navbar in our web application and use it for navigation.
We can use the router navigate()
method if we want to navigate the user from one page to another programmatically, which means we navigate components without using the exact links. We use component names instead.
Create a Navbar in Angular
Let’s go through an example in which we will create a navbar and use it to navigate through different components using navigate()
. So, let’s make a new application using the following command.
# angular
ng new my-app
After creating our new application, we will go to our application directory using this command.
# angular
cd my-app
Now, let’s run our app to ensure all dependencies are installed correctly.
# angular
ng serve --open
Generate Navbar Components in Angular
Now, let’s generate the navbar components. First, we will generate our index
component, which will act as our application’s home
component.
# angular
ng generate component index
Then, we will generate the about us
component.
# angular
ng generate component aboutUs
Lastly, we will generate our products
component using the following command.
# angular
ng generate component products
We will have 3 components in separate folders with 3 files, which you can see below.
Output:
Create the Views for the Components
Now, we will create views for our components. First, we will open aboutUs.component.html
from the about
folder and add the following code.
# angular
<div class="container" >
<h1> This is about Us Component </h1>
<h3> This is the content of About Us </h3>
</div>
Next, we will open the index.component.html
file from the home
folder and add the following code.
# angular
<div class="container">
<h1> This is index component </h1>
<h3> This is the content of Index </h3>
</div>
We will open the products.component.html
file from the services
folder and add the following code.
# angular
<div class="container">
<h1> This is products component </h1>
<h3> This is the content of Products </h3>
</div>
Define the Component Routes
Once we have created our components and views, we will define our routes in app-routing.module.ts
.
We will import ngModule
. We will also import Routes
and RouterModule
from the router, and last but not least, the components we just created.
# angular
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { AboutUsComponent} from './aboutUs/aboutUs.component';
import { IndexComponent} from './index/index.component';
import { ProductsComponent } from './products/products.component';
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
After importing, we will define our components’ routes, as shown below.
# angular
const routes: Routes = [
{ path: 'aboutUs', component: AboutUsComponent },
{ path: 'index', component: IndexComponent},
{ path: 'products', component: ProductsComponent },
];
Create a Navigation Menu
We will then make our navigation menu in app.component.html
. Each link will call a function with the (click)
method.
We will display the components data using router-outlet
as shown below.
# angular
<ul class="nav navbar-nav">
<li>
<a (click)="goToIndex()">Home</a>
</li>
<li>
<a (click)="goToAboutUs()">About Us</a>
</li>
<li>
<a (click)="goToProducts()">Products</a>
</li>
</ul>
<router-outlet> </router-outlet>
Create the Functions to Navigate Between Components
Next, we will create our functions goToIndex()
, goToAboutUs()
, and goToProducts()
. We’ll open app.component.ts
and import Router
.
Using router.navigate()
, we will create these functions to navigate between components.
# angular
import { Component } from '@angular/core';
import { Router } from '@angular/router';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
constructor(private router: Router) {
}
goToIndex() {
this.router.navigate(['/', 'index']);
}
goToAboutUs() {
this.router.navigate(['/', 'aboutUs']);
}
goToProducts() {
this.router.navigate(['/', 'products']);
}
}
Output:
As you can see from the example, we can easily create a navbar in our angular applications and use it to navigate between components using navigate()
and defining routes.
You can access the complete code used in this tutorial.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn