Concept of ngIf in Angular 2
-
Hide Components in CSS vs.
ngIf
in Angular 2 -
Expressions in
ngIf
in Angular 2 -
Steps to Use
ngIf
in Angular 2
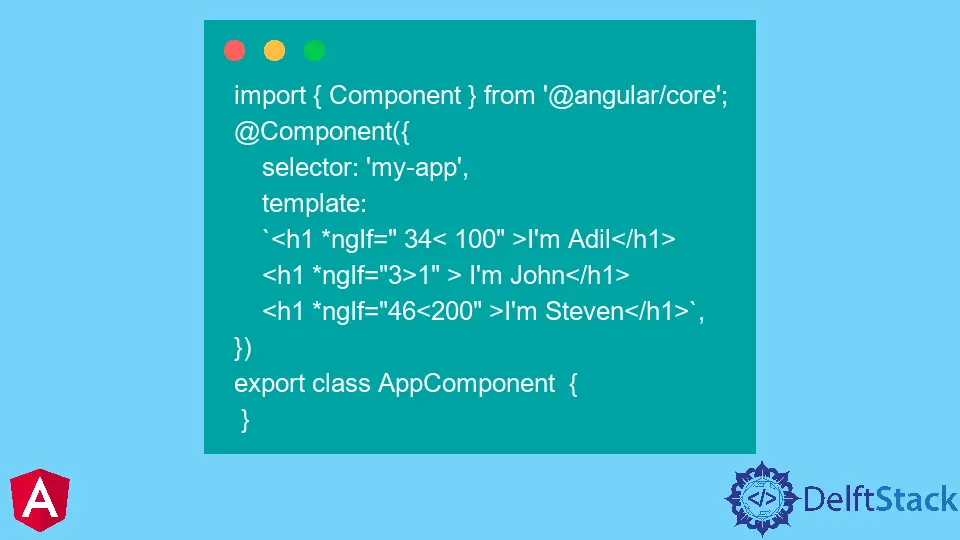
In Angular 2, the ngIf
directive is one of the most straightforward and useful directives in Angular 2. It is used to conditionally display or hide HTML elements based on the evaluation of an expression.
The ngIf
directive evaluates an expression, and if it evaluates true, it will render a given element. If not, it will not render that element at all.
This directive helps reduce the number of DOM elements when multiple views share common characteristics.
This article will teach you everything about ngIf
.
Hide Components in CSS vs. ngIf
in Angular 2
Even though HTML lacks an if
statement, the show and visibility attributes in CSS can be used to hide sections of the page.
We can add or remove CSS properties from an HTML element and hide an element from the page using JavaScript. However, this is not the same as ngIf
.
If an element is hidden in ngIf
, it does not exist on the page.
If you use Chrome Dev Tools to investigate the page, you will not see any HTML elements in the DOM.
Expressions in ngIf
in Angular 2
The ngIf
directive accepts any valid Typescript phrase as an input, not only a boolean. The expression’s thoroughness will next be assessed to determine whether the element should be displayed or not.
We may also send texts, arrays, and objects to ngIf
in addition to booleans.
Steps to Use ngIf
in Angular 2
The following steps are to be followed to use ngIf
in Angular 2:
-
Write an expression that will be evaluated as true or false.
-
Wrap the expression with opening and closing parentheses, for example,
(true || false)
. -
Add a pipe character
|
between the opening and closing parentheses. -
Add the
ngIf
directive to your template with the expression from step 1.
Example:
Let’s discuss a complete example of ng-if
in angular 2.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template:
`<h1 *ngIf=" 34< 100" >I'm Adil</h1>
<h1 *ngIf="3>1" > I'm John</h1>
<h1 *ngIf="46<200" >I'm Steven</h1>`,
})
export class AppComponent {
}
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook